*Data Structures And Algorithm (C Programming) You are going to make C program to solve the general k2 − 1 puzzle. Your program will read an initial state for a k × k table, calculate (preferably minimum number of) steps taking player from initial state to the goal state, and print the solution into an output file.This is a graph search problem and can be solved using several different methods. You will implement Breadth First Search (BFS).
*Data Structures And
You are going to make C program to solve the general k2 − 1 puzzle. Your program will read an initial state for a k × k table, calculate (preferably minimum number of) steps taking player from initial state to the goal state, and print the solution into an output file.This is a graph search problem and can be solved using several different methods. You will implement Breadth First Search (BFS).
Files to work with:
starter.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char **argv)
{
FILE *fp_in,*fp_out;
fp_in = fopen(argv[1], "r");
if (fp_in == NULL){
printf("Could not open a file.\n");
return -1;
}
fp_out = fopen(argv[2], "w");
if (fp_out == NULL){
printf("Could not open a file.\n");
return -1;
}
char *line = NULL;
size_t lineBuffSize = 0;
ssize_t lineSize;
int k;
getline(&line,&lineBuffSize,fp_in);//ignore the first line in file, which is a comment
fscanf(fp_in,"%d\n",&k);//read size of the board
//printf("k = %d\n", k); //make sure you read k properly for DEBUG purposes
getline(&line,&lineBuffSize,fp_in);//ignore the second line in file, which is a comment
int initial_board[k*k];//get kxk memory to hold the initial board
for(int i=0;i<k*k;i++)
fscanf(fp_in,"%d ",&initial_board[i]);
//printBoard(initial_board, k);//Assuming that I have a function to print the board, print it here to make sure I read the input board properly for DEBUG purposes
fclose(fp_in);
////////////////////
// do the rest to solve the puzzle
////////////////////
//once you are done, you can use the code similar to the one below to print the output into file
//if the puzzle is NOT solvable use something as follows
fprintf(fp_out, "#moves\n");
fprintf(fp_out, "no solution\n");
//if it is solvable, then use something as follows:
fprintf(fp_out, "#moves\n");
//probably within a loop, or however you stored proper moves, print them one by one by leaving a space between moves, as below
for(int i=0;i<numberOfMoves;i++)
fprintf(fp_out, "%d ", move[i]);
fclose(fp_out);
return 0;
}
makefile
SOURCE := main.c
CC := gcc
TARGET := solve
all: $(TARGET)
$(TARGET): $(SOURCE)
$(CC) -o $(TARGET) $(SOURCE)
clean:
rm -f $(TARGET)
3boardEasy.txt
#k
3
#initial state
1 0 3 4 2 6 7 5 8
3boardEasyOut.txt
moves
2 5 8
3boardHard.txt
#k
3
#initial state
1 2 8 4 5 0 7 6 3
3boardHardOut.txt
#moves
8 2 5 8 3 6 8 5 2 3 6



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

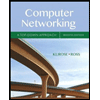
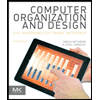
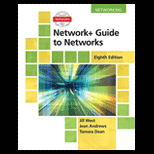
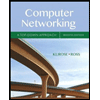
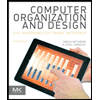
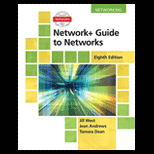
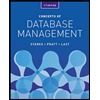
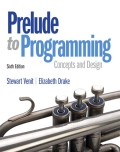
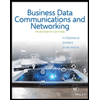