Create a main() function, and place lines 23-38 in it. Then add a call to main() at the end of the file. Summary The program will design a new version of the program I created for Part 2 of Assignment 02. This program will create a function for the formula used in that program with the appropriate parameters, and return value. ''' def CalculateSpeed(total_miles_driven,time_driven): speed = float(total_miles_driven) / float(time_driven) return float(speed) def CalculateMPG(total_miles_driven,gallon_gas): MPG = float(total_miles_driven) / float(gallon_gas) return MPG #main part of code LINE 23 total_miles_driven = float(input("Enter the no of miles driven: ")) LINE 24time_driven = float(input("Enter how many hours did it driven: ")) LINE 25 gallon_gas = float(input("Enter the gallons of gas used: ")) LINE 26 LINE 27 #calling function calculating MPG LINE 28 MPG=CalculateMPG(total_miles_driven,gallon_gas) #print the MPG value print("The milesPerGallon of the car is {MPG}".format(MPG=round(MPG, 2))) #calling function- calculate speed and display the result print("The speed you were traveling is:",CalculateSpeed(total_miles_driven,time_driven))
Create a main() function, and place lines 23-38 in it. Then add a call to main() at the end of the file.
Summary
The program will design a new version of the program I created for Part 2 of Assignment 02.
This program will create a function for the formula used in that program
with the appropriate parameters, and return value.
'''
def CalculateSpeed(total_miles_driven,time_driven):
speed = float(total_miles_driven) / float(time_driven)
return float(speed)
def CalculateMPG(total_miles_driven,gallon_gas):
MPG = float(total_miles_driven) / float(gallon_gas)
return MPG
#main part of code
LINE 23 total_miles_driven = float(input("Enter the no of miles driven: "))
LINE 24time_driven = float(input("Enter how many hours did it driven: "))
LINE 25 gallon_gas = float(input("Enter the gallons of gas used: "))
LINE 26
LINE 27 #calling function calculating MPG
LINE 28
MPG=CalculateMPG(total_miles_driven,gallon_gas)
#print the MPG value
print("The milesPerGallon of the car is {MPG}".format(MPG=round(MPG, 2)))
#calling function- calculate speed and display the result
print("The speed you were traveling is:",CalculateSpeed(total_miles_driven,time_driven))

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

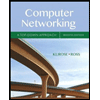
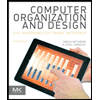
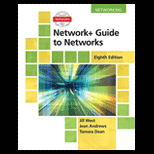
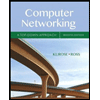
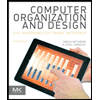
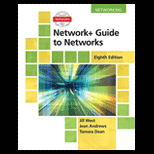
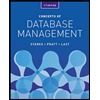
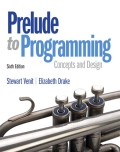
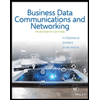