Define a function named coin_flip that returns "Heads" or "Tails" according to a random value 1 or 0. Assume the value 1 represents "Heads" and 0 represents "Tails". Then, write a main program that reads the desired number of coin flips as an input, calls function coin_flip() repeatedly according to the number of coin flips, and outputs the results. Assume the input is a value greater than 0. here is my code: import random # Define your function here def coin_flip(number_of_flips): heads_tails = [0, 1] count = 0 while count != number_of_flips: end_value = random.choice(heads_tails) if end_value == 1: print('Heads') count += 1 else: print('Tails') count += 1 return if __name__ == '__main__': random.seed(5) number_of_flips = int(input()) flips = number_of_flips coin_flip(flips) i keep getting these errors
Define a function named coin_flip that returns "Heads" or "Tails" according to a random value 1 or 0. Assume the value 1 represents "Heads" and 0 represents "Tails". Then, write a main
here is my code:
import random
# Define your function here
def coin_flip(number_of_flips):
heads_tails = [0, 1]
count = 0
while count != number_of_flips:
end_value = random.choice(heads_tails)
if end_value == 1:
print('Heads')
count += 1
else:
print('Tails')
count += 1
return
if __name__ == '__main__':
random.seed(5)
number_of_flips = int(input())
flips = number_of_flips
coin_flip(flips)
i keep getting these errors


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

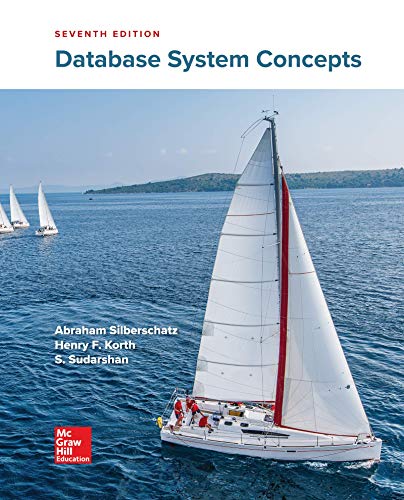
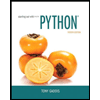
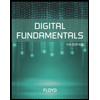
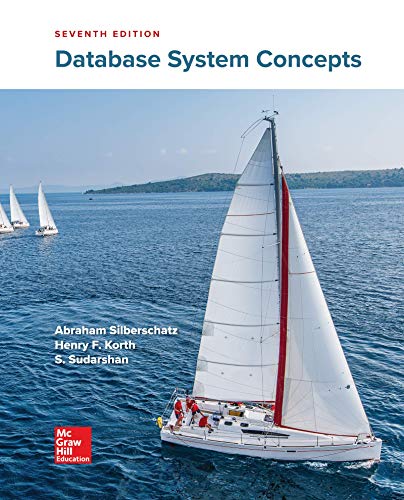
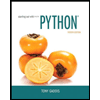
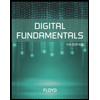
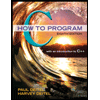
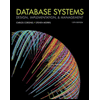
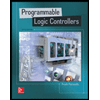