Exercise 2 (BankAccount) Write a Java program that simulates primitive bank operations. To achieve this goal, follow these steps: 1. Declare a static field amount with default value 5000.00. 2. Declare another static field of type java.util.Scanner. 3. Define a method displayMenu() that prints the available operations (Amount, Deposit, Withdraw, and Exit). 4. Define a method getChoice() that calls displayMenu(), prompt the user to enter his/her choice, reads and returns the choice (1, 2, 3, or 4). 5. Define a method displayAmount() that display the current amount value. 6. Define a method depositAmount() that takes an amount as parameter, adds it to the current amount, and displays the new value of the amount. 7. Define a method withdrawAmount() that takes an amount as parameter, removes it from the current amount, and displays the new value of the amount. 8. Write a main() method that calls getChoice() repeatedly until the user enters the choice 4 (exit), performs (calls) the right operation (method) according to the user choice. The main() content could be: int operation; while ( (operation = getChoice()) != 4 ) { switch (operation) { case 1 : displayAmount(); break; case 2: System.out.print("Enter the amount to deposit: "); depositAmount(input.nextDouble()); break; case 3: System.out.print("Enter the amount to withdraw: "); withdrawAmount(input.nextDouble()); break; default: System.out.println("Unknown operation."); } }
Exercise 2 (BankAccount)
Write a Java program that simulates primitive bank operations. To achieve this goal, follow
these steps:
1. Declare a static field amount with default value 5000.00.
2. Declare another static field of type java.util.Scanner.
3. Define a method displayMenu() that prints the available operations (Amount,
Deposit, Withdraw, and Exit).
4. Define a method getChoice() that calls displayMenu(), prompt the user to enter
his/her choice, reads and returns the choice (1, 2, 3, or 4).
5. Define a method displayAmount() that display the current amount value.
6. Define a method depositAmount() that takes an amount as parameter, adds it to the
current amount, and displays the new value of the amount.
7. Define a method withdrawAmount() that takes an amount as parameter, removes it
from the current amount, and displays the new value of the amount.
8. Write a main() method that calls getChoice() repeatedly until the user enters the
choice 4 (exit), performs (calls) the right operation (method) according to the user
choice.
The main() content could be:
int operation;
while ( (operation = getChoice()) != 4 )
{
switch (operation)
{
case 1 :
displayAmount();
break;
case 2:
System.out.print("Enter the amount to deposit: ");
depositAmount(input.nextDouble());
break;
case 3:
System.out.print("Enter the amount to withdraw: ");
withdrawAmount(input.nextDouble());
break;
default:
System.out.println("Unknown operation.");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

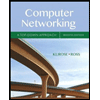
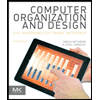
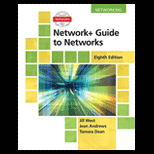
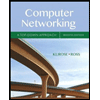
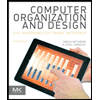
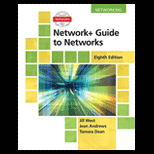
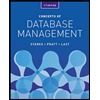
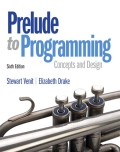
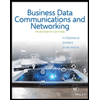