Declare and define a function that computes bonus for an employee depending on the base salary and the number of years of experience of the employee. Inside the function, check the number of years of experience. If the number of years of experience is greater than 10 years, the bonus is 10% of the base salary, otherwise it is 5% of the base salary. You will need to call this function from your main function and pass the base salary and the number of years as arguments. Code: #include using namespace std; double compute_bonus(double base_salary, int experience); int main() { double base_salary; int experience; //get values of base_salary //get experience from user //call compute_bonus by passing necessary parameters //display the bonus returned by the function return 0; } //function definition { double bonus; //compare experience/calculate bonus //return computed bonus }
Declare and define a function that computes bonus for an employee depending on
the base salary and the number of years of experience of the employee.
Inside the function, check the number of years of experience. If the number of years
of experience is greater than 10 years, the bonus is 10% of the base salary, otherwise
it is 5% of the base salary. You will need to call this function from your main function
and pass the base salary and the number of years as arguments.
Code:
#include <iostream>
using namespace std;
double compute_bonus(double base_salary, int experience);
int main()
{
double base_salary;
int experience;
//get values of base_salary
//get experience from user
//call compute_bonus by passing necessary parameters
//display the bonus returned by the function
return 0;
}
//function definition
{
double bonus;
//compare experience/calculate bonus
//return computed bonus
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

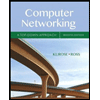
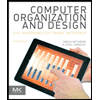
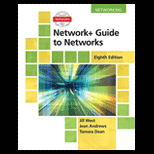
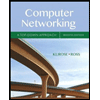
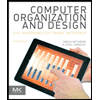
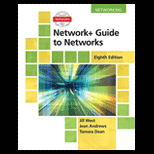
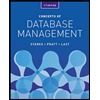
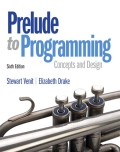
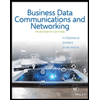