Create the inheritance hierarchy displayed below. The Student class has name(string) as a private data member. The UndergraduateStudent class has private data member rank(string) that can be either freshman, sophmore, junior or senior. The GraduateStudent has private data member project (string). Include constrcutor and get/set functions as well as a print function in all classes. Test the class hierarchy by demonstrating polymorphic behiavior by decalring a vector containing Student, UndergraduatStudent, and GraduateStudent. Process the vector elements by calling the print function on each object in the array. Student Undergraduate Student Graduate Student
Create the inheritance hierarchy displayed below. The Student class has name(string) as a private data member. The UndergraduateStudent class has private data member rank(string) that can be either freshman, sophmore, junior or senior. The GraduateStudent has private data member project (string). Include constrcutor and get/set functions as well as a print function in all classes. Test the class hierarchy by demonstrating polymorphic behiavior by decalring a vector containing Student, UndergraduatStudent, and GraduateStudent. Process the vector elements by calling the print function on each object in the array. Student Undergraduate Student Graduate Student
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question

Transcribed Image Text:**Creating an Inheritance Hierarchy in Programming**
### Overview
The task is to create an inheritance hierarchy based on the design in the diagram. The hierarchy consists of a base class and two derived classes, each with specific attributes and functionalities.
### Class Descriptions
1. **Student Class**
- **Attributes:**
- `name (string)`: A private data member to store the student's name.
- **Functions:**
- Include a constructor for initialization.
- Get and set functions for accessing and updating the `name`.
- A print function to display the student's information.
2. **UndergraduateStudent Class (Derived from Student)**
- **Attributes:**
- `rank (string)`: A private data member that can be one of the following—freshman, sophomore, junior, or senior.
- **Functions:**
- Include a constructor to initialize data members.
- Get and set functions for accessing and updating the `rank`.
- Override the print function to display undergraduate student details.
3. **GraduateStudent Class (Derived from Student)**
- **Attributes:**
- `project (string)`: A private data member to describe the student's project.
- **Functions:**
- Include a constructor to initialize data members.
- Get and set functions for accessing and updating the `project`.
- Override the print function to display graduate student details.
### Implementation
- **Polymorphic Behavior:**
- Demonstrate polymorphism by creating a vector containing objects of `Student`, `UndergraduateStudent`, and `GraduateStudent`.
- Process each element in the vector by calling the print function, showcasing dynamic binding.
### Diagram Explanation
The diagram is a simple representation of class inheritance:
- **Student** is the base class.
- **UndergraduateStudent** and **GraduateStudent** are derived classes, each inheriting from the `Student` class.
- This hierarchy illustrates the "is-a" relationship central to object-oriented design, where both Undergraduate and Graduate students are types of Students.
By following this structure, one can efficiently manage and extend student-related functionalities in an educational software system.
Expert Solution

Step 1: Algorithm:
- Start.
- Include necessary header files:
<iostream>
and<vector>
. - Define a
Student
class with a private membername
, a constructor, and methods to get and set the name, and a virtualprint
method. - Define an
UndergraduateStudent
the class that inherits fromStudent
, with an additionalrank
member and methods to get and set the rank, and an overriddenprint
method. - Define a
GraduateStudent
the class that inherits fromStudent
, with an additionalproject
member and methods to get and set the project, and an overriddenprint
method. - In the
main
function, create a vector of pointers toStudent
objects. - Add instances of
Student
,UndergraduateStudent
, andGraduateStudent
to the vector. - Loop through the vector and call the
print
method for each student, which dynamically dispatches to the appropriate class'sprint
method due to polymorphism. - Clean up the allocated memory by deleting the objects in the vector.
- Return 0 to indicate successful execution.
- End.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
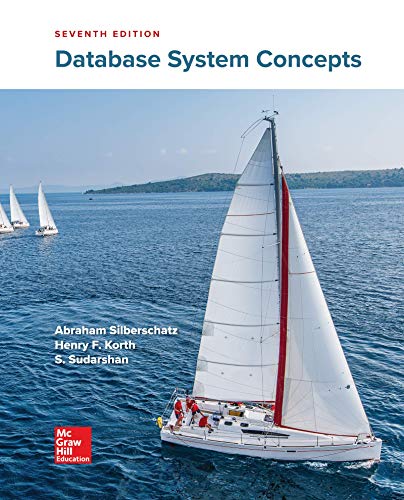
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
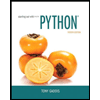
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
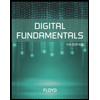
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
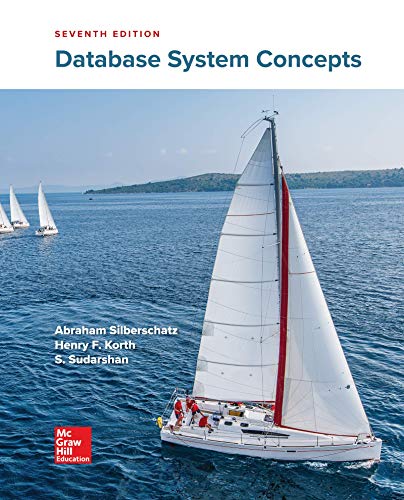
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
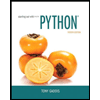
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
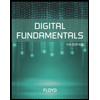
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
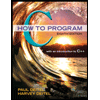
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
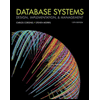
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
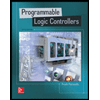
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education