You are doing fun math problems. Given a string of combination of '{}', '[]', '()', you are required to return the substring included by the outermost '{}'. You need to write your code in one line. Args: target (str): the string to search in Returns: str >>> mathmatic('{[[[[]()]]]}') '[[[[]()]]]' >>> mathmatic('[(){([{}])}]') '([{}])' """ pass test code: # print(mathmatic('{[[[[]()]]]}')) # print(mathmatic('[(){([{}])}]'))
Regex, APIs, BeautifulSoup: python
import requests, re
from pprint import pprint
from bs4 import BeautifulSoup
complete the missing bodies of the functions below:
def mathmatic(target):
"""
Question 1
You are doing fun math problems. Given a string of combination of '{}', '[]',
'()', you are required to return the substring included by the outermost '{}'.
You need to write your code in one line.
Args:
target (str): the string to search in
Returns:
str
>>> mathmatic('{[[[[]()]]]}')
'[[[[]()]]]'
>>> mathmatic('[(){([{}])}]')
'([{}])'
"""
pass
test code:
# print(mathmatic('{[[[[]()]]]}'))
# print(mathmatic('[(){([{}])}]'))

Python:
Python is high level general purpose programming language.
It was developed by Guido Van Rossum in 1991.
It has simple syntax and dynamic semantics.
It uses indentation instead of curly braces.
It supports both procedural and object oriented programming approaches.
Step by step
Solved in 2 steps with 1 images

Could you help me with this one too, please
Regex, APIs, BeautifulSoup: python
import requests, re
from pprint import pprint
from bs4 import BeautifulSoup
complete the missing bodies of the functions below:
"""
Question 2
- Your friends are blowing up your group chat. Given a string of text messages
from your friends and a specific friend's name, return the first text message
they sent, excluding their name.
- Each text message ends with either a ?, !, or .
- Your code must be written in one line.
text_message (astr)
friend (astr)
Returns:
str of first match
>>> text_message = "Madison: How are you guys going today?" + \
"Anna: I'm doing pretty well!" + \
"Madison: That's good to hear. How is everyone else?"
>>> friend = "Madison"
>>>group_chat(text_message, friend)
How are you guys going today?
"""
pass
test code:
# text_message = "Madison: How are you guys going today?" + \
# "Anna: I'm doing pretty well!" + \
# "Madison: That's good to hear. How is everyone else?"
# friend1 = "Madison"
# print(group_chat(text_message, friend1))
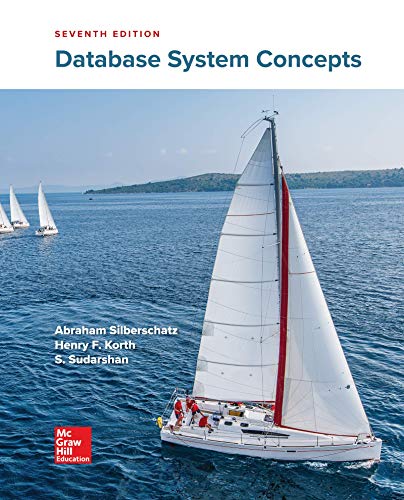
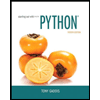
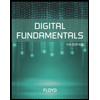
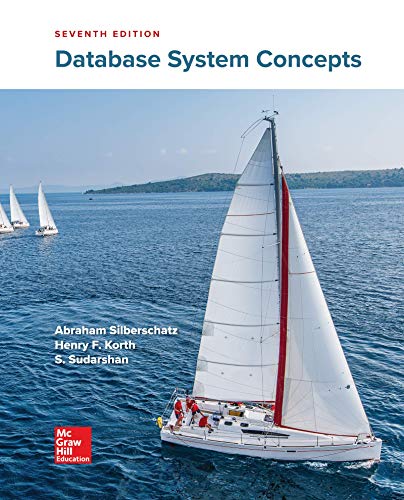
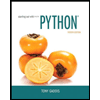
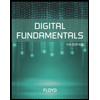
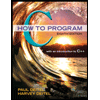
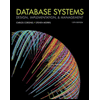
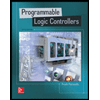