/** * Converts an uppercase letter to its circled counterpart. * * @precondition 'A' <= letter <= 'Z' * @postcondition none * * @param letter the uppercase letter to convert * @return the circled version of the uppercase letter */ public static char convertUppercaseLetter(char letter) { if (letter < 'A' || letter > 'Z') { throw new IllegalArgumentException("letter must be uppercase"); } return (char)(letter + 9333); } when the parameter is one less than the minimum allowed when the parameter is at the minimum allowed when the parameter is one more than the minimum allowed when the parameter is one less than the maximum allowed when the parameter is at the maximum allowed when the parameter is one more than the maximum allowed
I am trying to create a J-Unit test class for the following method:
/**
* Converts an uppercase letter to its circled counterpart.
*
* @precondition 'A' <= letter <= 'Z'
* @postcondition none
*
* @param letter the uppercase letter to convert
* @return the circled version of the uppercase letter
*/
public static char convertUppercaseLetter(char letter) {
if (letter < 'A' || letter > 'Z') {
throw new IllegalArgumentException("letter must be uppercase");
}
return (char)(letter + 9333);
}
- when the parameter is one less than the minimum allowed
- when the parameter is at the minimum allowed
- when the parameter is one more than the minimum allowed
- when the parameter is one less than the maximum allowed
- when the parameter is at the maximum allowed
- when the parameter is one more than the maximum allowed

This code will convert the given letter to its circled version. If the given letter is not in uppercase then it will throw an error. If the given letter is not in the alpha range then it will throw an invalid error.
Code :
public class Main
{
public static void main(String[] args)
{
Scanner scan = new Scanner(System.in);
System.out.print("Enter an Alphabet: ");
char chUpper = scan.next().charAt(0);
int ascii = chUpper;
if(ascii>=65 && ascii<=90)
{
ascii = ascii + 32;
char chLower = (char)ascii;
System.out.println("\nCircled version = " +chLower);
}
else if(ascii>=97 && ascii<=122)
System.out.println("\nLetter must be in Uppercase");
else
System.out.println("\nInvalid Input!");
}
}
Step by step
Solved in 2 steps with 4 images

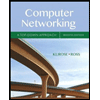
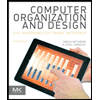
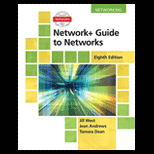
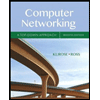
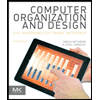
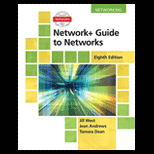
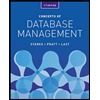
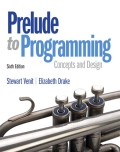
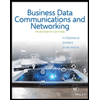