Course:Data Structure and Algorithms Kindly do this correct as soon as possible: I am providing the a menu base programs in java for address book by using doubly LinkedList and arrayList in a menu which are following: 1.Enter 1 for using Doubly linkedList 2.Enter 2 for using arrayList YOU have to use File handling which create person.text file like this: Perform these steps while application is loading up. You can add a new method loadPersons. • Establish a data channel with a file by using streams • Start reading data (person records) from file line by line • Construct PersonInfo objects from each line you have read • Add those PersonInfo objects in arraylist/ doublylinked list persons. • Close the stream with the file Read records from a text file named persons.txt. The person records will be present in the file in the following format. Add at least 50 records in text file, Ali,defence,9201211 Usman,gulberg,5173940 Salman,Johar Town,5272670 persons.txt AddressBook by using doubly LinkedList Code Are: Person.java public class Person{ //declaring instance variables private String name; //variable used to store name of Person private String address; //variable used to store address of Person private long phoneNumber; //variable used to store phone number of Person //declaring constructor public Person(String name, String address, long phoneNumber){ //assign the values to each of instance variables this.name=name; this.address=address; this.phoneNumber=phoneNumber; } //getter metod to return name public String getName(){ return this.name; } //getter metod to return address public String getAddress(){ return this.address; } //getter metod to return phoneNumber public long getPhoneNumber(){ return this.phoneNumber; } //returning entire instance in form of String public String toString(){ return "Name: "+this.name+" Address: "+this.address+" Phone Number: "+this.phoneNumber; //return all instance variables } } Main.java import java.util.Scanner; import java.util.List; import java.util.ArrayList; import java.util.LinkedList; public class Main { public static void main(String[] args) { List AddressBook; //declare a list Scanner sc=new Scanner(System.in); //display menu for type of list System.out.println("1.Enter 1 for using Doubly Linked List"); System.out.println("2.Enter 2 for using Array List"); int option=Integer.parseInt(sc.nextLine()); //input option if(option==1) //if user enters 1 AddressBook=new LinkedList(); //create using LinkedList else //if user enters 2 AddressBook=new ArrayList(); //create using ArrayList System.out.println("1.Add"); System.out.println("2.Delete"); System.out.println("3.Search"); System.out.println("4.Exit"); while(true){ System.out.print("Enter the choice: "); int choice=Integer.parseInt(sc.nextLine()); if(choice==1){ System.out.print("Enter Name: "); String name=sc.nextLine(); System.out.print("Enter Address: "); String address=sc.nextLine(); System.out.print("Enter Phone Number: "); long phoneNumber=Long.parseLong(sc.nextLine()); Person p=new Person(name,address,phoneNumber); AddressBook.add(p); System.out.println("Person added succesfully"); } else if(choice==2){ System.out.print("Enter Name: "); String inputName=sc.nextLine(); for(Person p:AddressBook){ if(p.getName().equals(inputName)){ AddressBook.remove(p); System.out.println("Person deleted succesfully"); } } } else if(choice==3){ System.out.print("Enter Name: "); String inputName=sc.nextLine(); for(Person p:AddressBook){ if(p.getName().equals(inputName)){ System.out.println(p); } } } else if(choice==4){ System.exit(0); } else{ System.out.println("Invalid Choice!Try again"); } } } }
Course:Data Structure and
Kindly do this correct as soon as possible:
I am providing the a menu base programs in java for address book by using doubly LinkedList and arrayList in a menu which are following:
1.Enter 1 for using Doubly linkedList
2.Enter 2 for using arrayList
YOU have to use File handling which create person.text file like this:
Perform these steps while application is loading up. You can add a new method loadPersons.
• Establish a data channel with a file by using streams
• Start reading data (person records) from file line by line
• Construct PersonInfo objects from each line you have read
• Add those PersonInfo objects in arraylist/ doublylinked list persons.
• Close the stream with the file
Read records from a text file named persons.txt. The person records will be present in the
file in the following format. Add at least 50 records in text file,
Ali,defence,9201211
Usman,gulberg,5173940
Salman,Johar Town,5272670
persons.txt
AddressBook by using doubly LinkedList Code Are:
public class Person{
//declaring instance variables
private String name; //variable used to store name of Person
private String address; //variable used to store address of Person
private long phoneNumber; //variable used to store phone number of Person
//declaring constructor
public Person(String name, String address, long phoneNumber){
//assign the values to each of instance variables
this.name=name;
this.address=address;
this.phoneNumber=phoneNumber;
}
//getter metod to return name
public String getName(){
return this.name;
}
//getter metod to return address
public String getAddress(){
return this.address;
}
//getter metod to return phoneNumber
public long getPhoneNumber(){
return this.phoneNumber;
}
//returning entire instance in form of String
public String toString(){
return "Name: "+this.name+" Address: "+this.address+" Phone Number: "+this.phoneNumber; //return all instance variables
}
}
import java.util.Scanner;
import java.util.List;
import java.util.ArrayList;
import java.util.LinkedList;
public class Main
{
public static void main(String[] args) {
List<Person> AddressBook; //declare a list
Scanner sc=new Scanner(System.in);
//display menu for type of list
System.out.println("1.Enter 1 for using Doubly Linked List");
System.out.println("2.Enter 2 for using Array List");
int option=Integer.parseInt(sc.nextLine()); //input option
if(option==1) //if user enters 1
AddressBook=new LinkedList<Person>(); //create using LinkedList
else //if user enters 2
AddressBook=new ArrayList<Person>(); //create using ArrayList
System.out.println("1.Add");
System.out.println("2.Delete");
System.out.println("3.Search");
System.out.println("4.Exit");
while(true){
System.out.print("Enter the choice: ");
int choice=Integer.parseInt(sc.nextLine());
if(choice==1){
System.out.print("Enter Name: ");
String name=sc.nextLine();
System.out.print("Enter Address: ");
String address=sc.nextLine();
System.out.print("Enter Phone Number: ");
long phoneNumber=Long.parseLong(sc.nextLine());
Person p=new Person(name,address,phoneNumber);
AddressBook.add(p);
System.out.println("Person added succesfully");
}
else if(choice==2){
System.out.print("Enter Name: ");
String inputName=sc.nextLine();
for(Person p:AddressBook){
if(p.getName().equals(inputName)){
AddressBook.remove(p);
System.out.println("Person deleted succesfully");
}
}
}
else if(choice==3){
System.out.print("Enter Name: ");
String inputName=sc.nextLine();
for(Person p:AddressBook){
if(p.getName().equals(inputName)){
System.out.println(p);
}
}
}
else if(choice==4){
System.exit(0);
}
else{
System.out.println("Invalid Choice!Try again");
}
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

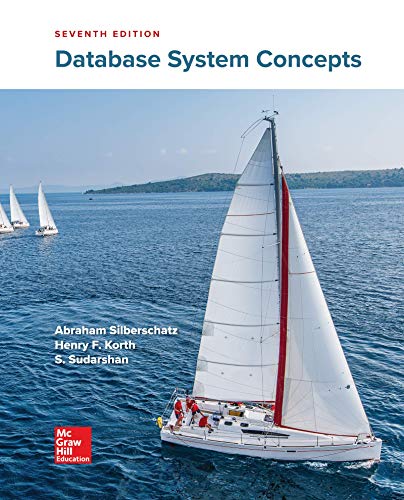
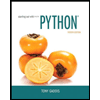
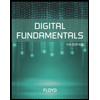
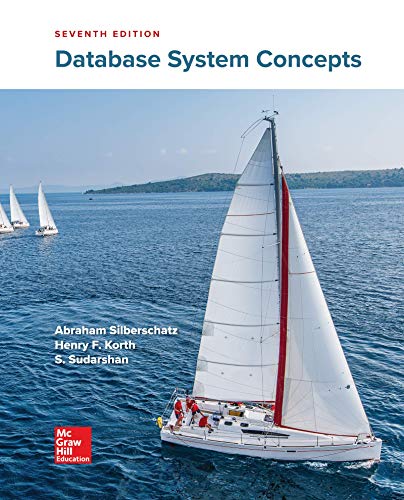
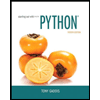
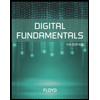
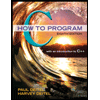
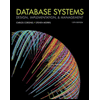
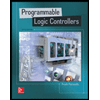