/** * Define string that prints correctly formatted multiplication table with * header and column prefix. Add --- and | delimiters between prefix and * table data * * You may assume table has at least 1 row with 1 element * * Use System.lineSeparator() to delimit individual lines * * | 0, 1, 2, 3, 4, 5 --------------------------- 0| 0 1| 0, 1 2| 0, 2, 4 3| 0, 3, 6, 9 4| 0, 4, 8, 12, 16 5| 0, 5, 10, 15, 20, 25 * * * @param table * 2D array of integers (not rectangular!) * @return String as formatted multiplication table */ public static String tableToString(int[][] table) {
/**
* Define string that prints correctly formatted multiplication table with
* header and column prefix. Add --- and | delimiters between prefix and
* table data
*
* You may assume table has at least 1 row with 1 element
*
* Use System.lineSeparator() to delimit individual lines
*
* <pre>
| 0, 1, 2, 3, 4, 5
---------------------------
0| 0
1| 0, 1
2| 0, 2, 4
3| 0, 3, 6, 9
4| 0, 4, 8, 12, 16
5| 0, 5, 10, 15, 20, 25
* </pre>
*
* @param table
* 2D array of integers (not rectangular!)
* @return String as formatted multiplication table
*/
public static String tableToString(int[][] table) {
![/**
* Define string that prints correctly formatted multiplication table with
* header and column prefix. Add --- and | delimiters between prefix and
* table data
* You may assume table has at least 1 row with 1 element
* Use System.lineSeparator () to delimit individual lines
*
<pre>
Г о, 1, 2, 3, 4, 5
0| 0
1|
2|
1
0,
3|
2,
3,
4
0,
4||
6,
9
0, 4, 8, 12, 16
5|
0, 5, 10, 15, 20, 25
* </pre>
*
* @param table
2D array of integers (not rectangular!)
@return String as formatted multiplication table
*/
public static String tableToString(int[][] table) {
*
return null;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F238fed3b-2fcf-40ca-a8e1-295985b4dcab%2Feed4b6e4-0c3b-4b68-95ec-8d93beccf9d4%2F1oimy4_processed.png&w=3840&q=75)

Solution:
Implementation of tableToString(int[][] table) Method:
public static String tableToString(int[][] table){
String str = "";
//iterating the table from 0 to number of rows
for(int i =0;i<table.length;i++ ){
//iterating for columns
for(int j=0;j<table[0].length;j++){
//appending formatted string in str
str += String.valueOf(j)+"| ";
for(int k=0;k<=j;k++){
if(k>=0 && k<j){
str += String.valueOf(j*k)+", ";
}
else{
str += String.valueOf(j*k);
}
}
//using System.lineSeparator() to seperate the line
str += System.lineSeparator();
}
}
//return the str
return str;
}
Step by step
Solved in 4 steps with 3 images

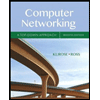
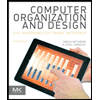
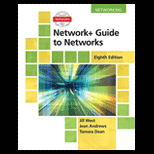
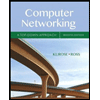
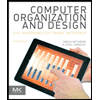
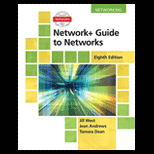
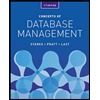
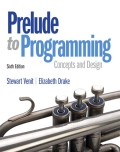
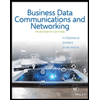