CPP File #include "result.h" #include "Date.h" Result::Result() { m_name = ""; m_unitID = ""; m_credits = 0; m_mark = 0.0; m_day = 0; m_month = ""; m_year = 0; } Result::Result( string name, string id,unsigned credits, double M , unsigned d, string m, unsigned y) { m_name = name; m_unitID = id; m_credits = credits; m_mark = M; m_day = d; m_month = m; m_year = y; } istream & operator >>( istream & input, Result & RE) { string strInput; getline(input,strInput, ','); RE.SetUnitID(strInput); getline(input, strInput, ','); RE.SetName(strInput); getline(input, strInput, ','); RE.SetCredits(stoi(strInput)); getline(input, strInput, ','); RE.SetMark(stod(strInput)); getline(input,strInput, ','); RE.SetDay(stoi(strInput)); getline(input, strInput, ','); RE.SetMonth(strInput); getline(input, strInput, ','); RE.SetYear(stoi(strInput)); getline(input, strInput, ','); return input; } ostream & operator <<( ostream & os,const Result & RE ) { string unitID; string name; string month; double mark; RE.GetUnitID(unitID); RE.GetName(name); RE.GetMonth(month); RE.GetMark(mark); os << " Unit ID: " << unitID << '\n' << " Unit Name: " << name << '\n' << " Credits: " << RE.GetCredits() << '\n' << " Mark: " << mark << '\n' << " Date: " << RE.GetDay() << " " << month << " " << RE.GetYear() << '\n'; return os; } Header File #ifndef RESULT_H #define RESULT_H #include #include using namespace std; const unsigned UnitNameSize = 40; class Result { public: Result(); Result( std::string (nam), string id, unsigned credits , double M, unsigned d, string m, unsigned y); // Construct a course from a name, section letter, // and number of credits. void GetName (string &name) const; void SetName(const string &name); void GetUnitID(string &id) const; void SetUnitID(const string &id); unsigned GetCredits() const; void SetCredits(unsigned credits); double GetMark() const; void SetMark(double M); unsigned GetDay() const; void SetDay(unsigned d); void GetMonth(string &m) const; void SetMonth(string &m); unsigned GetYear() const; void SetYear(unsigned y); // These operators have been made friends. They have // privileged access to class internals. // Very useful for debugging the class, but not very good for class design. // We will keep using it for now but you will have a chance in a later lab // to redesign this class. friend ostream & operator <<( ostream & os, const Result & RE ); friend istream & operator >>( istream & input, Result & RE ); private: string m_name; // course name, C style string. not a C++ string object string m_unitID; // section (letter) can be enrolment mode int m_credits; // number of credits double m_mark; // marks int m_day; // day of the date string m_month; //month of the date int m_year; // year of the date }; void Result::GetName (string &name) const { name = m_name; } void Result::SetName(const string &name) { m_name = name; } void Result::GetUnitID(string &id) const { id = m_unitID; } void Result::SetUnitID(const string &id) { m_unitID = id; } unsigned Result::GetCredits() const { return m_credits; } void Result::SetCredits(unsigned credits) { m_credits = credits; } double Result::GetMark() const { return m_mark; } void Result::SetMark(double M) { m_mark = M; } unsigned Result::GetDay() const { return m_day; } void Result::SetDay(unsigned d) { m_day = d; } void Result::GetMonth(string &m) const { m = m_month; } void Result::SetMonth(string &m) { m_month = m; } unsigned Result::GetYear() const { return m_year; } void Result::SetYear(unsigned y) { m_year = y; } after implementing this code in an attempt to let the program read an input file with the method getline, I came across an error that says "error: no matching function for call to 'Result: : GetMark(double&) const'" I need the body of the methods to be in the CPP file and only the method and class declaration to be in the .H file only
CPP File
#include "result.h"
#include "Date.h"
Result::Result()
{
m_name = "";
m_unitID = "";
m_credits = 0;
m_mark = 0.0;
m_day = 0;
m_month = "";
m_year = 0;
}
Result::Result( string name, string id,unsigned credits, double M , unsigned d, string m, unsigned y)
{
m_name = name;
m_unitID = id;
m_credits = credits;
m_mark = M;
m_day = d;
m_month = m;
m_year = y;
}
istream & operator >>( istream & input, Result & RE)
{
string strInput;
getline(input,strInput, ',');
RE.SetUnitID(strInput);
getline(input, strInput, ',');
RE.SetName(strInput);
getline(input, strInput, ',');
RE.SetCredits(stoi(strInput));
getline(input, strInput, ',');
RE.SetMark(stod(strInput));
getline(input,strInput, ',');
RE.SetDay(stoi(strInput));
getline(input, strInput, ',');
RE.SetMonth(strInput);
getline(input, strInput, ',');
RE.SetYear(stoi(strInput));
getline(input, strInput, ',');
return input;
}
ostream & operator <<( ostream & os,const Result & RE )
{
string unitID;
string name;
string month;
double mark;
RE.GetUnitID(unitID);
RE.GetName(name);
RE.GetMonth(month);
RE.GetMark(mark);
os << " Unit ID: " << unitID << '\n'
<< " Unit Name: " << name << '\n'
<< " Credits: " << RE.GetCredits() << '\n'
<< " Mark: " << mark << '\n'
<< " Date: " << RE.GetDay() << " " << month << " " << RE.GetYear() << '\n';
return os;
}
Header File
#ifndef RESULT_H
#define RESULT_H
#include <iostream>
#include <string>
using namespace std;
const unsigned UnitNameSize = 40;
class Result {
public:
Result();
Result( std::string (nam), string id, unsigned credits , double M, unsigned d, string m, unsigned y);
// Construct a course from a name, section letter,
// and number of credits.
void GetName (string &name) const;
void SetName(const string &name);
void GetUnitID(string &id) const;
void SetUnitID(const string &id);
unsigned GetCredits() const;
void SetCredits(unsigned credits);
double GetMark() const;
void SetMark(double M);
unsigned GetDay() const;
void SetDay(unsigned d);
void GetMonth(string &m) const;
void SetMonth(string &m);
unsigned GetYear() const;
void SetYear(unsigned y);
// These operators have been made friends. They have
// privileged access to class internals.
// Very useful for debugging the class, but not very good for class design.
// We will keep using it for now but you will have a chance in a later lab
// to redesign this class.
friend ostream & operator <<( ostream & os, const Result & RE );
friend istream & operator >>( istream & input, Result & RE );
private:
string m_name; // course name, C style string. not a C++ string object
string m_unitID; // section (letter) can be enrolment mode
int m_credits; // number of credits
double m_mark; // marks
int m_day; // day of the date
string m_month; //month of the date
int m_year; // year of the date
};
void Result::GetName (string &name) const
{
name = m_name;
}
void Result::SetName(const string &name)
{
m_name = name;
}
void Result::GetUnitID(string &id) const
{
id = m_unitID;
}
void Result::SetUnitID(const string &id)
{
m_unitID = id;
}
unsigned Result::GetCredits() const
{
return m_credits;
}
void Result::SetCredits(unsigned credits)
{
m_credits = credits;
}
double Result::GetMark() const
{
return m_mark;
}
void Result::SetMark(double M)
{
m_mark = M;
}
unsigned Result::GetDay() const
{
return m_day;
}
void Result::SetDay(unsigned d)
{
m_day = d;
}
void Result::GetMonth(string &m) const
{
m = m_month;
}
void Result::SetMonth(string &m)
{
m_month = m;
}
unsigned Result::GetYear() const
{
return m_year;
}
void Result::SetYear(unsigned y)
{
m_year = y;
}
after implementing this code in an attempt to let the program read an input file with the method getline, I came across an error that says "error: no matching function for call to 'Result: : GetMark(double&) const'"
I need the body of the methods to be in the CPP file and only the method and class declaration to be in the .H file only. i have multiple other files that I cant post here but it all works together.


Step by step
Solved in 2 steps

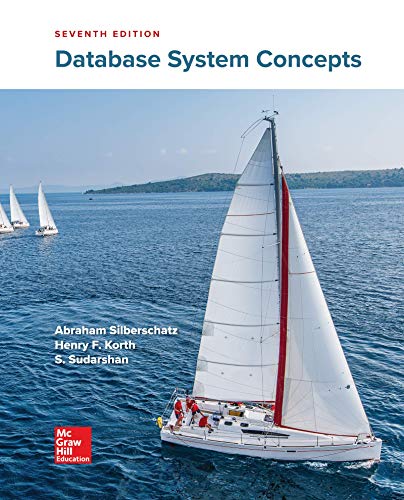
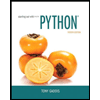
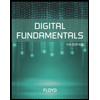
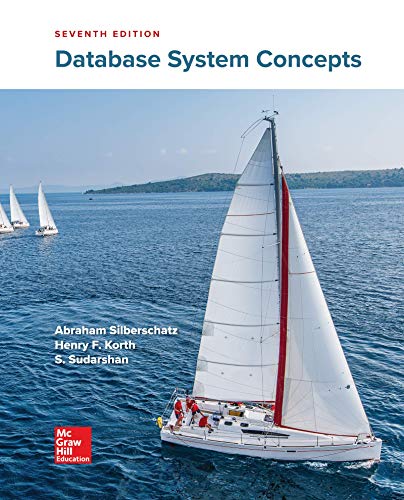
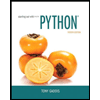
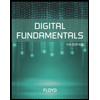
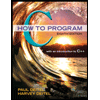
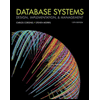
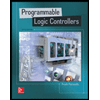