Edit the isPrime function so that it returns False when any number less than 3 is passed (including negative numbers). Then write a function called nPrimes that accepts an integer parameter called n and then prints the first n prime numbers. Start looking for prime numbers at 3, and keep looking until you find n of them. Your nPrimes function should use the isPrime function to determine if a number is prime. Both of these functions should be placed in a file called NPrimes.py. Here is a sample run of the completed program: Enter the number of primes you want to find: 10 3 5 7 11 13 17 19 23 29 31
Edit the isPrime function so that it returns False when any number less than 3 is passed (including negative numbers). Then write a function called nPrimes that accepts an integer parameter called n and then prints the first n prime numbers. Start looking for prime numbers at 3, and keep looking until you find n of them. Your nPrimes function should use the isPrime function to determine if a number is prime. Both of these functions should be placed in a file called NPrimes.py.
Here is a sample run of the completed program:
Enter the number of primes you want to find: 10
3
5
7
11
13
17
19
23
29
31
from NPrimes import nPrimes
def main():
n = int(input("Enter the number of primes you want to find: \n"))
nPrimes(n)
main()
def isPrime(num):
prime = True
for x in range(2, num):
if num % x == 0:
prime = False
break
return prime;

Ans:-
NPrimes.py:-
from math import sqrt
#isPrime function to check whether a number is prime or not
def isPrime(n):
#if number is less than 3 then return false
if n < 3:
return False
#run a loop from 2 to sqrt(n) + 1
for i in range(2, int(sqrt(n)) + 1):
#if a number has any factor then return False
if n % i == 0:
return False
#return True at last
#if program reaches this line then it signifies that the number is not prime
return True
#nPrimes function to print first n prime numbers
def nPrimes(n):
#initialize count to 0
count = 0
#initialize i with 3 to start looking for prime numbers from 3
i = 3
#run a while loop
while True:
#Call isPrime function to check whether a number is prime or not
if(isPrime(i)):
#if function returns true then print the number
print(i)
#increase the count by 1 after printing each prime number
count = count+1
#if n prime number has been print then break from loop
if count == n:
break
#increament the value of i by 1 after every iteration
i = i + 1
Step by step
Solved in 2 steps with 2 images

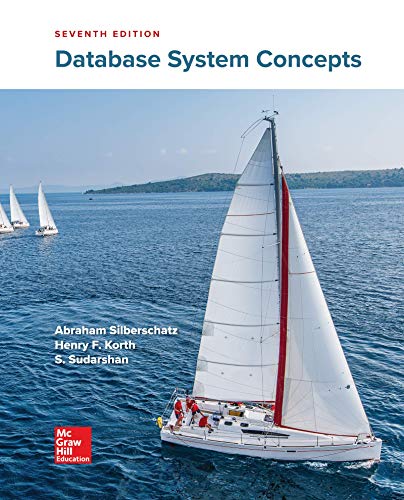
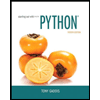
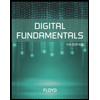
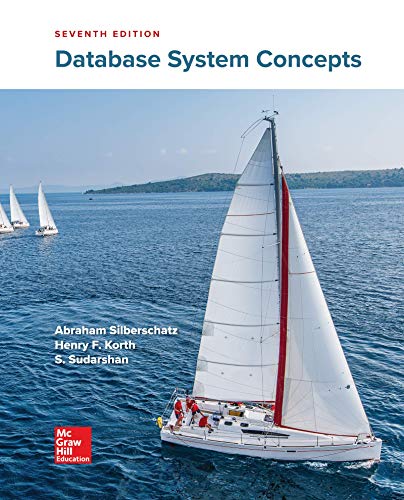
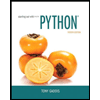
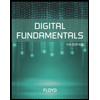
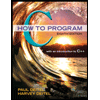
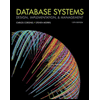
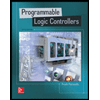