contain the array of struct Employee and proper operations on it. Choose array size to be 30. 4. Program must run successfully on empty file, file with more than 30 Employees, and less than 30. Keep employee array size to 30. In each case program must inform user properly. For example, if file has data more than the capacity of the array then user must be informed that file has excess data and only 30 employees would be processed. Suggestions for member and non-member function Design: 1. Provide get and set member functions for all necessary private data members. 2. Provide member function to calculate gross salary and required error correction. 3. Provide a struct member function that prints the employee data either to a file or to the standard output. The output is properly formatted. If employee gross salary was set to zero then function prints relevant message that there were calculation error due to bad file data. 4. Provide functions for sorting and searching based on different criteria.
contain the array of struct Employee and proper operations on it. Choose array size to be 30. 4. Program must run successfully on empty file, file with more than 30 Employees, and less than 30. Keep employee array size to 30. In each case program must inform user properly. For example, if file has data more than the capacity of the array then user must be informed that file has excess data and only 30 employees would be processed. Suggestions for member and non-member function Design: 1. Provide get and set member functions for all necessary private data members. 2. Provide member function to calculate gross salary and required error correction. 3. Provide a struct member function that prints the employee data either to a file or to the standard output. The output is properly formatted. If employee gross salary was set to zero then function prints relevant message that there were calculation error due to bad file data. 4. Provide functions for sorting and searching based on different criteria.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Employee Struct Array and Program Design**
**Array Size Requirement:**
The program should contain an array of `struct Employee` with appropriate operations. The chosen array size should be 30.
**Program Functionality:**
1. The program must successfully manage files containing employee data, accommodating scenarios where the file is empty, or contains greater or fewer than 30 employees. The employee array size must remain 30.
2. Users need to be properly informed in various situations:
- If the file's data exceeds the array's capacity, notify the user that only 30 employees will be processed.
**Function Design Suggestions:**
1. Provide getter and setter member functions for all necessary private data members.
2. Include a member function to calculate gross salary and perform necessary error corrections.
3. Implement a struct member function to print employee data to a file or standard output, ensuring the output is well-formatted. If the employee's gross salary is zero, the function should indicate a calculation error due to bad file data.
4. Provide functions for sorting and searching based on different criteria.

Transcribed Image Text:**Program Design and Output Specification for Employee Data Management**
This guide outlines the requirements for designing a program to manage employee data efficiently. The program should handle up to 30 employee records and is built with modularity in mind.
### Program Requirements
- **Data Capacity**: The program is designed to hold a maximum of 30 employee records. If a data file contains more than 30 records, the program should only read 30 and notify the user if the limit is exceeded. If the data file is empty, a message indicating this must be displayed.
- **Output Format**: The output should be structured as follows:
```
Name | ID# | Job Type | Gross Salary
Jack Smith | 1234 | Management | $2205.00
```
Ensure that the gross salary is checked for accuracy.
### Error Handling
If there are any issues with the data, such as:
- Employee code in lowercase
- Years of service exceeding 50
- Incorrect educational/job classification codes
The program must notify the user that the gross salary calculation is affected due to bad data.
### Output File and Sorting Requirements
The program should generate an output file with sorted employee data:
1. Unsorted Employee data displayed as above.
2. Sorted by Employee ID#.
3. Sorted by Last Name.
4. Sorted by Gross Salary.
### User Interaction
- Provide a menu for users to search the data by Employee ID or Last Name. If multiple matches are found for a last name, the first match should be displayed.
- Allow users multiple searches and the ability to exit after completing their searches.
- Implement either binary (requires sorted array) or linear search algorithms.
### Testing
Before final testing:
- Use an empty file, a file with over 30 records, and a file with fewer than 30 records for validation.
- Compare the program's output to a given data file sample, which will be provided.
### Design Considerations
1. **Data Structure Members**: Last name, ID, and gross salary must be included as struct members for robust design.
2. **Constructors**: Implement both default and explicit constructors.
3. **Data Structure Choice**: Decide between using one or two structs. If using two, one should be Employee and the other should be relevant to the business logic.
This design ensures efficient data handling and flexibility in searching and sorting employee records.
Expert Solution

Step 1
Here's a simple application, ArrayDemo (in a.java source file), that produces an array, fills it with values, and displays them.
public class ArrayDemo {
public static void main(String[] args) {
int[] anArray; // declare an array of integers
anArray = new int[10]; // create an array of integers
// assign a value to each array element and print
for (int i = 0; i < anArray.length; i++) {
anArray[i] = i;
System.out.print(anArray[i] + " ");
}
System.out.println();
}
}
The output from this program is:
0 1 2 3 4 5 6 7 8 9
- Declaring a Variable as An Array Reference
- Accessing an Array Element
- Getting the Size of an Array
- Creating an Array
- Array Initializers
Trending now
This is a popular solution!
Step by step
Solved in 6 steps

Recommended textbooks for you
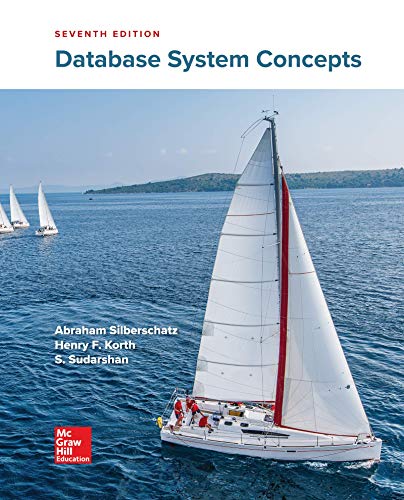
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
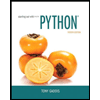
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
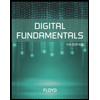
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
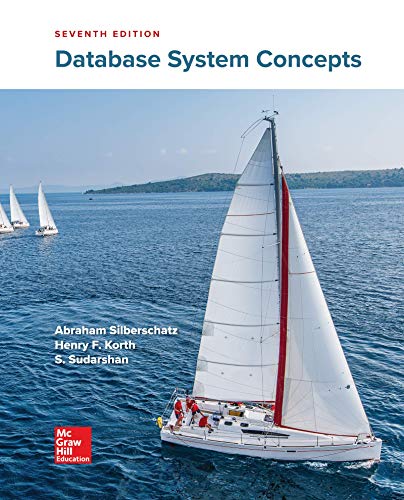
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
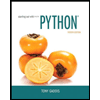
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
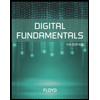
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
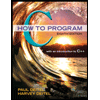
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
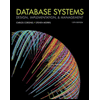
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
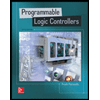
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education