PLEASE HELP ME WITH THIS! THANK YOU! Develop a program that will define and store a student record. The data will be entered by the user. Afterwards, calculate and display the GPA of each student. You will need to use structure with array. In case you don't know how to calculate GPA, here's the formula: Sample Console Input Expected Console Output 2 John Anthony 10912345 ECE Physics 100 3 Mathematics 70 2 Geology 50 1 Jose 10854321 CPE Physics 80 3 Mathematics 60 2 Geology 90 1 Enter number of students to record: 2 student name: John Anthony ID number: 10912345 degree: ECE course 1 name: Physics course 1 grade: 100 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 70 course 2 total units: 2 course 3 name: Geology course 3 grade: 50 course 3 total units: 1 student name: Jose ID number: 10854321 degree: CPE course 1 name: Physics course 1 grade: 80 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 60 course 2 total units: 2 course 3 name: Geology course 3 grade: 90 course 3 total units: 1 John Anthony with ID number 10912345 has a GPA of 81.667 Jose with ID number 10854321 has a GPA of 75.000 -1 2 John 10912345 ECE Physics 100 3 Mathematics 70 2 Geology 50 1 Jose 10854321 CPE Physics 80 3 Mathematics 60 2 Geology 90 1 Enter number of students to record: -1 Enter number of students to record: 2 student name: John ID number: 10912345 degree: ECE course 1 name: Physics course 1 grade: 100 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 70 course 2 total units: 2 course 3 name: Geology course 3 grade: 50 course 3 total units: 1 student name: Jose ID number: 10854321 degree: CPE course 1 name: Physics course 1 grade: 80 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 60 course 2 total units: 2 course 3 name: Geology course 3 grade: 90 course 3 total units: 1 John with ID number 10912345 has a GPA of 81.667 Jose with ID number 10854321 has a GPA of 75.000 0 2 John 10912345 ECE Physics 100 3 Mathematics 70 2 Geology 50 1 Jose 10854321 CPE Physics 80 3 Mathematics 60 2 Geology 90 1 Enter number of students to record: 0 Enter number of students to record: 2 student name: John ID number: 10912345 degree: ECE course 1 name: Physics course 1 grade: 100 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 70 course 2 total units: 2 course 3 name: Geology course 3 grade: 50 course 3 total units: 1 student name: Jose ID number: 10854321 degree: CPE course 1 name: Physics course 1 grade: 80 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 60 course 2 total units: 2 course 3 name: Geology course 3 grade: 90 course 3 total units: 1 John with ID number 10912345 has a GPA of 81.667 Jose with ID number 10854321 has a GPA of 75.000 Structure Guideline Structure #1: Course Name Type Description name char(80) Stores the name of the course. Max. Length of 80 characters unit int Stores the no. of units on the course. grade float Stores the grade of a student on the course. Structure #2: Student Name Type Description id int Stores the ID number of the student. name char(80) Stores the name of the student. Max. Length of 80 characters degree char(80) Stores the degree program of the student courses Course(TOTAL_COURSE) Uses the Course Data Structure to store course content GPA float Stores the grade of a student on the course. Testing Guideline: Make sure to use the TOTAL_COURSE MACRO from your code. Please use it to define the number of courses per student Make sure that the user can enter any value of value of N. Hint: In order for you to get dynamically allocate number of arrays of type Student, you can use the malloc function you've seen from your homework as shown below: Student *record; record = (Student *)malloc(N * sizeof(Student)); where N is the number of students entered by the user. PLEASE MODIFY THESE CODES TO MAKE THE PROGRAM WORK.
PLEASE HELP ME WITH THIS! THANK YOU!
Develop a program that will define and store a student record. The data will be entered by the user. Afterwards, calculate and display the GPA of each student. You will need to use structure with array.
In case you don't know how to calculate GPA, here's the formula:
Sample Console Input | Expected Console Output |
---|---|
2 John Anthony 10912345 ECE Physics 100 3 Mathematics 70 2 Geology 50 1 Jose 10854321 CPE Physics 80 3 Mathematics 60 2 Geology 90 1 |
Enter number of students to record: 2 student name: John Anthony ID number: 10912345 degree: ECE course 1 name: Physics course 1 grade: 100 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 70 course 2 total units: 2 course 3 name: Geology course 3 grade: 50 course 3 total units: 1 student name: Jose ID number: 10854321 degree: CPE course 1 name: Physics course 1 grade: 80 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 60 course 2 total units: 2 course 3 name: Geology course 3 grade: 90 course 3 total units: 1 John Anthony with ID number 10912345 has a GPA of 81.667 Jose with ID number 10854321 has a GPA of 75.000 |
-1 2 John 10912345 ECE Physics 100 3 Mathematics 70 2 Geology 50 1 Jose 10854321 CPE Physics 80 3 Mathematics 60 2 Geology 90 1 |
Enter number of students to record: -1 Enter number of students to record: 2 student name: John ID number: 10912345 degree: ECE course 1 name: Physics course 1 grade: 100 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 70 course 2 total units: 2 course 3 name: Geology course 3 grade: 50 course 3 total units: 1 student name: Jose ID number: 10854321 degree: CPE course 1 name: Physics course 1 grade: 80 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 60 course 2 total units: 2 course 3 name: Geology course 3 grade: 90 course 3 total units: 1 John with ID number 10912345 has a GPA of 81.667 Jose with ID number 10854321 has a GPA of 75.000 |
0 2 John 10912345 ECE Physics 100 3 Mathematics 70 2 Geology 50 1 Jose 10854321 CPE Physics 80 3 Mathematics 60 2 Geology 90 1 |
Enter number of students to record: 0 Enter number of students to record: 2 student name: John ID number: 10912345 degree: ECE course 1 name: Physics course 1 grade: 100 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 70 course 2 total units: 2 course 3 name: Geology course 3 grade: 50 course 3 total units: 1 student name: Jose ID number: 10854321 degree: CPE course 1 name: Physics course 1 grade: 80 course 1 total units: 3 course 2 name: Mathematics course 2 grade: 60 course 2 total units: 2 course 3 name: Geology course 3 grade: 90 course 3 total units: 1 John with ID number 10912345 has a GPA of 81.667 Jose with ID number 10854321 has a GPA of 75.000 |
Structure Guideline
Structure #1: Course
Name | Type | Description |
---|---|---|
name | char(80) | Stores the name of the course. Max. Length of 80 characters |
unit | int | Stores the no. of units on the course. |
grade | float | Stores the grade of a student on the course. |
Structure #2: Student
Name | Type | Description |
---|---|---|
id | int | Stores the ID number of the student. |
name | char(80) | Stores the name of the student. Max. Length of 80 characters |
degree | char(80) | Stores the degree program of the student |
courses | Course(TOTAL_COURSE) | Uses the Course Data Structure to store course content |
GPA | float | Stores the grade of a student on the course. |
Testing Guideline:
- Make sure to use the TOTAL_COURSE MACRO from your code. Please use it to define the number of courses per student
- Make sure that the user can enter any value of value of N.
Hint:
In order for you to get dynamically allocate number of arrays of type Student, you can use the malloc function you've seen from your homework as shown below:
Student *record; record = (Student *)malloc(N * sizeof(Student));
where N is the number of students entered by the user.
PLEASE MODIFY THESE CODES TO MAKE THE PROGRAM WORK.
![//
***
** MODIFY ME!!! ***
********
3
#include <stdio.h>
4
#include <string.h>
#include "lab4_ex1.h"
// Removes or cleans the dangling 'new line' character in stdin
// @param void
// @return none
8
9
10
11
void flush()
12
{
13
char c;
14
while ((c
= getchar()) != '\n' && c != E0F)
15
16
}
17
// Creates the course (one course only) by asking the for user input
// @param num course number, e.g. 1,2,3
// @return course
18
19
20
21
Course createCourse(int num)
22
{
23
Course course;
24
// ******
***** YOUR CODE HERE *****
25
return course;
26
}
27
// Displays the course
// @param course number, e.g. 1, 2, 3
// @return none
void displayCourse(Course course)
28
29
30
31
32
{
// printf("course name: %s\n", course.name);
// printf("course grade: %0.2f\n", course.grade);
// printf("course units: %d\n", course.unit);
33
34
35
36
}
37
// Computes the GPA from three courses, based on the formula in README.md
// @param courses [TOTAL_COURSE]
// @return GPA
float computeGPA(Course courses [TOTAL_COURSE] )
38
39
40
41
42
{
43
// **
YOUR CODE HERE](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6f4adae0-2fda-4c43-b97b-09c46a19ec3e%2F9759f96d-80a4-4ef6-beb1-2f09c6ae58bf%2Fng4pmg1_processed.png&w=3840&q=75)
![42
{
43
//
YOUR CODE HERE
44
return 0.0f;
45
}
46
// Creates the student by asking for user input
// @param none
// @return student structure with id, name, degree, courses, and GPA
Student createStudent ()
47
48
49
50
51
{
52
Student student;
53
|// ********
YOUR CODE HERE
54
return student;
55
}
56
// Displays the student
// @param student struct
// @return none
void displayStudent (Student student)
57
58
59
60
61
{
// printf("student name: %s\n", student.name);
// printf("ID number: %d\n", student.id);
// printf("degree: %s\n", student.degree);
// for (int i = 0; i < T0TAL_COURSE; i++)
// {
62
63
64
65
66
displayCourse(student.courses [i]);
// }
67
//
68
69
}
70
// Displays the student with GPA
// @param student struct
// @return none
void displayStudentGPA(Student student)
71
72
73
74
75
{
// printf("&s with ID number %d has a GPA of %.3f\n", student.name, student.id, student.GPA);
76
77
}
78](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6f4adae0-2fda-4c43-b97b-09c46a19ec3e%2F9759f96d-80a4-4ef6-beb1-2f09c6ae58bf%2Fr4f97b7_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 2 images

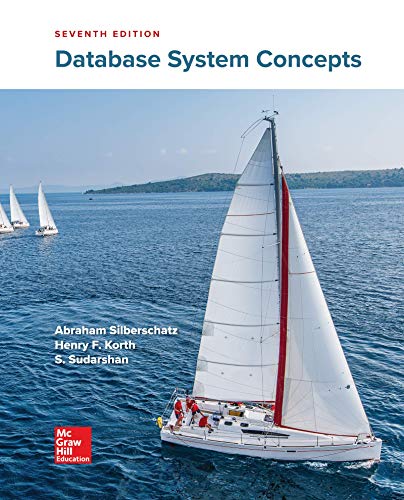
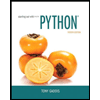
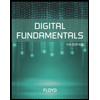
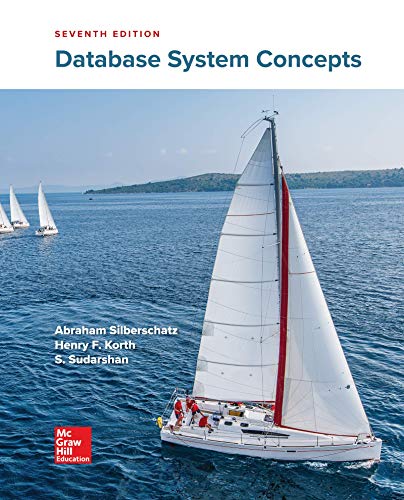
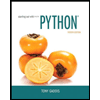
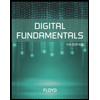
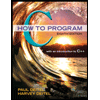
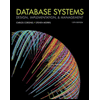
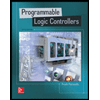