Food Functions - How do I do this practice exercise using Swift code?
Food Functions - How do I do this practice exercise using Swift code?
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Food Functions - How do I do this practice exercise using Swift code?
![App Exercise - Food Functions
Note
These exercises reinforce Swift concepts in the context of a fitness tracking app.
Suppose you want your fitness tracking app to give users the ability to log food. Once food has been logged, users should be able to go back and see what they ate at a specific meal.
Write a function that takes a String parameter where you will pass in either "Breakfast", "Lunch", or "Dinner". The function should then return the Meal object associated with that meal, or
return nil if the user hasn't logged that meal yet. Note that a Meal object and a dictionary, meals, representing the meal log have been created for you below. Call the function one or twice and
print the return value.
11
struct Meal {
12
var food: [String]
13
var calories: Int
14
}
15
16
var meals: [String: Meal] = ["Breakfast": Meal(food: ["Bagel", "Orange Juice", "Egg Whites"], calories: 530)]
17
18
iOS comes with a few different APIS for persistence, or saving data. You'll learn more about persistence in another lesson, but for now imagine what an app experience would be like if every time
you opened the app all of your data was gone. That would be frustrating, right?
Write a function that will check to see if your meal log (a dictionary like that in the previous exercise) is saved to the device. If it is, return the meal log. If it isn't, return an empty dictionary of
type [String: Any]. The code you should use in this exercise for retrieving something saved to the device is UserDefaults.standard.dictionary(forKey: "meallog").This code will
return an optional [String: Any]. If it returns a value, that is your meal log. If it returns nil, then no meal log has been saved. Call the function and print the return value.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbea0901b-9b71-43dc-90d5-d9e10ed3de89%2F86c97009-4657-472a-beda-95dd9da45cd3%2F7h5w41h_processed.png&w=3840&q=75)
Transcribed Image Text:App Exercise - Food Functions
Note
These exercises reinforce Swift concepts in the context of a fitness tracking app.
Suppose you want your fitness tracking app to give users the ability to log food. Once food has been logged, users should be able to go back and see what they ate at a specific meal.
Write a function that takes a String parameter where you will pass in either "Breakfast", "Lunch", or "Dinner". The function should then return the Meal object associated with that meal, or
return nil if the user hasn't logged that meal yet. Note that a Meal object and a dictionary, meals, representing the meal log have been created for you below. Call the function one or twice and
print the return value.
11
struct Meal {
12
var food: [String]
13
var calories: Int
14
}
15
16
var meals: [String: Meal] = ["Breakfast": Meal(food: ["Bagel", "Orange Juice", "Egg Whites"], calories: 530)]
17
18
iOS comes with a few different APIS for persistence, or saving data. You'll learn more about persistence in another lesson, but for now imagine what an app experience would be like if every time
you opened the app all of your data was gone. That would be frustrating, right?
Write a function that will check to see if your meal log (a dictionary like that in the previous exercise) is saved to the device. If it is, return the meal log. If it isn't, return an empty dictionary of
type [String: Any]. The code you should use in this exercise for retrieving something saved to the device is UserDefaults.standard.dictionary(forKey: "meallog").This code will
return an optional [String: Any]. If it returns a value, that is your meal log. If it returns nil, then no meal log has been saved. Call the function and print the return value.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
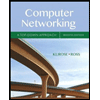
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
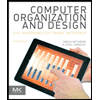
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
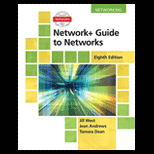
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
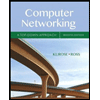
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
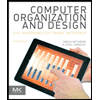
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
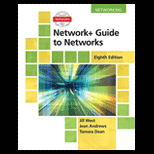
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
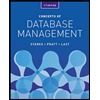
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
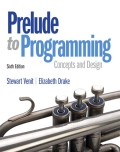
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
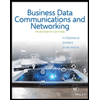
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY