Define the Artist class in Artist.py with a constructor to initialize an artist's information. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0. Define the Artwork class in Artwork.py with a constructor to initialize an artwork's information. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values. Add an import statement to import the Artist class. Add import statements to main.py to import the Artist and Artwork classes. Ex: If the input is: Pablo Picasso 1881 1973 Three Musicians 1921 the output is: Artist: Pablo Picasso (1881-1973) Title: Three Musicians, 1921 If the input is: Brice Marden 1938 -1 Distant Muses 2000 the output is: Artist: Brice Marden, born 1938 Title: Distant Muses, 2000 Code: def __init__(self,aname = "None",abirth = 0,adeath = 0): self.aname = aname self.abirth_year = abirth self.adeath_year = adeath #Define print_info() method def print_info(self): # If death_year is -1 if self.adeath_year == -1: #Print only birth_year print('Artist: {}, born {}'.format(self.aname, self.abirth_year)) else: #Print birth_year and death year print('Artist: {} ({}-{})'.format(self.aname, self.abirth_year, self.adeath_year)) #Create a class class Artist: #Define the constructor def __init__(self,userartist_name = "None",userbirth_year = 0, userdeath_year = 0): self.userartist_name=userartist_name self.userbirth_year=userbirth_year self.userdeath_year=userdeath_year #Define the method def print_info(self): #If death year is -1 if(userdeath_year==-1): #Print artist name, birth_year print("Artist: ",userartist_name,", born ",userbirth_year) else: #Print artist name, birth_year and death year print("Artist: ",userartist_name," (",userbirth_year,"-", userdeath_year,")") #Create a class class Artwork: #Define the constructor def __init__(self,atitle = "None",ayear = 0,anartist = Artist()): self.atitle = atitle self.ayear_created = ayear self.anartist = anartist #Define the method def print_info(self): #Call the method self.anartist.print_info() #Print the title and the year print('Title: %s, %d' % (self.atitle, self.ayear_created)) # Main function if __name__ == "__main__": #Get the artist name userartist_name = input() #Get the birth year userbirth_year = int(input()) #Get the death year userdeath_year = int(input()) #Get the title usertitle = input() #Get the year created useryear_created = int(input()) userartist = Artist(userartist_name, userbirth_year, userdeath_year) new_anartwork = Artwork(usertitle, useryear_created, userartist) #Call the method new_anartwork.print
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Define the Artist class in Artist.py with a constructor to initialize an artist's information. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0.
Define the Artwork class in Artwork.py with a constructor to initialize an artwork's information. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values. Add an import statement to import the Artist class.
Add import statements to main.py to import the Artist and Artwork classes.
Ex: If the input is:
Pablo Picasso 1881 1973 Three Musicians 1921
the output is:
Artist: Pablo Picasso (1881-1973) Title: Three Musicians, 1921
If the input is:
Brice Marden 1938 -1 Distant Muses 2000
the output is:
Artist: Brice Marden, born 1938 Title: Distant Muses, 2000
Code:
def __init__(self,aname = "None",abirth = 0,adeath = 0):
self.aname = aname
self.abirth_year = abirth
self.adeath_year = adeath
#Define print_info() method
def print_info(self):
# If death_year is -1
if self.adeath_year == -1:
#Print only birth_year
print('Artist: {}, born {}'.format(self.aname, self.abirth_year))
else:
#Print birth_year and death year
print('Artist: {} ({}-{})'.format(self.aname, self.abirth_year, self.adeath_year))
#Create a class
class Artist:
#Define the constructor
def __init__(self,userartist_name = "None",userbirth_year = 0, userdeath_year = 0):
self.userartist_name=userartist_name
self.userbirth_year=userbirth_year
self.userdeath_year=userdeath_year
#Define the method
def print_info(self):
#If death year is -1
if(userdeath_year==-1):
#Print artist name, birth_year
print("Artist: ",userartist_name,", born ",userbirth_year)
else:
#Print artist name, birth_year and death year
print("Artist: ",userartist_name," (",userbirth_year,"-", userdeath_year,")")
#Create a class
class Artwork:
#Define the constructor
def __init__(self,atitle = "None",ayear = 0,anartist = Artist()):
self.atitle = atitle
self.ayear_created = ayear
self.anartist = anartist
#Define the method
def print_info(self):
#Call the method
self.anartist.print_info()
#Print the title and the year
print('Title: %s, %d' % (self.atitle, self.ayear_created))
# Main function
if __name__ == "__main__":
#Get the artist name
userartist_name = input()
#Get the birth year
userbirth_year = int(input())
#Get the death year
userdeath_year = int(input())
#Get the title
usertitle = input()
#Get the year created
useryear_created = int(input())
userartist = Artist(userartist_name, userbirth_year, userdeath_year)
new_anartwork = Artwork(usertitle, useryear_created, userartist)
#Call the method
new_anartwork.print_info()


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

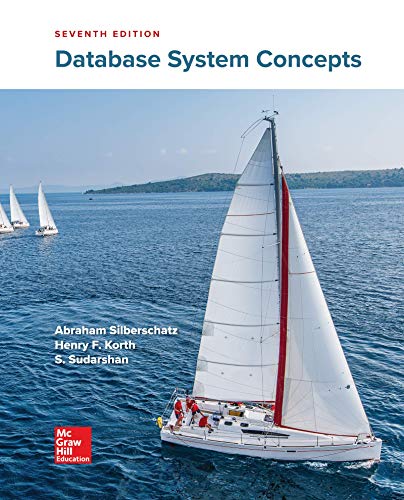
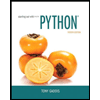
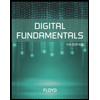
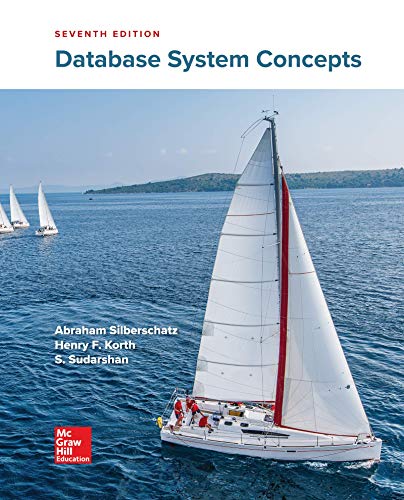
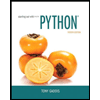
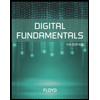
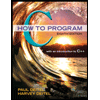
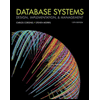
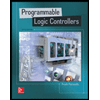