Create a program in C++ that provides a solution to the mathematical puzzle described in the number-wheel-puzzle.jpg file. To solve the number wheel puzzle, a person needs to "place numbers 1 through 11 in the circles so that any three circles in a straight line make the same sum." Basic algorithm that your program shall follow: Declare an array of eleven elements to represent the number wheel. Each index in the array will denote a specific circle in the wheel as shown in the number-wheel-puzzle.jpg file. The circles are numbered clockwise beginning at the top and starting with index zero. The center circle is given index 10 Fill array with the numbers 1 through 11 by putting 1 in the location for index 0, 2 in the location for index 1, etc. Check the current contents of the wheel to see if the sums match up for each straight line through the center of the wheel. If they don't, then randomly reorder the numbers in each of the eleven circles and check the sums again. Do this until a correct solution is found. Display the solution (i.e., the values) contained in the eleven circles The program shall have no interaction with a user except for starting the program. The output of the program shall follow the wording and format shown in the example-program-execution.txt file. Note that this file shows one solution to the puzzle. Actually, there are only three correct solutions, but because of the layout of the puzzle, thousands of permutations exist for those three solutions. Implement the design described below: // You will need to set these Global Constants const int MAX_CIRCLES = 11; const int CENTER_CIRCLE_INDEX = 10; int main(void) srand(time(NULL)); Declare an array for the wheel that holds MAX_CIRCLES integers Declare an integer variable for storing the number of interactions when searching for a solution, and initialize it to zero Call the fillTheWheel function, passing it the wheel array Set up a while loop that has (!matchingSums(wheel)) as its conditional expression, which calls the matchingSums function and negates the returned result. Inside the while loop, first increment the iteration counter by one. Next, call the randomizeTheContents function, passing it the MAX_CIRCLES constant and the wheel array After the while loop in finished, print a line containing the message "After 1000 unsuccessful attempts, the following solution was found:" as shown in the example-program-execution.txt file. In the message, replace "1000" with the value stored in the iteration counter Call the displayWheelContents function, passing it the wheel array void fillTheWheel(int wheel[]) Use a for loop to store the values 1 through (MAX_CIRCLES - 1), respectively, at the indexes 0 through 10 in the wheel array. void displayWheelContents(int wheel[]) Display a line containg the message "* Outside circles (clockwise from the top):" Print a blank line Use a for loop to print the contents of indexes 0 through (MAX_CIRCLES - 1) in the wheel array. Print each value in a column width of 4 as shown in the example-program-execution.txt file Print two blank lines Display the message "* Center circle: " followed by the value stored in the CENTER_CIRCLE_INDEX of the wheel array void randomizeTheContents(int nbrOfItems, int table[]) The design of this function is generalized so that it can be used to randomize the contents of any length of integer array, not just the wheel array. That is why two parameters are passed into the function. The function uses a random number generator function (rand()) along with a swap algorithm to randomly relocate the elements in the array. The swap routine uses three integer variables: temp, indexA, and indexB. Declare the temp, indexA and indexB integer variables Use a for loop that sets indexA to zero and increments indexA after each iteration. The for loop should continue iterating as long as indexA is less than the number of items in the array (not less than or equal, which will cause a one-off error). Do the following (in this order) inside the for loop: indexB = rand() % nbrOfItems temp = table[indexA] table[indexA] = table[indexB] table[indexB] = temp bool matchingSums(int wheel[]) Copy and paste this exactly as it is: bool matchingSums(int wheel[]) { const int MAX_OUTER_CIRCLES = MAX_CIRCLES - 1; const int OPPOSITE_SIDE_FACTOR = 5; const int STARTING_INDEX = 0; int firstSum; int nextSum; // Calculate the sum of the first pair of numbers firstSum = wheel[STARTING_INDEX] + wheel[CENTER_CIRCLE_INDEX] + wheel[STARTING_INDEX + OPPOSITE_SIDE_FACTOR]; // Compare the first sum to each of the sums of the other pairs for (int i = 1; i < MAX_OUTER_CIRCLES/2; i++) { nextSum = wheel[i] + wheel[CENTER_CIRCLE_INDEX] + wheel[i + OPPOSITE_SIDE_FACTOR]; if (firstSum != nextSum) return false; } // End for return true; } // End matchingSums Referenced Files number-wheel-puzzle.jpg (see attached file example-program-execution(2).txt (see screenshot)
Create a
Basic
Declare an array of eleven elements to represent the number wheel. Each index in the array will denote a specific circle in the wheel as shown in the number-wheel-puzzle.jpg file. The circles are numbered clockwise beginning at the top and starting with index zero. The center circle is given index 10
Fill array with the numbers 1 through 11 by putting 1 in the location for index 0, 2 in the location for index 1, etc.
Check the current contents of the wheel to see if the sums match up for each straight line through the center of the wheel. If they don't, then randomly reorder the numbers in each of the eleven circles and check the sums again. Do this until a correct solution is found.
Display the solution (i.e., the values) contained in the eleven circles
The program shall have no interaction with a user except for starting the program. The output of the program shall follow the wording and format shown in the example-program-execution.txt file. Note that this file shows one solution to the puzzle. Actually, there are only three correct solutions, but because of the layout of the puzzle, thousands of permutations exist for those three solutions.
Implement the design described below:
// You will need to set these Global Constants
const int MAX_CIRCLES = 11;
const int CENTER_CIRCLE_INDEX = 10;
int main(void)
srand(time(NULL));
Declare an array for the wheel that holds MAX_CIRCLES integers
Declare an integer variable for storing the number of interactions when searching for a solution, and initialize it to zero
Call the fillTheWheel function, passing it the wheel array
Set up a while loop that has (!matchingSums(wheel)) as its conditional expression, which calls the matchingSums function and negates the returned result. Inside the while loop, first increment the iteration counter by one. Next, call the randomizeTheContents function, passing it the MAX_CIRCLES constant and the wheel array
After the while loop in finished, print a line containing the message "After 1000 unsuccessful attempts, the following solution was found:" as shown in the example-program-execution.txt file. In the message, replace "1000" with the value stored in the iteration counter
Call the displayWheelContents function, passing it the wheel array
void fillTheWheel(int wheel[])
Use a for loop to store the values 1 through (MAX_CIRCLES - 1), respectively, at the indexes 0 through 10 in the wheel array.
void displayWheelContents(int wheel[])
Display a line containg the message "* Outside circles (clockwise from the top):"
Print a blank line
Use a for loop to print the contents of indexes 0 through (MAX_CIRCLES - 1) in the wheel array. Print each value in a column width of 4 as shown in the example-program-execution.txt file
Print two blank lines
Display the message "* Center circle: " followed by the value stored in the CENTER_CIRCLE_INDEX of the wheel array
void randomizeTheContents(int nbrOfItems, int table[])
The design of this function is generalized so that it can be used to randomize the contents of any length of integer array, not just the wheel array. That is why two parameters are passed into the function. The function uses a random number generator function (rand()) along with a swap algorithm to randomly relocate the elements in the array. The swap routine uses three integer variables: temp, indexA, and indexB.
Declare the temp, indexA and indexB integer variables
Use a for loop that sets indexA to zero and increments indexA after each iteration. The for loop should continue iterating as long as indexA is less than the number of items in the array (not less than or equal, which will cause a one-off error). Do the following (in this order) inside the for loop:
indexB = rand() % nbrOfItems
temp = table[indexA]
table[indexA] = table[indexB]
table[indexB] = temp
bool matchingSums(int wheel[])
Copy and paste this exactly as it is:
bool matchingSums(int wheel[])
{
const int MAX_OUTER_CIRCLES = MAX_CIRCLES - 1;
const int OPPOSITE_SIDE_FACTOR = 5;
const int STARTING_INDEX = 0;
int firstSum;
int nextSum;
// Calculate the sum of the first pair of numbers
firstSum = wheel[STARTING_INDEX] + wheel[CENTER_CIRCLE_INDEX]
+ wheel[STARTING_INDEX + OPPOSITE_SIDE_FACTOR];
// Compare the first sum to each of the sums of the other pairs
for (int i = 1; i < MAX_OUTER_CIRCLES/2; i++)
{
nextSum = wheel[i] + wheel[CENTER_CIRCLE_INDEX] + wheel[i + OPPOSITE_SIDE_FACTOR];
if (firstSum != nextSum)
return false;
} // End for
return true;
} // End matchingSums
Referenced Files
number-wheel-puzzle.jpg (see attached file
example-program-execution(2).txt (see screenshot)



Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

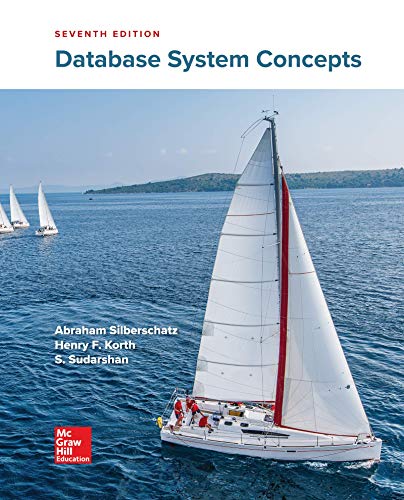
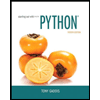
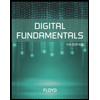
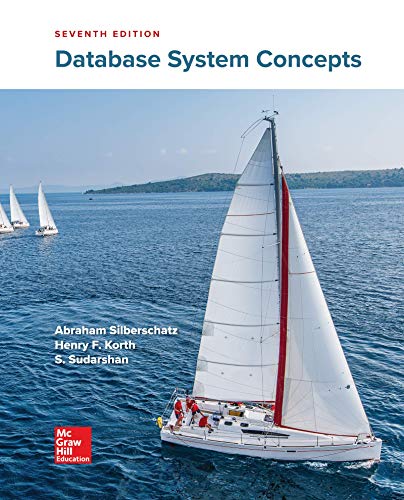
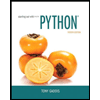
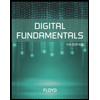
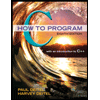
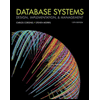
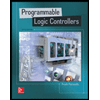