Draw a flow chart according coding statement below. #include #include #include #include using namespace std; //calcAccommodation function int calcAccommodation(char accommodation, int days, char nationality) { int price = 0; //calculate price according to local or international if (nationality == 'L') { price = days * 150; } else { price = days * 100; } return price; } //calcFee Function void calcFee(char category, char nationality, int& fees) { //local presenter or observer if (nationality == 'L') { if (category == 'P') fees = 990; else fees = 600; } //international presenter or observer else { if (category == 'P') fees = 260; else fees = 150; } } //printInvoice function void printInvoice(int No, char name[], char category, char accommodation, int totalCharge, float fees, float price, char nationality) { float tot = 0; //declare output file ofstream output; //open file and check for error output.open("Output.txt", ios::app); if (output.fail()) { cout << "The onput file cannot be opened" << endl; exit(-1); } //total charge if local or international if (nationality == 'L') tot = totalCharge; else tot = totalCharge * 4.2; //output to file output << "------------------------------------------------" << endl; output << "International Conference on" << endl; output << "Mathematical Sciences and Technology (MathTech)" << endl; output << "------------------------------------------------" << endl; output << "\nInvoice Date : 20 January 2020" << endl; output << "Invoice No : " << setw(4) << setfill('0') << No << endl; No++; output << "Name : " << name << endl; if (category == 'L') { output << "Category : " << "Local" << endl; } else { output << "Category : " << "International" << endl; } if (accommodation == 'Y') { output << "Accomodation (Y/N) : " << "Yes" << endl; } else { output << "Accomodation (Y/N) : " << "No" << endl; } output << setiosflags(ios::showpoint); output << fixed << setprecision(2); output << "\nConference Fee : " << fees << endl; output << "Accommodation Fee : " << price << endl; output << "Total Amount Due (RM) : " << tot << endl; output << "\nTerms & Conditions" << endl; output << "Payment is due within 30 days." << endl; output << "Please make payment via online banking to" << endl; output << "Maths USM (RHB: 1234554321)" << endl; output << "\n**************************************************" << endl; output.close(); } int main() { char name[30], accommodation, nationality, category; int days = 0, fees, totalCharge, price = 0, No = 0001; ifstream input; void calcFee(char, char, int&); int calcAccommodation(char, int, char); //open file and check input.open("File.txt"); if (input.fail()) { cout << "The input file cannot be opened" << endl; exit(-1); } //for every entry in the file for (int i = 1; i <= 5; i++) { //get name into name array input.getline(name, 30); //get details into each variables input >> category >> nationality >> accommodation >> days; price = calcAccommodation(accommodation, days, nationality); calcFee(category, nationality, fees); totalCharge = price + fees; printInvoice(No, name, category, accommodation, totalCharge, fees, price, nationality); //ignore the input input.ignore(); //increase invoice number No++; } //close file input.close(); }
Draw a flow chart according coding statement below.
#include <iostream>
#include <iomanip>
#include <fstream>
#include <stdlib.h>
using namespace std;
//calcAccommodation function
int calcAccommodation(char accommodation, int days, char nationality)
{
int price = 0;
//calculate price according to local or international
if (nationality == 'L')
{
price = days * 150;
}
else
{
price = days * 100;
}
return price;
}
//calcFee Function
void calcFee(char category, char nationality, int& fees)
{
//local presenter or observer
if (nationality == 'L')
{
if (category == 'P')
fees = 990;
else
fees = 600;
}
//international presenter or observer
else
{
if (category == 'P')
fees = 260;
else
fees = 150;
}
}
//printInvoice function
void printInvoice(int No, char name[], char category, char accommodation, int totalCharge, float fees, float price, char nationality)
{
float tot = 0;
//declare output file
ofstream output;
//open file and check for error
output.open("Output.txt", ios::app);
if (output.fail())
{
cout << "The onput file cannot be opened" << endl;
exit(-1);
}
//total charge if local or international
if (nationality == 'L')
tot = totalCharge;
else
tot = totalCharge * 4.2;
//output to file
output << "------------------------------------------------" << endl;
output << "International Conference on" << endl;
output << "Mathematical Sciences and Technology (MathTech)" << endl;
output << "------------------------------------------------" << endl;
output << "\nInvoice Date : 20 January 2020" << endl;
output << "Invoice No : " << setw(4) << setfill('0') << No << endl; No++;
output << "Name : " << name << endl;
if (category == 'L') {
output << "Category : " << "Local" << endl;
}
else {
output << "Category : " << "International" << endl;
}
if (accommodation == 'Y') {
output << "Accomodation (Y/N) : " << "Yes" << endl;
}
else {
output << "Accomodation (Y/N) : " << "No" << endl;
}
output << setiosflags(ios::showpoint);
output << fixed << setprecision(2);
output << "\nConference Fee : " << fees << endl;
output << "Accommodation Fee : " << price << endl;
output << "Total Amount Due (RM) : " << tot << endl;
output << "\nTerms & Conditions" << endl;
output << "Payment is due within 30 days." << endl;
output << "Please make payment via online banking to" << endl;
output << "Maths USM (RHB: 1234554321)" << endl;
output << "\n**************************************************" << endl;
output.close();
}
int main()
{
char name[30], accommodation, nationality, category;
int days = 0, fees, totalCharge, price = 0, No = 0001;
ifstream input;
void calcFee(char, char, int&);
int calcAccommodation(char, int, char);
//open file and check
input.open("File.txt");
if (input.fail())
{
cout << "The input file cannot be opened" << endl;
exit(-1);
}
//for every entry in the file
for (int i = 1; i <= 5; i++) {
//get name into name array
input.getline(name, 30);
//get details into each variables
input >> category >> nationality >> accommodation >> days;
price = calcAccommodation(accommodation, days, nationality);
calcFee(category, nationality, fees);
totalCharge = price + fees;
printInvoice(No, name, category, accommodation, totalCharge, fees, price, nationality);
//ignore the input
input.ignore();
//increase invoice number
No++;
}
//close file
input.close();
}

Step by step
Solved in 3 steps with 4 images

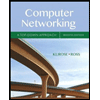
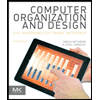
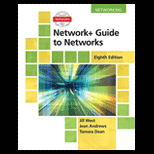
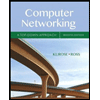
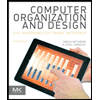
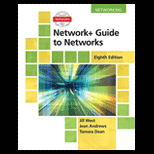
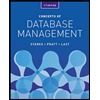
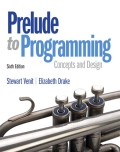
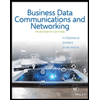