Define the class RectangularCube in C++. A RectangularCube has three sides length(L), width (W), and Height (h), define the sides as private data fields. Include a no-argument constructor that sets all three sides to 1. The class must also have a constructor that receive parameters to initialize sides of the RectangularCube. Include get and set functions for all three data fields. Finally define the functions volume () and surfaceArea () in the class RectangularCube that use the formulas given below to calculate and return the volume and the surface area of the RectangularCube. Give the UML diagram of the class, you may use the MSOffice/Visio to create the UML class diagram (best to download and use Visual paradigm tool, or any other free UML tool). Implement the class using separate files for definition and implementation, use inclusion guard to avoid duplicate code. Make sure to include at least one inline function implementation. Do not Implement the volume () and the SurfaceArea () as inline Include comments in your code. Write a test function for the class RectangularCube and perform the following tasks: Create two objects, one object using the no-argument constructor and another using the constructor with parameters (pick any values for the L, W, and H). Invoke the volume () and SurfaceArea () functions on both object and display their returned value. Invoke a set function on an object and change the value of one of the sides. Invoke the volume () and surfaceArea () functions on the object in part (c) and print their returned values. Assign (using the operator =) and anonymous RectanularCube object to the object in part (c). Once again invoke the volume () and surfaceArea () functions on the object in part (c). Code submission and sample output. In this problem we will continue to expand on the initial problem Write the function with the header void printCube (RectangularCube rc) that receives an object of the type RectangularCube and displays the length, width, height, volume and the surface area of the cube like: L = 2, W = 1, H = 1, V = 2, A = 8. This function should be implemented as a non-member function, meaning that it should be defined outside the class RectangularCube. Write a main function in which you declare an array of 5 RectangularCube objects. Inside the main function, use a loop to initialize/populate this array of RectangularCube. In the body of the loop use the rand () function to generate random integers between 1 and 10 to assign to length, width and height data fields of each RectangularCube object After populating the array, use another loop to display individual elements of the array in part (a) by calling the function printCube in part (a) Code submission and sample output
Define the class RectangularCube in C++. A RectangularCube has three sides length(L), width (W), and Height (h), define the sides as private data fields. Include a no-argument constructor that sets all three sides to 1. The class must also have a constructor that receive parameters to initialize sides of the RectangularCube. Include get and set functions for all three data fields. Finally define the functions volume () and surfaceArea () in the class RectangularCube that use the formulas given below to calculate and return the volume and the surface area of the RectangularCube. Give the UML diagram of the class, you may use the MSOffice/Visio to create the UML class diagram (best to download and use Visual paradigm tool, or any other free UML tool). Implement the class using separate files for definition and implementation, use inclusion guard to avoid duplicate code. Make sure to include at least one inline function implementation. Do not Implement the volume () and the SurfaceArea () as inline Include comments in your code. Write a test function for the class RectangularCube and perform the following tasks: Create two objects, one object using the no-argument constructor and another using the constructor with parameters (pick any values for the L, W, and H). Invoke the volume () and SurfaceArea () functions on both object and display their returned value. Invoke a set function on an object and change the value of one of the sides. Invoke the volume () and surfaceArea () functions on the object in part (c) and print their returned values. Assign (using the operator =) and anonymous RectanularCube object to the object in part (c). Once again invoke the volume () and surfaceArea () functions on the object in part (c). Code submission and sample output. In this problem we will continue to expand on the initial problem Write the function with the header void printCube (RectangularCube rc) that receives an object of the type RectangularCube and displays the length, width, height, volume and the surface area of the cube like: L = 2, W = 1, H = 1, V = 2, A = 8. This function should be implemented as a non-member function, meaning that it should be defined outside the class RectangularCube. Write a main function in which you declare an array of 5 RectangularCube objects. Inside the main function, use a loop to initialize/populate this array of RectangularCube. In the body of the loop use the rand () function to generate random integers between 1 and 10 to assign to length, width and height data fields of each RectangularCube object After populating the array, use another loop to display individual elements of the array in part (a) by calling the function printCube in part (a) Code submission and sample output
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Define the class RectangularCube in C++. A RectangularCube has three sides length(L), width (W), and Height (h), define the sides as private data fields. Include a no-argument constructor that sets all three sides to 1. The class must also have a constructor that receive parameters to initialize sides of the RectangularCube. Include get and set functions for all three data fields. Finally define the functions volume () and surfaceArea () in the class RectangularCube that use the formulas given below to calculate and return the volume and the surface area of the RectangularCube.
- Give the UML diagram of the class, you may use the MSOffice/Visio to create the UML class diagram (best to download and use Visual paradigm tool, or any other free UML tool).
- Implement the class using separate files for definition and implementation, use inclusion guard to avoid duplicate code. Make sure to include at least one inline function implementation. Do not Implement the volume () and the SurfaceArea () as inline Include comments in your code.
- Write a test function for the class RectangularCube and perform the following tasks:
- Create two objects, one object using the no-argument constructor and another using the constructor with parameters (pick any values for the L, W, and H).
- Invoke the volume () and SurfaceArea () functions on both object and display their returned value.
- Invoke a set function on an object and change the value of one of the sides.
- Invoke the volume () and surfaceArea () functions on the object in part (c) and print their returned values.
- Assign (using the operator =) and anonymous RectanularCube object to the object in part (c). Once again invoke the volume () and surfaceArea () functions on the object in part (c).
- Code submission and sample output.
In this problem we will continue to expand on the initial problem
- Write the function with the header void printCube (RectangularCube rc) that receives an object of the type RectangularCube and displays the length, width, height, volume and the surface area of the cube like: L = 2, W = 1, H = 1, V = 2, A = 8. This function should be implemented as a non-member function, meaning that it should be defined outside the class RectangularCube.
- Write a main function in which you declare an array of 5 RectangularCube objects. Inside the main function, use a loop to initialize/populate this array of RectangularCube. In the body of the loop use the rand () function to generate random integers between 1 and 10 to assign to length, width and height data fields of each RectangularCube object
- After populating the array, use another loop to display individual elements of the array in part (a) by calling the function printCube in part (a)
- Code submission and sample output
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
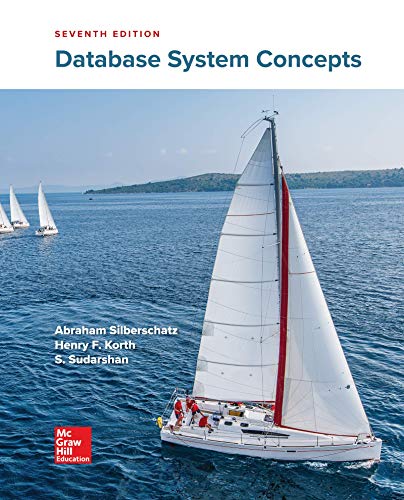
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
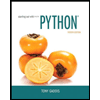
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
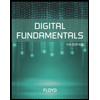
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
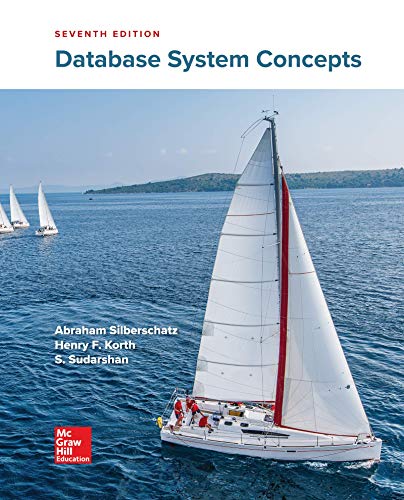
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
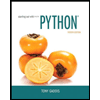
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
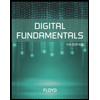
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
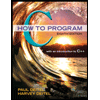
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
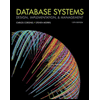
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
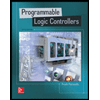
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education