Create a python program that would perform the given description below Your task is to write a simple program which pretends to play tic-tac-toe with the user. To make it all easier for you, we've decided to simplify the game. Here are our assumptions: the computer (i.e., your program) should play the game using 'X's; the user (e.g., you) should play the game using 'O's; the first move belongs to the computer - it always puts its first 'X' in the middle of the board; all the squares are numbered row by row starting with 1 (see the example session below for reference) the user inputs their move by entering the number of the square they choose - the number must be valid, i.e., it must be an integer, it must be greater than 0 and less than 10, and it cannot point to a field which is already occupied; the program checks if the game is over - there are four possible verdicts: the game should continue, or the game ends with a tie, your win, or the computer's win; the computer responds with its move and the check is repeated; don't implement any form of artificial intelligence - a random field choice made by the computer is good enough for the game. The example session with the program may look as follows: +-------+-------+-------+ | | | | | 1 | 2 | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | 8 | 9 | | | | | +-------+-------+-------+ Enter your move: 1 +-------+-------+-------+ | | | | | O | 2 | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | 8 | 9 | | | | | +-------+-------+-------+ +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | 8 | 9 | | | | | +-------+-------+-------+ Enter your move: 8 +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | X | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ Enter your move: 4 +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ Enter your move: 7 +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | O | O | 9 | | | | | +-------+-------+-------+ You won! Requirements: Implement the following features: the board should be stored as a three-element list, while each element is another three-element list (the inner lists represent rows) so that all of the squares may be accessed using the following syntax: board[row][column] each of the inner list's elements can contain 'O', 'X', or a digit representing the square's number (such a square is considered free) the board's appearance should be exactly the same as the one presented in the example. Drawing a random integer number can be done by utilizing a Python function called randrange(). The example program below shows how to use it (the program prints ten random numbers from 0 to 8). Note: the from-import instruction provides an access to the randrange function defined within an external Python module called random. from random import randrange for i in range(10): print(randrange(8))
Create a python program that would perform the given description below Your task is to write a simple program which pretends to play tic-tac-toe with the user. To make it all easier for you, we've decided to simplify the game. Here are our assumptions: the computer (i.e., your program) should play the game using 'X's; the user (e.g., you) should play the game using 'O's; the first move belongs to the computer - it always puts its first 'X' in the middle of the board; all the squares are numbered row by row starting with 1 (see the example session below for reference) the user inputs their move by entering the number of the square they choose - the number must be valid, i.e., it must be an integer, it must be greater than 0 and less than 10, and it cannot point to a field which is already occupied; the program checks if the game is over - there are four possible verdicts: the game should continue, or the game ends with a tie, your win, or the computer's win; the computer responds with its move and the check is repeated; don't implement any form of artificial intelligence - a random field choice made by the computer is good enough for the game. The example session with the program may look as follows: +-------+-------+-------+ | | | | | 1 | 2 | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | 8 | 9 | | | | | +-------+-------+-------+ Enter your move: 1 +-------+-------+-------+ | | | | | O | 2 | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | 8 | 9 | | | | | +-------+-------+-------+ +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | 8 | 9 | | | | | +-------+-------+-------+ Enter your move: 8 +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | 6 | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | 4 | X | X | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ Enter your move: 4 +-------+-------+-------+ | | | | | O | X | 3 | | | | | +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | 7 | O | 9 | | | | | +-------+-------+-------+ Enter your move: 7 +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | O | X | X | | | | | +-------+-------+-------+ | | | | | O | O | 9 | | | | | +-------+-------+-------+ You won! Requirements: Implement the following features: the board should be stored as a three-element list, while each element is another three-element list (the inner lists represent rows) so that all of the squares may be accessed using the following syntax: board[row][column] each of the inner list's elements can contain 'O', 'X', or a digit representing the square's number (such a square is considered free) the board's appearance should be exactly the same as the one presented in the example. Drawing a random integer number can be done by utilizing a Python function called randrange(). The example program below shows how to use it (the program prints ten random numbers from 0 to 8). Note: the from-import instruction provides an access to the randrange function defined within an external Python module called random. from random import randrange for i in range(10): print(randrange(8))
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Create a python program that would perform the given description below
Your task is to write a simple program which pretends to play tic-tac-toe with the user. To make it all easier for you, we've decided to simplify the game. Here are our assumptions:
the computer (i.e., your program) should play the game using 'X's;
the user (e.g., you) should play the game using 'O's;
the first move belongs to the computer - it always puts its first 'X' in the middle of the board;
all the squares are numbered row by row starting with 1 (see the example session below for reference)
the user inputs their move by entering the number of the square they choose - the number must be valid, i.e., it must be an integer, it must be greater than 0 and less than 10, and it cannot point to a field which is already occupied;
the program checks if the game is over - there are four possible verdicts: the game should continue, or the game ends with a tie, your win, or the computer's win;
the computer responds with its move and the check is repeated;
don't implement any form of artificial intelligence - a random field choice made by the computer is good enough for the game.
The example session with the program may look as follows:
+-------+-------+-------+
| | | |
| 1 | 2 | 3 |
| | | |
+-------+-------+-------+
| | | |
| 4 | X | 6 |
| | | |
+-------+-------+-------+
| | | |
| 7 | 8 | 9 |
| | | |
+-------+-------+-------+
Enter your move: 1
+-------+-------+-------+
| | | |
| O | 2 | 3 |
| | | |
+-------+-------+-------+
| | | |
| 4 | X | 6 |
| | | |
+-------+-------+-------+
| | | |
| 7 | 8 | 9 |
| | | |
+-------+-------+-------+
+-------+-------+-------+
| | | |
| O | X | 3 |
| | | |
+-------+-------+-------+
| | | |
| 4 | X | 6 |
| | | |
+-------+-------+-------+
| | | |
| 7 | 8 | 9 |
| | | |
+-------+-------+-------+
Enter your move: 8
+-------+-------+-------+
| | | |
| O | X | 3 |
| | | |
+-------+-------+-------+
| | | |
| 4 | X | 6 |
| | | |
+-------+-------+-------+
| | | |
| 7 | O | 9 |
| | | |
+-------+-------+-------+
+-------+-------+-------+
| | | |
| O | X | 3 |
| | | |
+-------+-------+-------+
| | | |
| 4 | X | X |
| | | |
+-------+-------+-------+
| | | |
| 7 | O | 9 |
| | | |
+-------+-------+-------+
Enter your move: 4
+-------+-------+-------+
| | | |
| O | X | 3 |
| | | |
+-------+-------+-------+
| | | |
| O | X | X |
| | | |
+-------+-------+-------+
| | | |
| 7 | O | 9 |
| | | |
+-------+-------+-------+
+-------+-------+-------+
| | | |
| O | X | X |
| | | |
+-------+-------+-------+
| | | |
| O | X | X |
| | | |
+-------+-------+-------+
| | | |
| 7 | O | 9 |
| | | |
+-------+-------+-------+
Enter your move: 7
+-------+-------+-------+
| | | |
| O | X | X |
| | | |
+-------+-------+-------+
| | | |
| O | X | X |
| | | |
+-------+-------+-------+
| | | |
| O | O | 9 |
| | | |
+-------+-------+-------+
You won!
Requirements:
Implement the following features:
the board should be stored as a three-element list, while each element is another three-element list (the inner lists represent rows) so that all of the squares may be accessed using the following syntax:
board[row][column]
each of the inner list's elements can contain 'O', 'X', or a digit representing the square's number (such a square is considered free)
the board's appearance should be exactly the same as the one presented in the example.
Drawing a random integer number can be done by utilizing a Python function called randrange(). The example program below shows how to use it (the program prints ten random numbers from 0 to 8).
Note: the from-import instruction provides an access to the randrange function defined within an external Python module called random.
from random import randrange
for i in range(10):
print(randrange(8))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
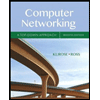
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
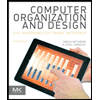
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
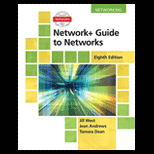
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
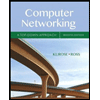
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
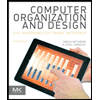
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
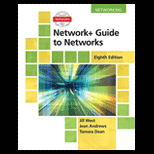
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
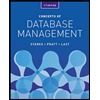
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
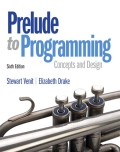
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
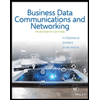
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY