create a Word document that contains the pseudocode, flowchart, and test plan for (Programming Projects 2( import java.util.Scanner; public class Exercise07_03 { public static void main(String[] args) { Scanner input = new Scanner(System.in); int[] counts = new int[101]; // Array to store the occurrences // Read integers from user until 0 is entered System.out.print("Enter the integers between 1 and 100: "); int number = input.nextInt(); while (number != 0) { if (number >= 1 && number <= 100) { counts[number]++; // Increment the count for the number } number = input.nextInt(); } // Display the counts for each number for (int i = 1; i < counts.length; i++) { if (counts[i] > 0) { System.out.println(i + " occurs " + counts[i] + (counts[i] > 1 ? " times" : " time")); } } } }) and 5( import java.util.Scanner; public class Exercise07_19 { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter list: "); int size = input.nextInt(); int[] list = new int[size]; for (int i = 0; i < size; i++) { list[i] = input.nextInt(); } if (isSorted(list)) { System.out.println("The list is already sorted"); } else { System.out.println("The list is not sorted"); } } public static boolean isSorted(int[] list) { for (int i = 0; i < list.length - 1; i++) { if (list[i] > list[i + 1]) { return false; // Not sorted in increasing order } } return true; // Sorted in increasing order }
create a Word document that contains the pseudocode, flowchart, and test plan for (Programming Projects 2(
import java.util.Scanner;
public class Exercise07_03 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int[] counts = new int[101]; // Array to store the occurrences
// Read integers from user until 0 is entered
System.out.print("Enter the integers between 1 and 100: ");
int number = input.nextInt();
while (number != 0) {
if (number >= 1 && number <= 100) {
counts[number]++; // Increment the count for the number
}
number = input.nextInt();
}
// Display the counts for each number
for (int i = 1; i < counts.length; i++) {
if (counts[i] > 0) {
System.out.println(i + " occurs " + counts[i] + (counts[i] > 1 ? " times" : " time"));
}
}
}
})
and 5(
import java.util.Scanner;
public class Exercise07_19 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter list: ");
int size = input.nextInt();
int[] list = new int[size];
for (int i = 0; i < size; i++) {
list[i] = input.nextInt();
}
if (isSorted(list)) {
System.out.println("The list is already sorted");
} else {
System.out.println("The list is not sorted");
}
}
public static boolean isSorted(int[] list) {
for (int i = 0; i < list.length - 1; i++) {
if (list[i] > list[i + 1]) {
return false; // Not sorted in increasing order
}
}
return true; // Sorted in increasing order
}
})
from Chapter 7) that you completed in Revel.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

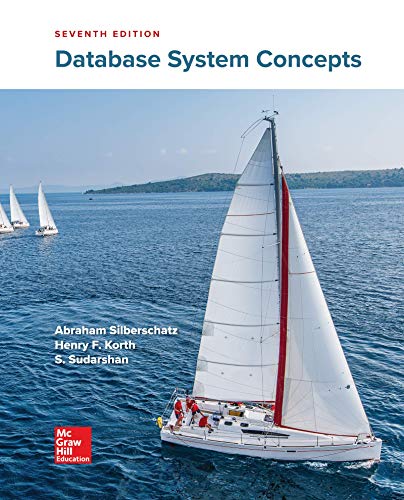
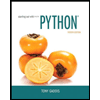
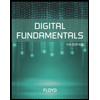
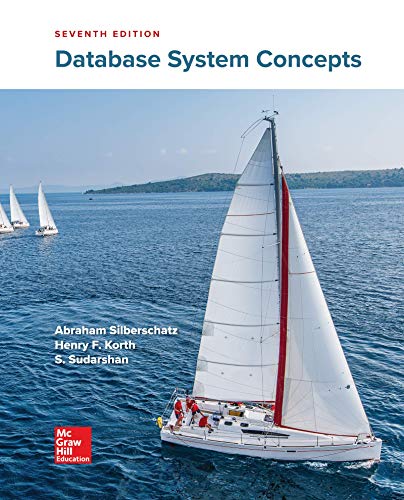
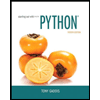
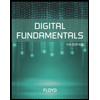
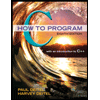
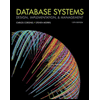
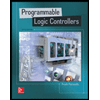