Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having value “Math, 99.9” and “Physics, 98.9” respectively. Display the values of both objects Change the value of object from 99.9 to 89.9 Compare both objects and display the values of the subject which have lowest score.
Create a class “Main” having main method to perform following tasks.
- Create two objects of Subject class having value “Math, 99.9” and “Physics, 98.9” respectively.
- Display the values of both objects
- Change the value of object from 99.9 to 89.9
- Compare both objects and display the values of the subject which have lowest score.
code:-
//Java program
package subject;
//class definition
public class Subject {
//instance variable
String subject;
double score;
//method to set the name and score
public void setSubject(String n1,double n2){
subject=n1;
score=n2;
if((n2<0.0) || (n2>100.0)){
System.out.println("Incorrect score");
score=0.0;
}
}
//method to set the score
public void setScore(double n){
score=n;
if((n<0.0) || (n>100.0)){
System.out.println("Incorrect score");
score=0.0;
}
}
//method to print the result
public void display(){
System.out.println("Name: "+subject+", Score: "+score);
}
//method to get the score
public double getScore(){
return score;
}
//method to compare teh score and retun true if the calling object has greater score
public boolean greaterThan(Subject obj){
return obj.score<score;
}
//driver method
public static void main(String args[]){
// Object of Student class is created
Subject obj = new Subject();
obj.setSubject("Math",99.9);
obj.display();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

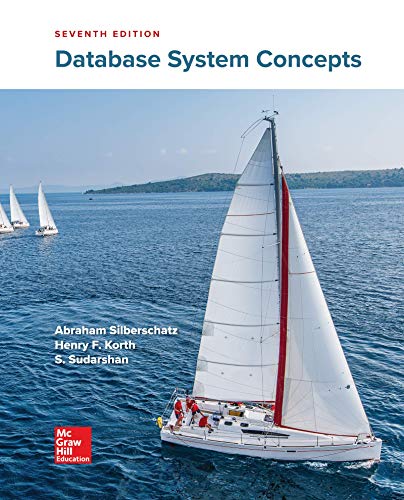
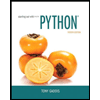
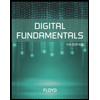
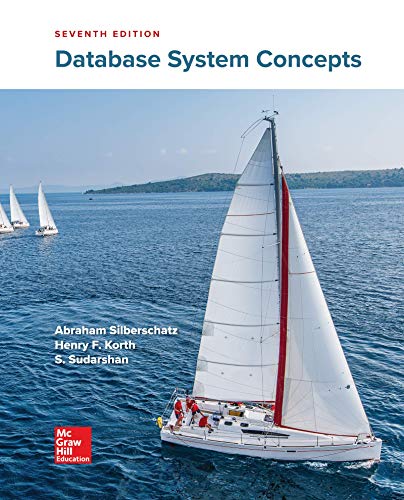
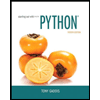
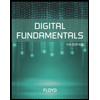
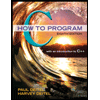
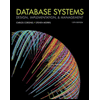
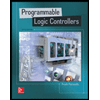