Define the Queue class template in the file DoublyLinkedQueue.cpp. // DoublyLinkedQueue.cpp #ifndef QUEUE_H #define QUEUE_H #include using namespace std; template class Queue { public: // Default constructor Queue() front = new NodeType; back new NodeType; front->next = back; back->prev = front; //Desctructor } ~Queue() { } clear(); delete front; delete back; // Makes the queue to the empty state. void clear() { } // Checks if the queue is empty. bool empty() const { } // Inserts item at the end of the queue. void enqueue(const T& item) { } // Removes the element at the start of the queue. void dequeue() { } // returns the front element const T& front_element () const { { } // Prints the elements of the queue. void print() const Node Type* ptr = front->next; while (ptr = back) { cout << ptr->data << ", ptr = ptr->next; } cout << endl; } private: struct NodeType { T data; Node Type* next; NodeType✶ prev; NodeType(const T & d = T()): data(d), prev(nullptr), next(nullptr) {} }; Node Type* front%3B // points to the header node Node Type back; // points to the tail node }; #endif Please read the comments carefully and implement the Queue template class. The main function is contained in the file lab07.cpp. // lab07.cpp #include "Employee.cpp" #include "DoublyLinkedQueue.cpp" int main() { Queue intQue; int x = 0, k = 0; cout << "Enqueue positive numbers (enter 0 to stop): "; cin >> x; // add your code return 0; } The main function contains 3 parts. A. Part 1: 1. Declares a queue which stores int values. 2. Prompts users to enter integers, enqueues the entered values, stops entering the integers when users enter O. 3. Prints the elements of queue. 4. Prompts the user to enter a number K, and dequeues ✓ values from the front of the queue. 5. Prints the elements of queue. B. Part 2: 1. Declares a queue which stores strings. 2. Prompts users to enter strings, enqueue the entered strings, stops entering the strings when the user enter "exit". 3. Prints the elements of queue. 4. Prompts users to enter a number K, and dequeues K strings from the front of the queue. 5. Prints the elements of queue. C. Part 3: 1. Declares a queue which stores Employee objects. Download Employee class file Employee.cpp ✓ and store it in cse2020/lab07 2. Prompts users to enter employee's id, name, and department name, create an employee object, enqueue the created employee boject, stops entering the employees when the user enter id as O. 3. Prints the elements of queue. 4. Prompts users to enter a number K, and dequeues ✓ employees from the front of the queue. 5. Prints the elements of queue. The expected result: Enqueue positive numbers (enter 0 to stop): 1234567890 print queue: 1, 2, 3, 4, 5, 6, 7, 8, 9, How many numbers to be removed from queue: 5 print queue: 6, 7, 8, 9, Enqueue string (enter exit to stop): aaa bbb ccc ddd eee fff exit print queue: aaa, bbb, ccc, ddd, eee, fff, How many strings to be removed from queue: 3 print queue: ddd, eee, fff, Enqueue employee's id, name, dept (enter id 0 to stop): 1 bob CSE Enqueue employee's id, name, dept: 2 mary Math Enqueue employee's id, name, dept: 3 joe Art Enqueue employee's id, name, dept: 4 Ellen CSE Enqueue employee's id, name, dept: 0 no no print queue: 1 bob CSE, 2 mary Math, 3 joe Art, 4 Ellen CSE, How many strings to be removed from queue: 2 print queue: 3 joe Art, 4 Ellen CSE,
Define the Queue class template in the file DoublyLinkedQueue.cpp. // DoublyLinkedQueue.cpp #ifndef QUEUE_H #define QUEUE_H #include using namespace std; template class Queue { public: // Default constructor Queue() front = new NodeType; back new NodeType; front->next = back; back->prev = front; //Desctructor } ~Queue() { } clear(); delete front; delete back; // Makes the queue to the empty state. void clear() { } // Checks if the queue is empty. bool empty() const { } // Inserts item at the end of the queue. void enqueue(const T& item) { } // Removes the element at the start of the queue. void dequeue() { } // returns the front element const T& front_element () const { { } // Prints the elements of the queue. void print() const Node Type* ptr = front->next; while (ptr = back) { cout << ptr->data << ", ptr = ptr->next; } cout << endl; } private: struct NodeType { T data; Node Type* next; NodeType✶ prev; NodeType(const T & d = T()): data(d), prev(nullptr), next(nullptr) {} }; Node Type* front%3B // points to the header node Node Type back; // points to the tail node }; #endif Please read the comments carefully and implement the Queue template class. The main function is contained in the file lab07.cpp. // lab07.cpp #include "Employee.cpp" #include "DoublyLinkedQueue.cpp" int main() { Queue intQue; int x = 0, k = 0; cout << "Enqueue positive numbers (enter 0 to stop): "; cin >> x; // add your code return 0; } The main function contains 3 parts. A. Part 1: 1. Declares a queue which stores int values. 2. Prompts users to enter integers, enqueues the entered values, stops entering the integers when users enter O. 3. Prints the elements of queue. 4. Prompts the user to enter a number K, and dequeues ✓ values from the front of the queue. 5. Prints the elements of queue. B. Part 2: 1. Declares a queue which stores strings. 2. Prompts users to enter strings, enqueue the entered strings, stops entering the strings when the user enter "exit". 3. Prints the elements of queue. 4. Prompts users to enter a number K, and dequeues K strings from the front of the queue. 5. Prints the elements of queue. C. Part 3: 1. Declares a queue which stores Employee objects. Download Employee class file Employee.cpp ✓ and store it in cse2020/lab07 2. Prompts users to enter employee's id, name, and department name, create an employee object, enqueue the created employee boject, stops entering the employees when the user enter id as O. 3. Prints the elements of queue. 4. Prompts users to enter a number K, and dequeues ✓ employees from the front of the queue. 5. Prints the elements of queue. The expected result: Enqueue positive numbers (enter 0 to stop): 1234567890 print queue: 1, 2, 3, 4, 5, 6, 7, 8, 9, How many numbers to be removed from queue: 5 print queue: 6, 7, 8, 9, Enqueue string (enter exit to stop): aaa bbb ccc ddd eee fff exit print queue: aaa, bbb, ccc, ddd, eee, fff, How many strings to be removed from queue: 3 print queue: ddd, eee, fff, Enqueue employee's id, name, dept (enter id 0 to stop): 1 bob CSE Enqueue employee's id, name, dept: 2 mary Math Enqueue employee's id, name, dept: 3 joe Art Enqueue employee's id, name, dept: 4 Ellen CSE Enqueue employee's id, name, dept: 0 no no print queue: 1 bob CSE, 2 mary Math, 3 joe Art, 4 Ellen CSE, How many strings to be removed from queue: 2 print queue: 3 joe Art, 4 Ellen CSE,
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the full C++ code for the provided output in the screenshot

Transcribed Image Text:Define the Queue class template in the file DoublyLinkedQueue.cpp.
// DoublyLinkedQueue.cpp
#ifndef QUEUE_H
#define QUEUE_H
#include <iostream>
using namespace std;
template<typename T>
class Queue
{
public:
// Default constructor
Queue()
front = new NodeType;
back new NodeType;
front->next = back;
back->prev = front;
//Desctructor
}
~Queue()
{
}
clear();
delete front;
delete back;
// Makes the queue to the empty state.
void clear()
{
}
// Checks if the queue is empty.
bool empty() const
{
}
// Inserts item at the end of the queue.
void enqueue(const T& item)
{
}
// Removes the element at the start of the queue.
void dequeue()
{
}
// returns the front element
const T& front_element () const
{
{
}
// Prints the elements of the queue.
void print() const
Node Type* ptr = front->next;
while (ptr = back)
{
cout << ptr->data << ",
ptr = ptr->next;
}
cout << endl;
}
private:
struct NodeType
{
T data;
Node Type* next;
NodeType✶ prev;
NodeType(const T & d = T()): data(d), prev(nullptr), next(nullptr)
{}
};
Node Type* front%3B // points to the header node
Node Type back; // points to the tail node
};
#endif

Transcribed Image Text:Please read the comments carefully and implement the Queue template class.
The main function is contained in the file lab07.cpp.
// lab07.cpp
#include "Employee.cpp"
#include "DoublyLinkedQueue.cpp"
int main()
{
Queue<int> intQue;
int x = 0, k = 0;
cout << "Enqueue positive numbers (enter 0 to stop): ";
cin >> x;
// add your code
return 0;
}
The main function contains 3 parts.
A. Part 1:
1. Declares a queue which stores int values.
2. Prompts users to enter integers, enqueues the entered values, stops entering the integers when users enter O.
3. Prints the elements of queue.
4. Prompts the user to enter a number K, and dequeues ✓ values from the front of the queue.
5. Prints the elements of queue.
B. Part 2:
1. Declares a queue which stores strings.
2. Prompts users to enter strings, enqueue the entered strings, stops entering the strings when the user enter "exit".
3. Prints the elements of queue.
4. Prompts users to enter a number K, and dequeues K strings from the front of the queue.
5. Prints the elements of queue.
C. Part 3:
1. Declares a queue which stores Employee objects. Download Employee class file Employee.cpp ✓ and store it in cse2020/lab07
2. Prompts users to enter employee's id, name, and department name, create an employee object, enqueue the created employee boject, stops entering the employees when the user enter id as O.
3. Prints the elements of queue.
4. Prompts users to enter a number K, and dequeues ✓ employees from the front of the queue.
5. Prints the elements of queue.
The expected result:
Enqueue positive numbers (enter 0 to stop): 1234567890
print queue: 1, 2, 3, 4, 5, 6, 7, 8, 9,
How many numbers to be removed from queue: 5
print queue: 6, 7, 8, 9,
Enqueue string (enter exit to stop): aaa bbb ccc ddd eee fff exit
print queue: aaa, bbb, ccc, ddd, eee, fff,
How many strings to be removed from queue: 3
print queue: ddd, eee, fff,
Enqueue employee's id, name, dept (enter id 0 to stop): 1 bob CSE
Enqueue employee's id, name, dept: 2 mary Math
Enqueue employee's id, name, dept: 3 joe Art
Enqueue employee's id, name, dept: 4 Ellen CSE
Enqueue employee's id, name, dept: 0 no no
print queue: 1 bob CSE, 2 mary Math, 3 joe Art, 4 Ellen CSE,
How many strings to be removed from queue: 2
print queue: 3 joe Art, 4 Ellen CSE,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
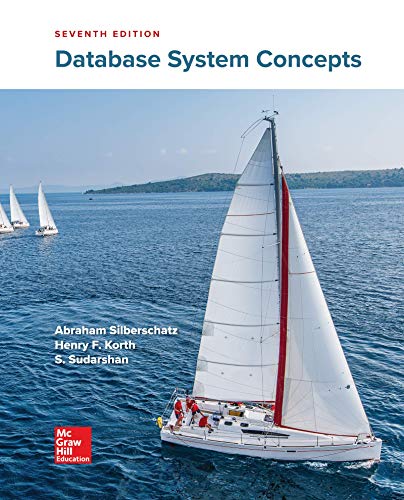
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
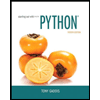
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
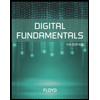
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
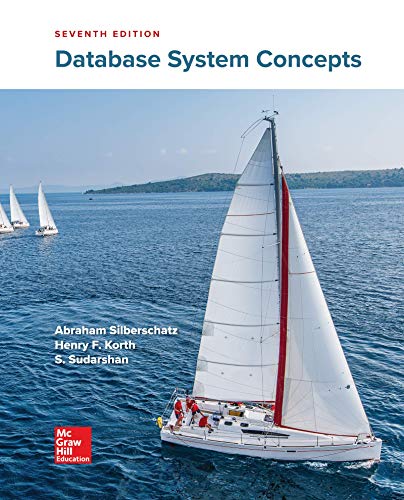
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
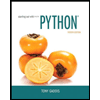
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
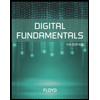
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
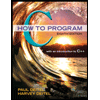
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
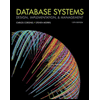
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
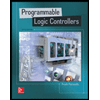
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education