Create a program in C++ that will (hypothetically) be a software component of an electronic coin counting machine that counts American coins. Valid coin denominations are the integers 1, 5, 10, 25, and 50. The program shall count the number of coins (by denomination) that are read from a coin file that is submitted to the program by way of standard input (which by default is the keyboard). It shall then produce a formatted coin report printed on standard output (which by default is the computer screen). The report shall contain information that follows the wording and format shown in the example-program-execution.txt file. Write the program as though you were reading from the keyboard. When you actually run the program, it will be run from the command line using a command line window. Command-line-redirection allows you to specify the name of a file to use as input. The contents of that file will be sent to the program as though those values were being typed on the keyboard, which is why this program will use cin and not file streams. Use only cin for input and cout for output. You will run your program from the command line and specify command-line redirection for the input, like this: smith12.exe
Create a program in C++ that will (hypothetically) be a software component of an electronic coin counting machine that counts American coins. Valid coin denominations are the integers 1, 5, 10, 25, and 50. The program shall count the number of coins (by denomination) that are read from a coin file that is submitted to the program by way of standard input (which by default is the keyboard). It shall then produce a formatted coin report printed on standard output (which by default is the computer screen). The report shall contain information that follows the wording and format shown in the example-program-execution.txt file.
Write the program as though you were reading from the keyboard. When you actually run the program, it will be run from the command line using a command line window. Command-line-redirection allows you to specify the name of a file to use as input. The contents of that file will be sent to the program as though those values were being typed on the keyboard, which is why this program will use cin and not file streams.
Use only cin for input and cout for output. You will run your program from the command line and specify command-line redirection for the input, like this:
smith12.exe <big-coin-file.txt
Be sure that the executable and the big-coin-file.txt file are in the same folder.
Format of a Coin File
The input data in a coin file shall consist of zero or more coin values, separated by spaces, tabs, or return characters, and terminated by a single mandatory 999 sentinel value that marks the end of the list of coins. The program may assume that all coin values are positive one-to-two-digit integers in the range from 1 to 99 inclusive. If a non-denominational number in that range (such as 40 or 71) is read from the input file, then that coin shall be counted as an invalid coin. (The 999 sentinel value shall neither be counted as an invalid coin, nor as any coin at all.)
Software Design
int main(void)
Declare integer variables to individually store the penny count, nickel count, dime count, quarter count, half dollar count, and invalid coin count. When doing so, set each to the value of zero. Then call the countCoins function, passing the six required parameters that it expects. Then call the produceCoinReport function, passing the six required parameters that it expects.
void countCoins(int &pennyCount, int &nickelCount, int &dimeCount, int &quarterCount, int &halfDollarCount, int &invalidCount);
Declare an integer variable to store the value of a coin. Then read a coin value from standard input using cin and store it in the coin variable. After doing that, start a "while" loop that loops as long as the coin value does not equal the SENTINEL, which marks the end of the data.
Inside the "while" loop, set up an "else if" control structure that counts the coins. It should do this by comparing the current coin value to 1, 5, 10, 25, and 50. As soon as a comparison is true, the program should increment the count for that coin and move on to reading the next coin. If no coin match is found, then the program should count the coin as invalid. The basic design of the "else if" control structure should look something like this:
if the coin is a penny
increment the penny count
else if the coin is a nickel
increment the nickel count
else if ...
...
else
increment the invalid coin count
When the "else if" control structure is finished, read the next coin value from standard input and store it in the coin variable. (This read statement should be INSIDE the while loop.)
void produceCoinReport(int pennyCount, int nickelCount, int dimeCount, int quarterCount, int halfDollarCount, int invalidCount);
Declare an integer variable to store the total coin count and a floating point variable to store the grand total monetary value of the coins. Add up all the coin counts and store the total. Use the coin count of each coin along with the monetary value of each coin to calculate the total monetary value of all the coins. Store that answer. Print the coin report in the format shown in the example-program-execution file. Set the precision to 2 before printing the floating point numbers in the report.
Files Referenced
example-program-execution(12).txt (attached as photo. You will need to convert to a txt file)
big-coin-file.txt (For testing the program; numbers shown below, convert to a txt file)
1
5
10
25
50
17
50
50
50
34
50
67
25
50
25
25
25
5
10
5
5
10
10
10
1
1
1
1
1
56
78
89
25
25
10
10
10
1
1
1
1
5
5
5
5
90
23
44
56
67
89
1
5
10
25
50
17
50
50
34
50
67
25
25
25
25
5
10
5
5
10
10
10
1
1
1
1
1
56
78
89
10
10
10
10
10
25
25
10
10
10
1
1
1
1
5
5
5
5
90
23
44
56
67
89
1
5
10
25
50
17
50
50
50
34
50
67
25
50
25
25
25
5
10
5
5
10
10
10
1
1
1
1
1
56
78
89
50
50
25
25
10
10
10
1
1
1
1
5
5
5
5
90
23
44
56
67
89
1
5
10
25
50
17
50
50
50
34
50
67
25
50
25
25
25
5
10
5
5
10
10
10
1
1
1
1
1
5
5
5
5
5
56
78
89
50
50
25
25
10
10
10
1
1
1
1
5
5
5
5
10
10
10
10
10
10
10
10
90
23
44
56
67
89
999


Step by step
Solved in 4 steps with 1 images

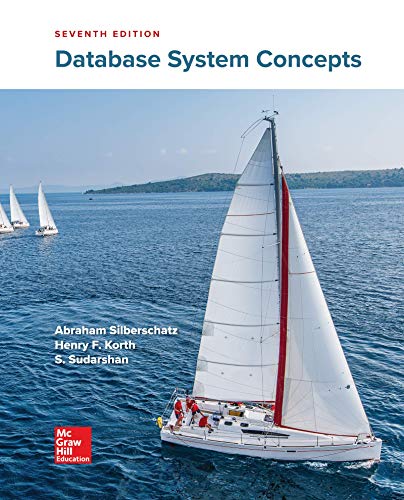
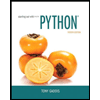
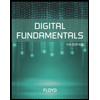
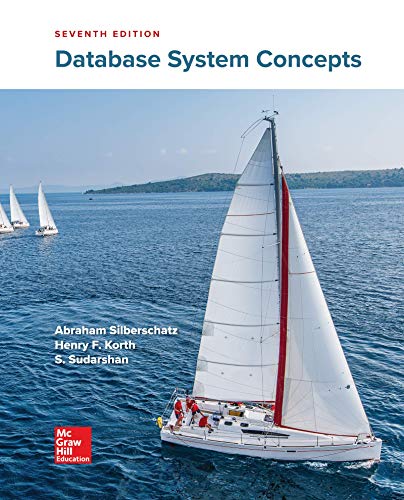
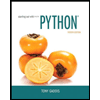
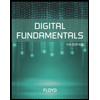
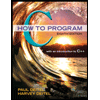
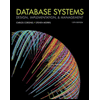
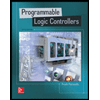