cstrings.cpp #include #include using namespace std; 1 3 4 int main() { const int MAX = 10; char cstr[MAX] = "bubble"; const char* a = "talent"; 7 8 9 10 strcat(cstr, a); 11 cout « "cstr->" « cstr <« endl; } 12
cstrings.cpp #include #include using namespace std; 1 3 4 int main() { const int MAX = 10; char cstr[MAX] = "bubble"; const char* a = "talent"; 7 8 9 10 strcat(cstr, a); 11 cout « "cstr->" « cstr <« endl; } 12
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Running program with substitutions
a
Actual
Expected
cstr->bubbleremind
fail
"remind"
cstr->bubbleremi
*** stack smashing detected ***: terminated
cstr->bubbletrust
fail
"trust"
cstr->bubbletrus
*** stack smashing detected ***: terminated
pass "wait"
cstr->bubblewait
cstr->bubblewait
![The following code doesn't always work. Can you fix it so that it does?
cstrings.cpp
1
#include <iostream>
#include <cstring>
using namespace std;
3
4
int main()
{
const int MAX = 10;
char cstr[MAX] = "bubble";
const char* a = "talent";
7
8
9.
strcat (cstr, a);
cout <« "cstr->" « cstr « endl;
10
11
12
CodeCheck
Reset](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2f0d11ad-d1e5-4d2b-a52c-9bd1292a7fad%2F87a8f1ab-f016-4bea-ab48-07f66d211c6d%2Faivyow_processed.png&w=3840&q=75)
Transcribed Image Text:The following code doesn't always work. Can you fix it so that it does?
cstrings.cpp
1
#include <iostream>
#include <cstring>
using namespace std;
3
4
int main()
{
const int MAX = 10;
char cstr[MAX] = "bubble";
const char* a = "talent";
7
8
9.
strcat (cstr, a);
cout <« "cstr->" « cstr « endl;
10
11
12
CodeCheck
Reset
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
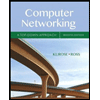
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
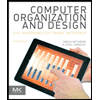
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
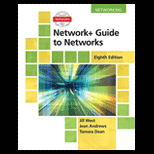
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
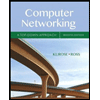
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
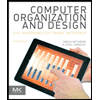
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
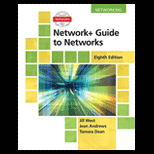
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
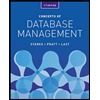
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
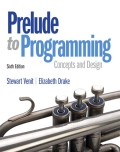
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
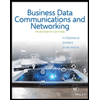
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY