Convert the C code below into Pseuedocode ______________________________________________________________ #include #define max 6 int count = 0, countSpan = 0, cost = 0; struct edge{ int x, y; int value; }; edge list[max]; edge spanning[max]; int graph[max][max], n; int find(int *belong, int node){ return(belong[node]); } void sort(){ edge temp; for (int i = 1; i < count; i++){ for (int j = 0; j < count - 1; j++){ if (list[j].value > list[j+1].value) { temp = list[j]; list[j] = list[j+1]; list[j + 1] = temp; } } } } void kruskal(){ int belong[max], node1, node2; for(int i = 1; i < max; i++){ for(int j = 0; j < i; j++){ if(graph[i][j] != 0){ list[count].x = i; list[count].y = j; list[count].value = graph[i][j]; count++; } } } sort(); for(int i = 0; i < max; i++){ belong[i] = i; } for(int i = 0; i < max; i++){ node1 = find(belong, list[i].x); node2 = find(belong, list[i].y); if(node1 != node2){ spanning[countSpan] = list[i]; cost += spanning[countSpan].value; countSpan++; for(int i = 0; i < max; i++){ if(belong[i] == node2){ belong[i] = node1; } } } } } void print(){ printf("%d\n", cost); for (int i = 0; i < countSpan; i++){ printf("%c - %c : %d\n", spanning[i].y+65, spanning[i].x+65, spanning[i].value); cost = cost + spanning[i].value; } } int main(){ char a, b; int value; for(int i = 0; i < 12; i++){ scanf("%c", &a); getchar(); scanf("%c", &b); getchar(); scanf("%d", &value); getchar(); list[i].value = value; graph[a-'A'][b-'A'] = graph[b-65][a-65] = value; } kruskal(); print(); return 0; }
Convert the C code below into Pseuedocode
______________________________________________________________
#include <stdio.h>
#define max 6
int count = 0, countSpan = 0, cost = 0;
struct edge{
int x, y;
int value;
};
edge list[max];
edge spanning[max];
int graph[max][max], n;
int find(int *belong, int node){
return(belong[node]);
}
void sort(){
edge temp;
for (int i = 1; i < count; i++){
for (int j = 0; j < count - 1; j++){
if (list[j].value > list[j+1].value) {
temp = list[j];
list[j] = list[j+1];
list[j + 1] = temp;
}
}
}
}
void kruskal(){
int belong[max], node1, node2;
for(int i = 1; i < max; i++){
for(int j = 0; j < i; j++){
if(graph[i][j] != 0){
list[count].x = i;
list[count].y = j;
list[count].value = graph[i][j];
count++;
}
}
}
sort();
for(int i = 0; i < max; i++){
belong[i] = i;
}
for(int i = 0; i < max; i++){
node1 = find(belong, list[i].x);
node2 = find(belong, list[i].y);
if(node1 != node2){
spanning[countSpan] = list[i];
cost += spanning[countSpan].value;
countSpan++;
for(int i = 0; i < max; i++){
if(belong[i] == node2){
belong[i] = node1;
}
}
}
}
}
void print(){
printf("%d\n", cost);
for (int i = 0; i < countSpan; i++){
printf("%c - %c : %d\n", spanning[i].y+65, spanning[i].x+65, spanning[i].value);
cost = cost + spanning[i].value;
}
}
int main(){
char a, b;
int value;
for(int i = 0; i < 12; i++){
scanf("%c", &a);
getchar();
scanf("%c", &b);
getchar();
scanf("%d", &value);
getchar();
list[i].value = value;
graph[a-'A'][b-'A'] = graph[b-65][a-65] = value;
}
kruskal();
print();
return 0;
}

Step by step
Solved in 3 steps

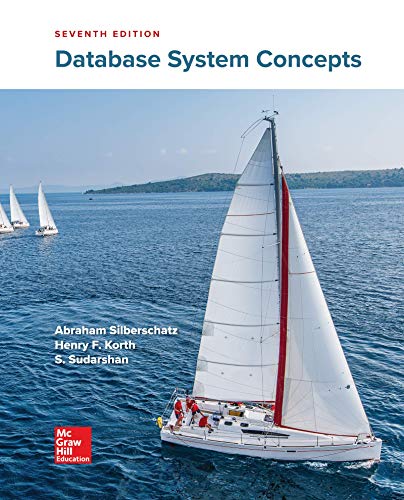
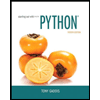
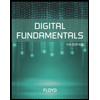
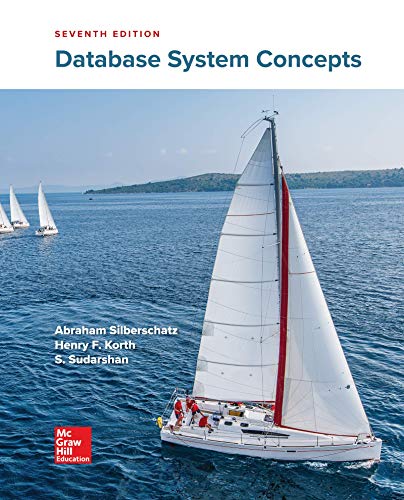
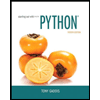
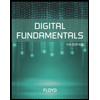
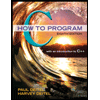
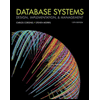
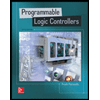