Declare a Boolean variable named valid Password. Use valid Password to output "Valid" if passwordStr contains at least 4 letters and passwordStr's length is less than or equal to 10. Otherwise, output "Invalid". Ex: If the input is 81v4b532YZ, then the output is: Valid Ex: If the input is wH4q1pkøbL2, then the output is: Invalid Note: isalpha() returns true if a character is a letter, and false otherwise. Ex: isalpha('a') returns true. isalpha('8') returns false. 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 T 12 13 14 15 } 16 string passwordStr; /* Additional variable declarations go here */ getline (cin, passwordStr); /* Type your additional code here */ if (validPassword) { 869. cout << "Valid" << endl; else { 17 18 } cout << "Invalid" << endl;
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

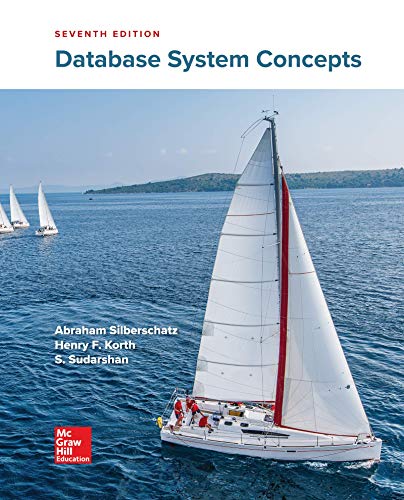
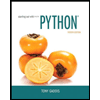
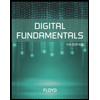
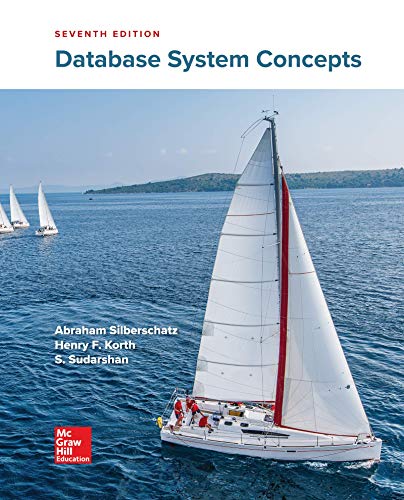
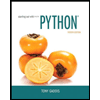
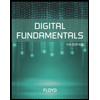
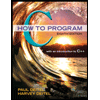
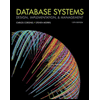
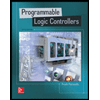