Define the instance method inc_num_kids() for PersonInfo. inc_num_kids increments the member data num_kids. Sample output for the given program with one call to inc_num_kids():
Define the instance method inc_num_kids() for PersonInfo. inc_num_kids increments the member data num_kids. Sample output for the given program with one call to inc_num_kids():
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%

Transcribed Image Text:### Python Class and Method Example
In this example, we are defining a class `PersonInfo` with an instance method `inc_num_kids()` that increments the member data `num_kids`.
#### Code Explanation:
```python
class PersonInfo:
def __init__(self):
self.num_kids = 0
# FIXME: Write inc_num_kids(self)
def inc_num_kids(self):
self.num_kids += 1
person1 = PersonInfo()
print(f'Kids: {person1.num_kids}')
person1.inc_num_kids()
print(f'New baby, kids now: {person1.num_kids}')
```
- **Class Definition**: The class `PersonInfo` has an initializer method `__init__()` that sets `num_kids` to 0.
- **Method `inc_num_kids()`**: This method, when called, will increment the `num_kids` attribute by 1.
- **Object Creation**: `person1` is an instance of `PersonInfo`.
- **Output Statements**: The program prints the number of kids before and after calling `inc_num_kids()`.
#### Error Message:
- **Test Error**: The program encountered an error, indicated by:
```
Exited with return code 1.
Traceback (most recent call last):
File "main.py", line 11, in <module>
person1.inc_num_kids()
AttributeError: 'PersonInfo' object has no attribute 'inc_num_kids'
```
This indicates that there was an attempt to call a non-existing method `inc_num_kids()` on the `person1` object, which suggests that the method definition might not have been recognized due to outdated code or a missed update.
#### Correction Notes:
- Ensure the method `inc_num_kids` is correctly defined and attributed to the class `PersonInfo`.
- Double-check that there are no syntax errors preventing the code from recognizing the method.
Understanding these basic principles will help in addressing similar issues in object-oriented programming with Python.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
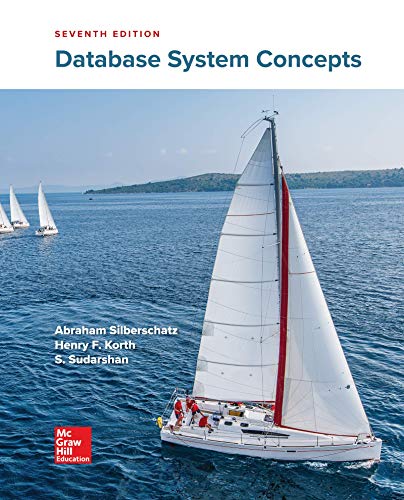
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
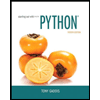
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
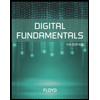
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
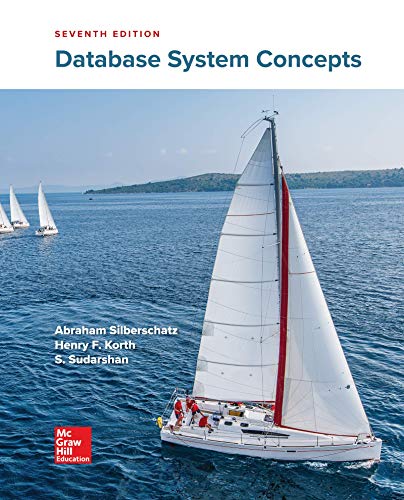
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
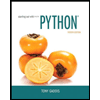
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
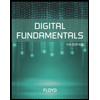
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
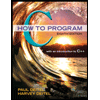
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
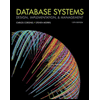
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
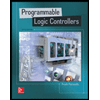
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education