Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness. Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list: - Create (insert) 4 stations - List the stations - Check if a station is in the list (print result). Check for a station that exists, and one that doesn’t - Remove a station. List the stations - Add a station before another station. List the stations - Add a station after another station. Print the stations LinkedList.java package linkedList; public interface LinkedList { public Boolean isItemInList(String thisItem); // true if it is, false if not public Boolean addItem(String thisItem); // true if added, false if it was already there, or an error public Integer itemCount(); public void listItems(); public Boolean deleteItem(String thisItem); // true if deleted, false if not there or error public Boolean insertBefore(String newItem, String itemToInsertBefore); public Boolean insertAfter(String newItem, String itemToInsertAfter); //ExtraCredit public void sort(); // ascending alphanumeric sort; nothing fancy but ALTERS THE LIST, DOES NOT COPY. } // Implement this interface using class ListItem // Also implement the tester in this package. LinkedListImpl.java package linkedList; public class LinkedListImpl implements LinkedList { } LinkedListTester.java package linkedList; public class LinkedListTester { public static void main(String[] args) { // create implementation, then... } } ListItem.java package linkedList; public class ListItem { public String data; public ListItem next; public ListItem(String data) { this.data = data; this.next = null; } }
Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness. Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list:
- Create (insert) 4 stations
- List the stations
- Check if a station is in the list (print result). Check for a station that exists, and one that doesn’t
- Remove a station. List the stations
- Add a station before another station. List the stations
- Add a station after another station. Print the stations
LinkedList.java
package linkedList;
public interface LinkedList {
public Boolean isItemInList(String thisItem);
// true if it is, false if not
public Boolean addItem(String thisItem);
// true if added, false if it was already there, or an error
public Integer itemCount();
public void listItems();
public Boolean deleteItem(String thisItem);
// true if deleted, false if not there or error
public Boolean insertBefore(String newItem, String itemToInsertBefore);
public Boolean insertAfter(String newItem, String itemToInsertAfter);
//ExtraCredit
public void sort();
// ascending alphanumeric sort; nothing fancy but ALTERS THE LIST, DOES NOT COPY.
}
// Implement this interface using class ListItem
// Also implement the tester in this package.
LinkedListImpl.java
package linkedList;
public class LinkedListImpl implements LinkedList {
}
LinkedListTester.java
package linkedList;
public class LinkedListTester {
public static void main(String[] args) {
// create implementation, then...
}
}
ListItem.java
package linkedList;
public class ListItem {
public String data;
public ListItem next;
public ListItem(String data) {
this.data = data;
this.next = null;
}
}

Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness. Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list:
- Create (insert) 4 stations
- List the stations
- Check if a station is in the list (print result). Check for a station that exists, and one that doesn’t
- Remove a station. List the stations
- Add a station before another station. List the stations
- Add a station after another station. Print the stations
LinkedList:
LinkedList is a linear data structure that consists of nodes and links that connect these nodes. Each node in a linked list contains a data element and a reference to the next node in the list. The first node in the list is called the head, and the last node is called the tail. Linked lists can be singly linked, where each node has only a reference to the next node or doubly linked, where each node has a reference to both the next and previous nodes.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

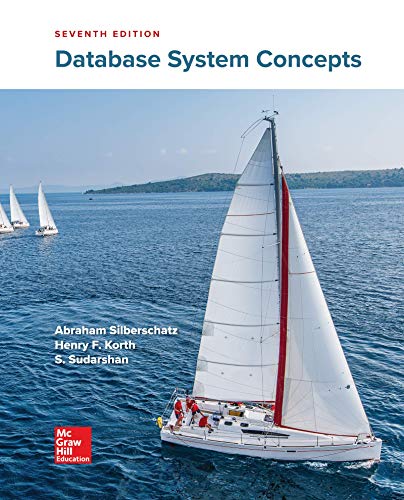
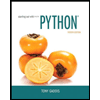
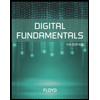
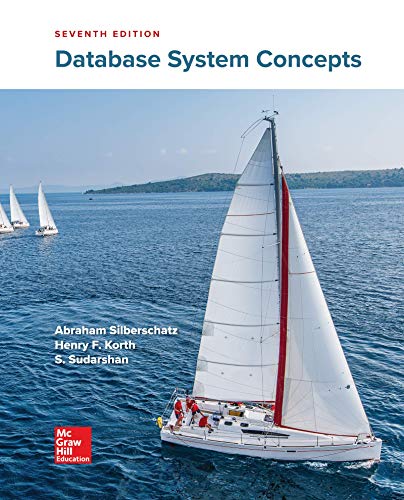
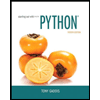
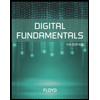
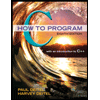
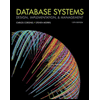
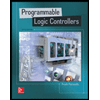