Create an HLA function that forces a value into all three passed parameters. This function should have the following signature: procedure makeDouble( var i : int16; var j : int16; var k : int16 );@nodisplay; @noframe; After calling this function, the value of all the driver’s variables should be changed to twice the largest of the three passed parameters. Your function should replicate the following C code: void makeDouble( int * i, int * j, int * k ) { int most = *i; if (*j > most) { most = *j; } if (*k > most) { most = *k; } *i = most + most; *j = most + most; *k = most + most; } IN ORDER TO RECEIVE FULL CREDIT, YOU MUST USE THE TEMPLATE SOLUTION SUPPLIED BELOW. Of course, you will need to add code to the function to implement the desired algorithm explained above. In addition, you will need to push the parameters to the function. Be sure your function preserves all the registers it touches. // Reference Parameter Template Solution For CS 17 Final // CS 17 Students must use this template as the basis for their solution. // Please look at the two TODO: notes below program ReferenceProgram; #include( "stdlib.hhf" ); static iValue1 : int16 := 0; iValue2 : int16 := 0; iValue3 : int16 := 0; // TODO: CS 17 Students add code below to implement this function // Several hints are supplied procedure makeDouble( var i : int16; var j : int16; var k : int16 );@nodisplay; @noframe; static dReturnAddress : dword; begin makeDouble; // entry sequence // preserve registers used pop( dReturnAddress ); // this is the return address // push back the return address push( dReturnAddress ); // preserve registers // TODO: implement function // restore the registers used ret(); end makeDouble; begin ReferenceProgram; stdout.put( "Gimme iValue1" ); stdin.get( iValue1 ); stdout.put( "Gimme iValue2" ); stdin.get( iValue2 ); stdout.put( "Gimme iValue3" ); stdin.get( iValue3 ); // TODO: push parameters to the function. // These parameters must be passed by-reference. call makeDouble; stdout.put( "after makeDouble!" ); stdout.newln(); stdout.put( "iValue1 = " ); stdout.put( iValue1 ); stdout.put( "iValue2 = " ); stdout.put( iValue2 ); stdout.put( "iValue3 = " ); stdout.put( iValue3 ); stdout.newln(); end ReferenceProgram;
Create an HLA function that forces a value into all three passed parameters.
This function should have the following signature:
procedure makeDouble( var i : int16; var j : int16; var k : int16 );@nodisplay; @noframe;
After calling this function, the value of all the driver’s variables should be changed to twice the largest of the three passed parameters. Your function should replicate the following C code:
void makeDouble( int * i, int * j, int * k ) {
int most = *i;
if (*j > most)
{
most = *j;
}
if (*k > most)
{
most = *k;
}
*i = most + most;
*j = most + most;
*k = most + most;
}
IN ORDER TO RECEIVE FULL CREDIT, YOU MUST USE THE TEMPLATE SOLUTION SUPPLIED BELOW. Of course, you will need to add code to the function to implement the desired
// Reference Parameter Template Solution For CS 17 Final
// CS 17 Students must use this template as the basis for their solution.
// Please look at the two TODO: notes below
program ReferenceProgram;
#include( "stdlib.hhf" );
static
iValue1 : int16 := 0;
iValue2 : int16 := 0;
iValue3 : int16 := 0;
// TODO: CS 17 Students add code below to implement this function
// Several hints are supplied
procedure makeDouble( var i : int16; var j : int16; var k : int16 );@nodisplay; @noframe;
static
dReturnAddress : dword;
begin makeDouble;
// entry sequence
// preserve registers used
pop( dReturnAddress );
// this is the return address
// push back the return address
push( dReturnAddress );
// preserve registers
// TODO: implement function
// restore the registers used
ret();
end makeDouble;
begin ReferenceProgram;
stdout.put( "Gimme iValue1" );
stdin.get( iValue1 );
stdout.put( "Gimme iValue2" );
stdin.get( iValue2 );
stdout.put( "Gimme iValue3" );
stdin.get( iValue3 );
// TODO: push parameters to the function.
// These parameters must be passed by-reference.
call makeDouble;
stdout.put( "after makeDouble!" );
stdout.newln();
stdout.put( "iValue1 = " );
stdout.put( iValue1 );
stdout.put( "iValue2 = " );
stdout.put( iValue2 );
stdout.put( "iValue3 = " );
stdout.put( iValue3 );
stdout.newln();
end ReferenceProgram;

Step by step
Solved in 2 steps

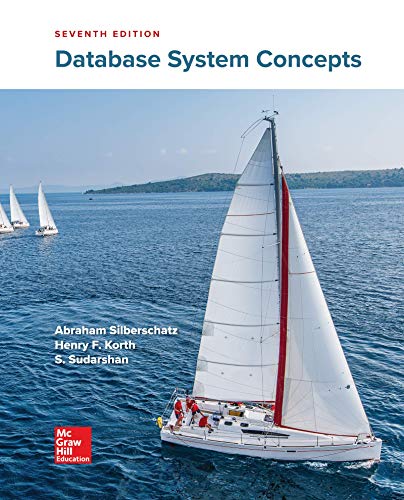
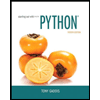
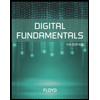
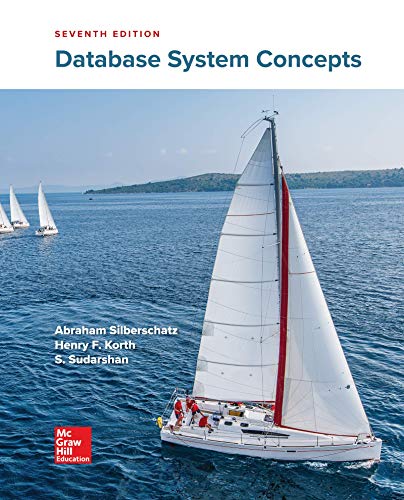
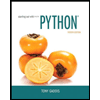
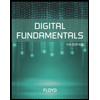
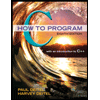
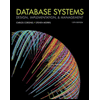
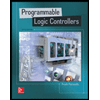