def update_task(task_list, task_id, task_field, task_update): """ Given * the task list (`task_list`) * the task index that has been selected (`task_id`) * the field of the selected task (`task_field`) * the updated information (`task_update`) Validate the parameters to check for syntax and structure. If all validations passed, return a tuple with a boolean True and the updated task (a dictionary from the `task_list` at the provided `task_id`). Ff validations fail, return a tuple with a boolean False and a string with the task_field that caused the error during validation. """ task_list = input() task_id = input() task_field = input() task_update = input() if task_id is is_valid_index(): if (task_field is in task_list.item()): return True , task_list(task_id) else: return (False , "field") else: return (False, "idx") fields = [ 'name', 'description', 'deadline', 'priority', 'completed' ] #you may use this to validate field_name
def update_task(task_list, task_id, task_field, task_update):
""" Given
* the task list (`task_list`)
* the task index that has been selected (`task_id`)
* the field of the selected task (`task_field`)
* the updated information (`task_update`)
Validate the parameters to check for syntax and structure.
If all validations passed, return a tuple with a boolean True and
the updated task (a dictionary from the `task_list` at the provided `task_id`).
Ff validations fail, return a tuple with a boolean False and
a string with the task_field that caused the error during validation.
"""
task_list = input()
task_id = input()
task_field = input()
task_update = input()
if task_id is is_valid_index():
if (task_field is in task_list.item()):
return True , task_list(task_id)
else:
return (False , "field")
else:
return (False, "idx")
fields = [
'name',
'description',
'deadline',
'priority',
'completed'
] #you may use this to validate field_name
if task_field == 'name': # use the correct function call(s)
if ...:#validate update using the correct function call(s)
return #TODO: return the necessary tuple
else:
return #TODO: update the task list accordingly
elif... #TODO: continue checking the next field
return True #TODO: return the proper value if all validations passed
if __name__ == "__main__":
# for testing purposes, so you can observe the output
my_list = [{
'name': 'get groceries',
'description': 'buy some jam and peanut butter',
'deadline': '02/23/2022',
'priority': 2,
'completed': False
},
{
'name': 'get some sleep',
'description': '8 hours of sleep is necessary',
'deadline': '02/03/2022',
'priority': 3,
'completed': True
},
{
'name': 'compar. lit essay',
'description': "finish comparative lit essay that's overdue",
'deadline': '02/15/2022',
'priority': 4,
'completed': True
}]
result = update_task(my_list, '5', 'name', 'go clubbing')
assert result[1] == "idx"
assert result[0] == False
print(f"--> update_task(my_list, '5', 'name', 'go clubbing') "+
f"successfully returned error with `{result[1]}`\n")
result = update_task(my_list, '1', 'Get Gift', 'I\'m quite hungry though')
assert result[1] == "field"
assert result[0] == False
print(f"--> update_task(my_list, '1', 'Get Gift', 'I\'m quite hungry though') "+
f"successfully returned error with `{result[1]}`\n")
result = update_task(my_list, '1', 'deadline', 'never')
assert result[1] == "deadline"
assert result[0] == False
print(f"--> update_task(my_list, '1', 'deadline', 'never') "+
f"successfully returned error with `{result[1]}`\n")
result = update_task(my_list, '1', 'priority', 'pants on FIRE!!!!')
assert result[1] == "priority"
assert result[0] == False
print(f"--> update_task(my_list, '1', 'priority', 'pants on FIRE!!!!') "+
f"successfully returned error with `{result[1]}`\n")
result = update_task(my_list, '1', 'completed', 'technically, yes')
assert result[1] == "completed"
assert result[0] == False
print(f"--> update_task(my_list, '1', 'completed', 'technically, yes') "+
f"successfully returned error with `{result[1]}`\n")
result = update_task(my_list, '1', 'deadline', '01/22/19')
assert result[0] == True
assert result[1]['deadline'] == '01/22/19'
print(f"--> update_task(my_list, '1', 'Deadline', '01/22/19' "+
f"successfully returned updated task: n{result[1]}n")
result = update_task(my_list, '1', 'completed', 'no')
assert result[0] == True
assert result[1]['completed'] == False
print(f"--> update_task(my_list, '1', 'completed', 'no') "+
f"successfully returned updated task: \n{result[1]}\n")
print(">>All assertions successfully run...\n")

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

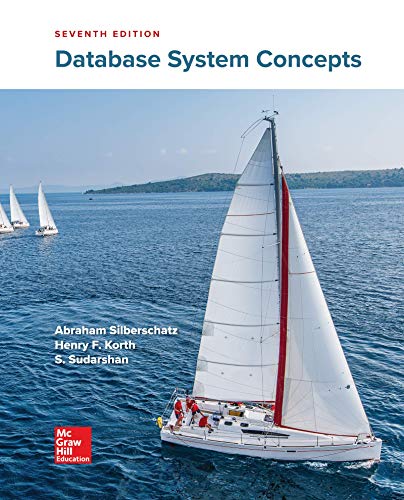
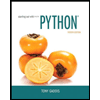
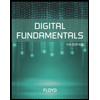
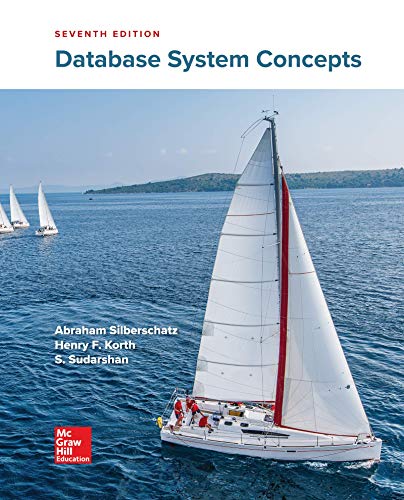
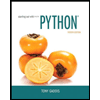
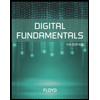
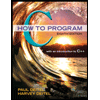
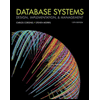
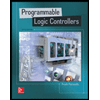