Could you fix the mistakes in this code so that it works. I’m having trouble making it work on visual studios. #include #include #include using namespace std; // Function to count the total number of "$"s in the economy matrix int CountTotalMoney(const vector>& matrix) { int count = 0; for (const auto& row : matrix) { for (const char& cell : row) { if (cell == '$') { count++; } } } return count; } // Function to recursively count the size of the money pot int CountMoneyPotSize(const vector>& matrix, vector>& visited, int row, int col) { if (row < 0 || col < 0 || row >= matrix.size() || col >= matrix[0].size() || visited[row][col]) { return 0; } visited[row][col] = true; if (matrix[row][col] != '$') { return 0; } int size = 1; size += CountMoneyPotSize(matrix, visited, row - 1, col); // Up size += CountMoneyPotSize(matrix, visited, row + 1, col); // Down size += CountMoneyPotSize(matrix, visited, row, col - 1); // Left size += CountMoneyPotSize(matrix, visited, row, col + 1); // Right return size; } // Function to read data from file and store it into a data structure vector> ReadDataFromFile(const string& filename) { ifstream file(filename); vector> matrix; if (file.is_open()) { string line; while (getline(file, line)) { vector row(line.begin(), line.end()); matrix.push_back(row); } file.close(); } return matrix; } int main() { vector> matrix = ReadDataFromFile("Payday.txt"); // Outputting the matrix for (const auto& row : matrix) { for (const char& cell : row) { cout << cell; } cout << endl; } // Counting total "$"s int totalMoney = CountTotalMoney(matrix); cout << "Total Money: " << totalMoney << endl; // Testing CountMoneyPotSize vector> visited(matrix.size(), vector(matrix[0].size(), false)); int startRow = 0; int startCol = 4; int moneyPotSize = CountMoneyPotSize(matrix, visited, startRow, startCol); cout << "Money Pot Size: " << moneyPotSize << endl; return 0; }
Could you fix the mistakes in this code so that it works. I’m having trouble making it work on visual studios. #include #include #include using namespace std; // Function to count the total number of "$"s in the economy matrix int CountTotalMoney(const vector>& matrix) { int count = 0; for (const auto& row : matrix) { for (const char& cell : row) { if (cell == '$') { count++; } } } return count; } // Function to recursively count the size of the money pot int CountMoneyPotSize(const vector>& matrix, vector>& visited, int row, int col) { if (row < 0 || col < 0 || row >= matrix.size() || col >= matrix[0].size() || visited[row][col]) { return 0; } visited[row][col] = true; if (matrix[row][col] != '$') { return 0; } int size = 1; size += CountMoneyPotSize(matrix, visited, row - 1, col); // Up size += CountMoneyPotSize(matrix, visited, row + 1, col); // Down size += CountMoneyPotSize(matrix, visited, row, col - 1); // Left size += CountMoneyPotSize(matrix, visited, row, col + 1); // Right return size; } // Function to read data from file and store it into a data structure vector> ReadDataFromFile(const string& filename) { ifstream file(filename); vector> matrix; if (file.is_open()) { string line; while (getline(file, line)) { vector row(line.begin(), line.end()); matrix.push_back(row); } file.close(); } return matrix; } int main() { vector> matrix = ReadDataFromFile("Payday.txt"); // Outputting the matrix for (const auto& row : matrix) { for (const char& cell : row) { cout << cell; } cout << endl; } // Counting total "$"s int totalMoney = CountTotalMoney(matrix); cout << "Total Money: " << totalMoney << endl; // Testing CountMoneyPotSize vector> visited(matrix.size(), vector(matrix[0].size(), false)); int startRow = 0; int startCol = 4; int moneyPotSize = CountMoneyPotSize(matrix, visited, startRow, startCol); cout << "Money Pot Size: " << moneyPotSize << endl; return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Could you fix the mistakes in this code so that it works. I’m having trouble making it work on visual studios. #include
#include
#include
using namespace std;
// Function to count the total number of "$"s in the economy matrix
int CountTotalMoney(const vector >& matrix) {
int count = 0;
for (const auto& row : matrix) {
for (const char& cell : row) {
if (cell == '$') {
count++;
}
}
}
return count;
}
// Function to recursively count the size of the money pot
int CountMoneyPotSize(const vector>& matrix, vector>& visited, int row, int col) {
if (row < 0 || col < 0 || row >= matrix.size() || col >= matrix[0].size() || visited[row][col]) {
return 0;
}
visited[row][col] = true;
if (matrix[row][col] != '$') {
return 0;
}
int size = 1;
size += CountMoneyPotSize(matrix, visited, row - 1, col); // Up
size += CountMoneyPotSize(matrix, visited, row + 1, col); // Down
size += CountMoneyPotSize(matrix, visited, row, col - 1); // Left
size += CountMoneyPotSize(matrix, visited, row, col + 1); // Right
return size;
}
// Function to read data from file and store it into a data structure
vector> ReadDataFromFile(const string& filename) {
ifstream file(filename);
vector> matrix;
if (file.is_open()) {
string line;
while (getline(file, line)) {
vector row(line.begin(), line.end());
matrix.push_back(row);
}
file.close();
}
return matrix;
}
int main() {
vector> matrix = ReadDataFromFile("Payday.txt");
// Outputting the matrix
for (const auto& row : matrix) {
for (const char& cell : row) {
cout << cell;
}
cout << endl;
}
// Counting total "$"s
int totalMoney = CountTotalMoney(matrix);
cout << "Total Money: " << totalMoney << endl;
// Testing CountMoneyPotSize
vector> visited(matrix.size(), vector(matrix[0].size(), false));
int startRow = 0;
int startCol = 4;
int moneyPotSize = CountMoneyPotSize(matrix, visited, startRow, startCol);
cout << "Money Pot Size: " << moneyPotSize << endl;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 7 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
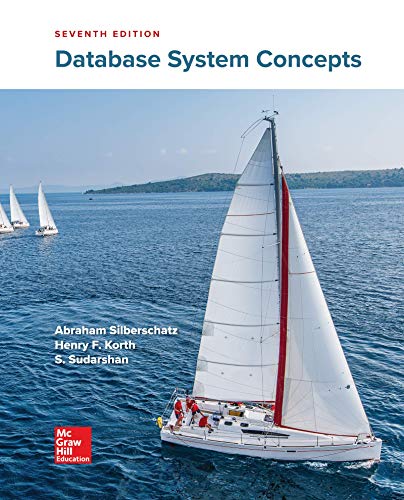
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
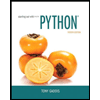
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
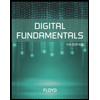
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
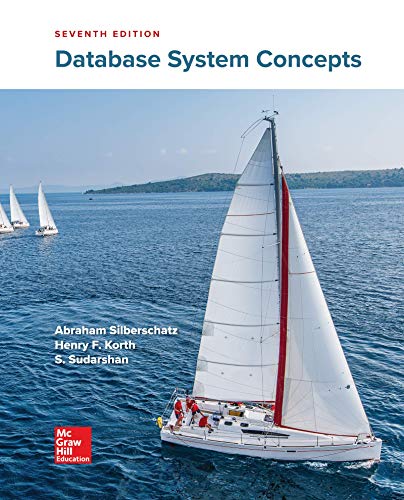
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
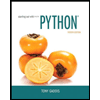
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
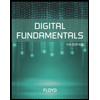
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
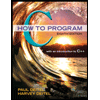
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
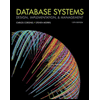
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
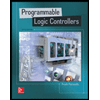
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education