1. Create the functions sadness_score, and happiness_score 2. Each function has only 1 parameter of type string: text 3. In each function, create a list of tuples for the words and their weights i.e in happiness_score you should have happiness_list = [ ("accomplish, 3), ....]
1. Create the functions sadness_score, and happiness_score 2. Each function has only 1 parameter of type string: text 3. In each function, create a list of tuples for the words and their weights i.e in happiness_score you should have happiness_list = [ ("accomplish, 3), ....]
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In python !
"""
You can create the storage for "happy" words as following:
sadness_list = [ ("abandon", 2), ("ashamed", 4), ....]
We advise you to do that inside the function where you would use it.
You should have 2 lists, sadness_list and happiness_list each inside the corresponding function
"""
''' Define function sadness_score '''
''' Define function happiness_score '''

Transcribed Image Text:**Learning Objectives**
1. Create a function in a modular structure.
2. Use Lists of tuples to calculate weights.
3. Loop over the input string and the list of words.
4. Print analysis of the given input.
**Instructions**
A very known application in natural language processing is to extract information from a given text. In this lab, you will be able to extract some emotions (Sad and Happy) from a given string and calculate the score of each emotion. You will be given below the words that express sadness, anger, and happiness. For each word, you will be given a weight as well, so that when you are calculating, for example, the score for sadness in the text (string), you can use this formula:
```
Sadness_Score = Weight_1 + Weight_3 + Weight_4 + ......
```
**Sadness Words**
| Word | Weight | Word | Weight | Word | Weight | Word | Weight |
|-----------|--------|----------|--------|--------|--------|--------|--------|
| abandon | 2 | ashamed | 4 | awful | 4 | bad | 5 |
| hard | 3 | belittle | 3 | betray | 5 | crash | 4 |
| crazy | 2 | cry | 3 | disappoint | 3 | death | 5 |
**Happiness Words**
| Word | Weight | Word | Weight | Word | Weight | Word | Weight |
|-----------|--------|----------|--------|-----------|--------|-----------|--------|
| accomplish| 3 | friend | 3 | beach | 4 | beautiful | 5 |
| dawn | 4 | adorable | 3 | enjoy | 5 | celebrate | 4 |
| cheer | 3 | bless | 4 | baby | 3 | angel | 5 |
1. Create the functions `sadness_score` and `happiness_score`.
2. Each function has only 1 parameter of type string: `text`.
3. In each function, create a list of tuples for the words and their weights, i.e., in `happiness_score` you should have `happiness_list = [ ("accomplish",
![1. Create the functions `sadness_score` and `happiness_score`.
2. Each function has only 1 parameter of type string: `text`.
3. In each function, create a list of tuples for the words and their weights, i.e., in `happiness_score` you should have `happiness_list = [ ("accomplish", 3), ... ]`.
4. Loop over the tuples and check if the word is in the given `text`. If the word is found, increment the result by the word's score; otherwise, do nothing.
5. Return the final score.
**Example**
Input: "This lab is driving me crazy. I want to accomplish more so I won't feel bad at the end of the day"
Return from `sadness_score` function: 7
Return from `happiness_score` function: 3
**Hint!**
- Do not calculate the number of words in the text. The sentence "I am so bad" and "I am so bad bad bad" should return the same score for this lab, though in the real world it is not always so.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffe70859f-d1ee-4d19-93f5-d3c21b44393e%2F8446940e-54a4-4abb-b8b2-4068176c168a%2Fsb2pf93_processed.png&w=3840&q=75)
Transcribed Image Text:1. Create the functions `sadness_score` and `happiness_score`.
2. Each function has only 1 parameter of type string: `text`.
3. In each function, create a list of tuples for the words and their weights, i.e., in `happiness_score` you should have `happiness_list = [ ("accomplish", 3), ... ]`.
4. Loop over the tuples and check if the word is in the given `text`. If the word is found, increment the result by the word's score; otherwise, do nothing.
5. Return the final score.
**Example**
Input: "This lab is driving me crazy. I want to accomplish more so I won't feel bad at the end of the day"
Return from `sadness_score` function: 7
Return from `happiness_score` function: 3
**Hint!**
- Do not calculate the number of words in the text. The sentence "I am so bad" and "I am so bad bad bad" should return the same score for this lab, though in the real world it is not always so.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
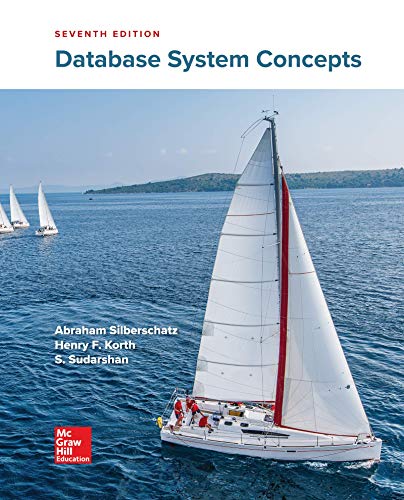
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
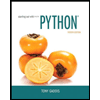
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
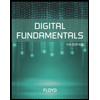
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
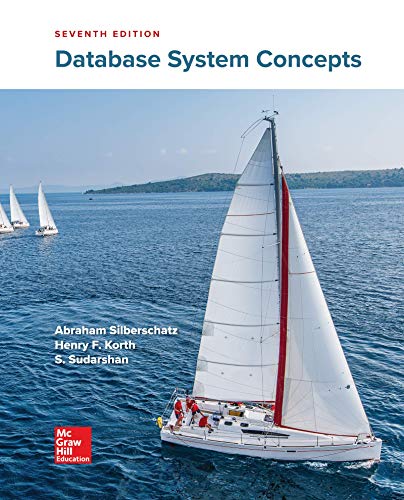
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
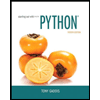
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
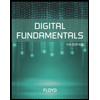
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
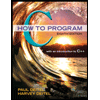
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
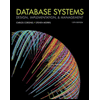
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
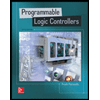
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education