d help creating 2 function that will return the front and rear element of a circular queue in C++ for example: string frontEle(); string rearEle(); these 2 function should return a string of the element at their position and can be store in a variable inside main Here is my code currently: #include #include #define SIZE 5 using namespace std; class Queue { private: string items[SIZE]; int front, rear; int size = 0; public: Queue() { front = -1; rear = -1; } // Check if the queue is full bool isFull() { if (front == 0 && rear == SIZE - 1) { return true; } if (front == rear + 1) {
I need help creating 2 function that will return the front and rear element of a circular queue in C++
for example:
string frontEle();
string rearEle();
these 2 function should return a string of the element at their position and can be store in a variable inside main
Here is my code currently:
#include <iostream>
#include <string>
#define SIZE 5
using namespace std;
class Queue
{
private:
string items[SIZE];
int front, rear;
int size = 0;
public:
Queue()
{
front = -1;
rear = -1;
}
// Check if the queue is full
bool isFull()
{
if (front == 0 && rear == SIZE - 1)
{
return true;
}
if (front == rear + 1)
{
return true;
}
return false;
}
// Check if the queue is empty
bool isEmpty()
{
if (front == -1)
{
return true;
}
else
{
return false;
}
}
// Adding an element
void enQueue(string element)
{
if (isFull())
{
cout << "Queue is full" << endl;
}
else
{
if (front == -1)
{
front = 0;
}
rear = (rear + 1) % SIZE;
items[rear] = element;
cout << endl << "Inserted " << element << endl;
size++;
}
}
//
// Removing an element
string deQueue()
{
string element;
if (isEmpty())
{
cout << "Queue is empty" << endl;
return 0;
}
else
{
element = items[front];
if (front == rear)
{
front = -1;
rear = -1;
}
// Q has only one element,
// so we reset the queue after deleting it.
else
{
front = (front + 1) % SIZE;
}
size--;
return element;
}
}
int arraysize()
{
return size;
}
};
int main()
{
Queue q;
q.enQueue("a");
q.enQueue("b");
q.enQueue("c");
q.enQueue("d");
q.enQueue("e");
// Fails to enqueue because front == 0 && rear == SIZE - 1
q.enQueue("f");
string elem = q.deQueue();
cout << elem << endl;
q.enQueue("f");
int s = q.arraysize();
cout << s << endl;
// Fails to enqueue because front == rear + 1
q.enQueue("g");
return 0;
}

Step by step
Solved in 2 steps

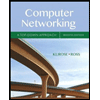
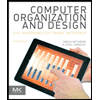
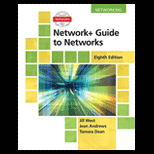
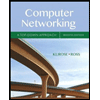
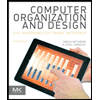
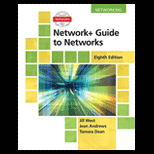
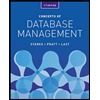
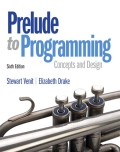
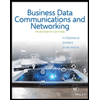