in C Create a do while loop. Inside the loop intake an age as an integer. If the age is less than 21 then the loop should repeat until an age over 21 is inputted.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
in C
Create a do while loop. Inside the loop intake an age as an integer. If the age is less than
21 then the loop should repeat until an age over 21 is inputted.

The algorithm for the C program uses a do-while loop to continuously prompt the user for their age until they enter an age greater than or equal to 21:
- Initialize Variables:
- Declare an integer variable age to store the user's input.
- Do-While Loop:
- Start a do-while loop that will execute the following steps at least once:
- Prompt for Age:
- Display a message asking the user to enter their age.
- Read User Input:
- Read the user input as an integer and store it in the age variable.
- Check Age:
- Check if the value of age is less than 21.
- Age Validation:
- If age is less than 21:
- Display a message informing the user that they must be at least 21 years old to continue.
- Repeat the loop (go back to step 3).
- If age is less than 21:
- Prompt for Age:
- Exit Loop:
- Once the value of age is greater than or equal to 21, exit the loop.
- Display Confirmation Message:
- Display a message thanking the user for entering a valid age.
- Program End:
- End the program.
Step by step
Solved in 3 steps with 3 images

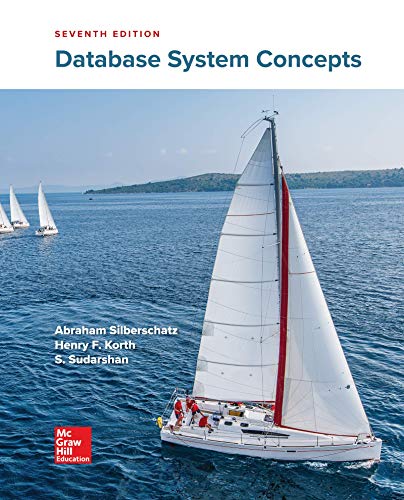
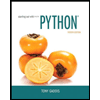
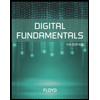
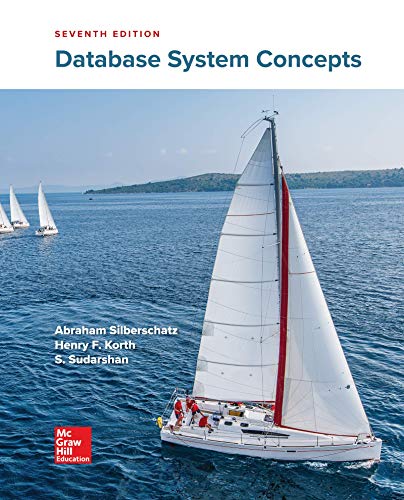
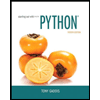
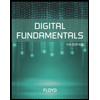
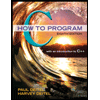
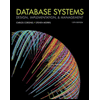
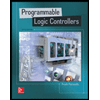