Correct logic error and modify the C++ code. 1-Use loop 3 times to ask pin from user when user enter wrong pin 3 times then excute the program. 2-if user correct guess before tries excute and ask if you play again if user say no excute the program. 3-Correct line Geneerate random number between 1 to 50. 4-Correct logic error if you found anyothers. Code: //included header file for basic input output stream #include //included header file for file stream #include //included header file to use rand() function #include //included header file to use time() function #include //included namespace using namespace std; //function to generate random number int generateRandom() { //use srand() function to change the random number after each execution srand((unsigned int) time(0)); //generated a random number between 1-50 through rand() function int randomNumber = rand()%50; //return random number to main() return randomNumber; } //function tooLow() void tooLow() { //print statement to print the message cout << "You have guessed too low." << endl; } //function tooHigh() void tooHigh() { //print statement to print the message cout << "You have guessed too high." << endl; } //function guessedCorrect() void guessedCorrect() { //print statement to print the message cout << "You have guessed correct." << endl; } //function to check guess void checkGuess(int guess, int randomNumber) { //If the users guesses below the number if (guess < randomNumber) //call a function tooLow() tooLow(); //If they guess over that number elseif (guess > randomNumber) //call a function tooHigh() tooHigh(); //else statement else //call a function guessedCorrect() guessedCorrect(); } //function to start game void guessGame() { //welcome message cout << "------------Welcome to the game------------" << endl; //variable declaration int guess, level, trial = 0, numberOfTries; cout << "Enter level of game: " << endl; cout << "Press 1 for easy level" << endl; cout << "Press 2 for medium level" << endl; cout << "Press 3 for hard level" << endl; //input level cin >> level; //switch level switch (level) { //case 1 case1: //assign numberOfTries to 8 numberOfTries = 8; //break break; //case 2 case2: //assign numberOfTries to 5 numberOfTries = 5; //break break; //case 3 case3: //assign numberOfTries to 2 numberOfTries = 2; //break break; //default case default: //display message "Wrong choice entered." cout << "Wrong choice entered." << endl; } //while loop run until numberOfTries while (trial < numberOfTries) { cout << "Enter your guess: "; //input number from user cin >> guess; //calling generateRandom int randomNumber = generateRandom(); //calling checkGuess() checkGuess(guess, randomNumber); //increment trial trial++; } } //main function int main() { //declared variable guess int pin, filePin; //created file ifstream file; //opening file file.open("gamePin.txt"); //input pin from user cout << "Enter pin: "; cin >> pin; //extract pin from file file >> filePin; //compare user pin and pin in file if (pin == filePin) //calling guessGame() guessGame(); //else statement else //display message "Wrong pin" cout << "Wrong pin" << endl; return0; }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

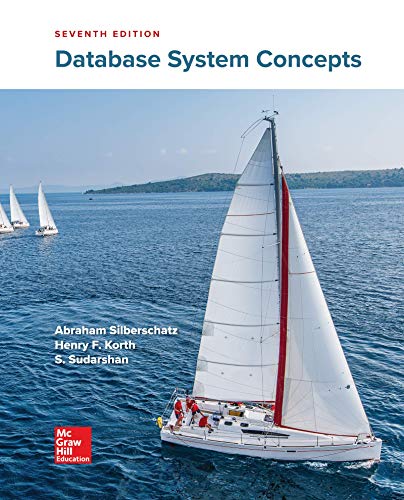
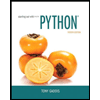
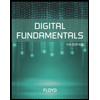
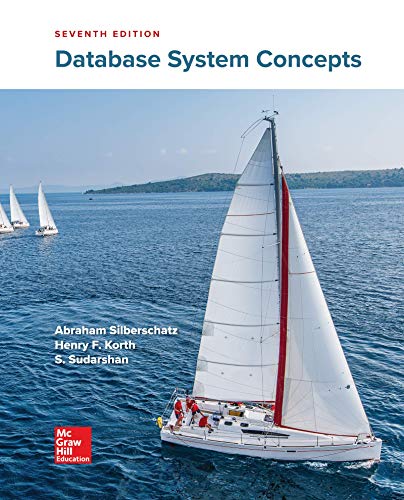
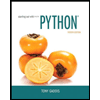
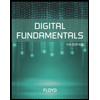
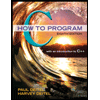
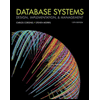
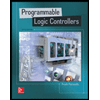