efine a JavaScript comparator function named rossorder with 2 parameters. Both parameters will be objects. Each object will have 3 keys: "points", "goals", and "games". T alue paired with each key will be a Number. Each object represents an NHL player. Since rossOrder is a comparator function, it must return a Number as follows: • a negative value - indicating that the value of the first parameter comes before the value of the second parameter • a positive value - indicating that the value of the first parameter comes after the value of the second parameter • zero-indicating that the values of the two parameters appear at the same location in the order. o determine how the objects should be ordered, rossOrder should use the following rules (based upon the NHL's Ross Trophy): • when the objects have different values for "points", the object with fewer points comes first; • when the objects have the same number of points but unequal values for "goals" key, the object with fewer goals comes first; • when the objects have equal points and goals, the objects are equivalent. ample test cases: et mcdavid = {"points": 64, "goals": 22, "games": 43}; et draisaitl= {"points": 64, "goals": 23, "games": 43); et madeup= {"points": 64, "goals": 22, "games": 45}; ossOrder (mcdavid, madeup) would evaluate to 0 ossOrder (draisaitl, mcdavid) would evaluate to any Number greater than 0 ossOrder (madeup, draisaitl) would evaluate to any Number less than 0 2. efine a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
efine a JavaScript comparator function named rossorder with 2 parameters. Both parameters will be objects. Each object will have 3 keys: "points", "goals", and "games". T alue paired with each key will be a Number. Each object represents an NHL player. Since rossOrder is a comparator function, it must return a Number as follows: • a negative value - indicating that the value of the first parameter comes before the value of the second parameter • a positive value - indicating that the value of the first parameter comes after the value of the second parameter • zero-indicating that the values of the two parameters appear at the same location in the order. o determine how the objects should be ordered, rossOrder should use the following rules (based upon the NHL's Ross Trophy): • when the objects have different values for "points", the object with fewer points comes first; • when the objects have the same number of points but unequal values for "goals" key, the object with fewer goals comes first; • when the objects have equal points and goals, the objects are equivalent. ample test cases: et mcdavid = {"points": 64, "goals": 22, "games": 43}; et draisaitl= {"points": 64, "goals": 23, "games": 43); et madeup= {"points": 64, "goals": 22, "games": 45}; ossOrder (mcdavid, madeup) would evaluate to 0 ossOrder (draisaitl, mcdavid) would evaluate to any Number greater than 0 ossOrder (madeup, draisaitl) would evaluate to any Number less than 0 2. efine a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
need help writing these two functions. they ar in two seperate images if it helps you view them.
![### Task Overview:
**Objective:**
Define a JavaScript custom sorting function named `rossTrophy` with one parameter. This parameter will be an array of objects. The objects will be as described in a previous section (D1). Your function should sort the array’s objects from the object ranked lowest for the NHL's Ross Trophy to the object ranked highest. This is done by ordering from the smallest to largest points. If objects have equal points, they are sorted by the smallest to largest number of goals.
Since JavaScript's built-in sort function rearranges an array's entries "in place," your `rossTrophy` function must return `undefined`.
### Sample Test Cases:
```javascript
let nhl = [
{ "points": 64, "goals": 22, "games": 43 },
{ "points": 64, "goals": 23, "games": 43 }
];
rossTrophy(nhl);
// Expected Result:
// The order of `nhl` entries remains the same.
// Note: This finds a bug if sorting is not done using the built-in in-place function or
// if sorting is not done by `rossOrder`.
```
### Explanation:
- **Input:**
The function takes an array `nhl`, which consists of objects each having properties: `points`, `goals`, and `games`.
- **Sorting Criteria:**
- First, sort by `points` in ascending order.
- If `points` are the same, sort by `goals` in ascending order.
- **Expected Function Behavior:**
- Rearrange the array `nhl` directly using JavaScript’s built-in sorting method since it modifies the array in place.
- Return `undefined` as the function outcome.
The provided code snippet illustrates a scenario where two elements are compared and are expected to stay in the same order even without swapping, indicating a properly applied in-place sort logic.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F89b7b4a3-edc3-4856-b3b8-970ee403a9a8%2F69b2f09c-e511-498a-85cd-caf9dd1a4d9d%2Fr5j5m3_processed.png&w=3840&q=75)
Transcribed Image Text:### Task Overview:
**Objective:**
Define a JavaScript custom sorting function named `rossTrophy` with one parameter. This parameter will be an array of objects. The objects will be as described in a previous section (D1). Your function should sort the array’s objects from the object ranked lowest for the NHL's Ross Trophy to the object ranked highest. This is done by ordering from the smallest to largest points. If objects have equal points, they are sorted by the smallest to largest number of goals.
Since JavaScript's built-in sort function rearranges an array's entries "in place," your `rossTrophy` function must return `undefined`.
### Sample Test Cases:
```javascript
let nhl = [
{ "points": 64, "goals": 22, "games": 43 },
{ "points": 64, "goals": 23, "games": 43 }
];
rossTrophy(nhl);
// Expected Result:
// The order of `nhl` entries remains the same.
// Note: This finds a bug if sorting is not done using the built-in in-place function or
// if sorting is not done by `rossOrder`.
```
### Explanation:
- **Input:**
The function takes an array `nhl`, which consists of objects each having properties: `points`, `goals`, and `games`.
- **Sorting Criteria:**
- First, sort by `points` in ascending order.
- If `points` are the same, sort by `goals` in ascending order.
- **Expected Function Behavior:**
- Rearrange the array `nhl` directly using JavaScript’s built-in sorting method since it modifies the array in place.
- Return `undefined` as the function outcome.
The provided code snippet illustrates a scenario where two elements are compared and are expected to stay in the same order even without swapping, indicating a properly applied in-place sort logic.
:
- If the "points" are different, the object with fewer points comes first.
- If the "points" are the same but "goals" are different, the object with fewer goals comes first.
- If both "points" and "goals" are equal, the objects are equivalent.
**Sample test cases:**
```javascript
let mcdavid = {"points": 64, "goals": 22, "games": 43};
let draisaitl = {"points": 64, "goals": 23, "games": 43};
let madeup = {"points": 64, "goals": 22, "games": 45};
rossOrder(mcdavid, madeup) would evaluate to 0
rossOrder(draisaitl, mcdavid) would evaluate to any Number greater than 0
rossOrder(madeup, draisaitl) would evaluate to any Number less than 0
```
### D2: JavaScript Custom Sorting Function
Define a **JavaScript** custom sorting function named `rossTrophy` with 1 parameter. This parameter will be an array of objects, as described in D1.
The function should sort the array in ascending order from the object ranked lowest for the NHL's Ross Trophy to the highest, utilizing the `rossOrder` function defined in D1.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F89b7b4a3-edc3-4856-b3b8-970ee403a9a8%2F69b2f09c-e511-498a-85cd-caf9dd1a4d9d%2Fkv0x04b_processed.png&w=3840&q=75)
Transcribed Image Text:### D1: JavaScript Comparator Function
Define a **JavaScript** comparator function named `rossOrder` with 2 parameters. Both parameters will be objects, and each will have 3 keys: `"points"`, `"goals"`, and `"games"`. Each key will correspond to a value that is a **Number**, representing an NHL player.
The `rossOrder` function must return a **Number** as follows:
- **Negative value**: Indicates the first parameter should come before the second.
- **Positive value**: Indicates the second parameter should come before the first.
- **Zero**: Indicates that both parameters are equivalent in their order.
To determine the order, `rossOrder` should use these rules based on the [NHL's Ross Trophy](https://en.wikipedia.org/wiki/Art_Ross_Trophy):
- If the "points" are different, the object with fewer points comes first.
- If the "points" are the same but "goals" are different, the object with fewer goals comes first.
- If both "points" and "goals" are equal, the objects are equivalent.
**Sample test cases:**
```javascript
let mcdavid = {"points": 64, "goals": 22, "games": 43};
let draisaitl = {"points": 64, "goals": 23, "games": 43};
let madeup = {"points": 64, "goals": 22, "games": 45};
rossOrder(mcdavid, madeup) would evaluate to 0
rossOrder(draisaitl, mcdavid) would evaluate to any Number greater than 0
rossOrder(madeup, draisaitl) would evaluate to any Number less than 0
```
### D2: JavaScript Custom Sorting Function
Define a **JavaScript** custom sorting function named `rossTrophy` with 1 parameter. This parameter will be an array of objects, as described in D1.
The function should sort the array in ascending order from the object ranked lowest for the NHL's Ross Trophy to the highest, utilizing the `rossOrder` function defined in D1.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
This cant be right.
first, first function needs to be called "rossOrder"
second, the second function requires only 1 parameter. this has 2.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
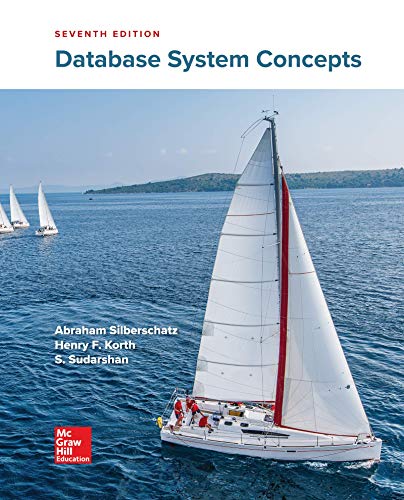
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
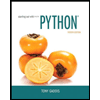
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
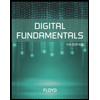
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
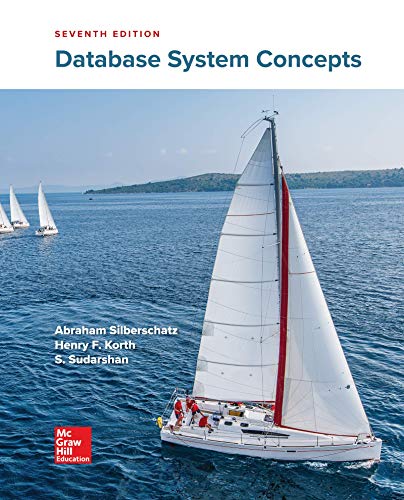
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
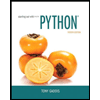
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
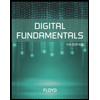
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
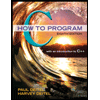
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
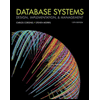
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
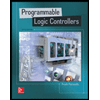
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education