erwise, it should return the Student associated with the id. If the student that is removed was registered, then this student should be replaced by the student who is first in the waitlist queue. If the student who is removed was on
Implement solution for remove(int id)
removes the Student (classt type) associated with this id; if the id is not found in the table or on the waitlist, then it should return null; otherwise, it should return the Student associated with the id. If the student that is removed was registered, then this student should be replaced by the student who is first in the waitlist queue. If the student who is removed was on the waitlist, then they should just be removed from the waitlist. You should go directly to slot id % m rather than iterating through all the slots.
public class Course {
public String code;
public int capacity;
public SLinkedList<Student>[] studentTable;
public int size;
public SLinkedList<Student> waitlist;
public Course(String code) {
this.code = code;
this.studentTable = new SLinkedList[10];
this.size = 0;
this.waitlist = new SLinkedList<Student>();
this.capacity = 10;
}
public Course(String code, int capacity) {
this.code = code;
this.studentTable = new SLinkedList[capacity];
this.size = 0;
this.waitlist = new SLinkedList<>();
this.capacity = capacity;
}
public Student remove(int id) {
// insert your solution here and modify the return statement
return null;
}
![public String tostring() {
30
4
String s = "Course: "+ this.code +"\n";
S +=
-\n";
for (int i = 0; i < this.studentTable.length; i++) {
|\n";
s += "|"+i+"
s += "|
SLinkedList<Student> list = this.studentTable[i];
if (list != null) {
for (int j = 0; j < list.size(); j++) {
------> ":
Student student = list.get(j);
S +=
student.id + ": "+ student.name +" --> ";
4
}
}
S += "\n-
--\n\n";
}
return s;
}
}
1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9c002e4e-ece1-4dd1-9934-cbb442392244%2F4747c57b-47d3-4102-b8d8-948cf09629b6%2Fajyct9n_processed.png&w=3840&q=75)


Step by step
Solved in 2 steps

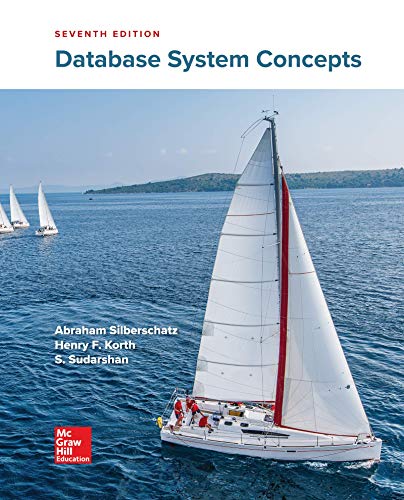
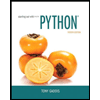
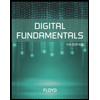
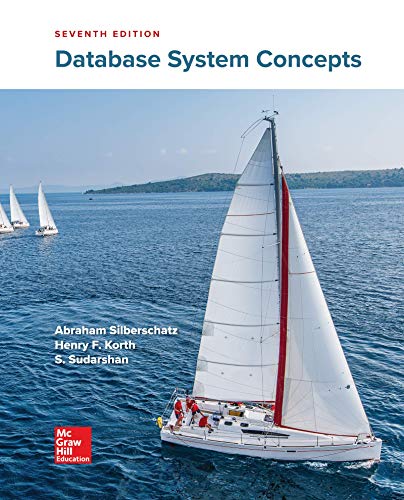
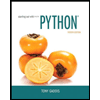
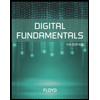
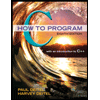
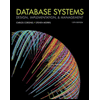
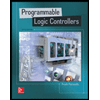