Consider the following program. Assume all variables are declared and the program compiles. #include using namespace std; void getVal(int&, int&); void procVal(int, int&); int x; int main() { int intNum1; int intNum2; x = 6; getVal(intNum1, intNum2); cout << intNum1 << " " << intNum2 << " " << x << endl; procVal(intNum1, intNum2); cout << intNum1 << " " << intNum2 << " " << x << endl; return 0; } void getVal(int& a, int& b) { cout << “Enter value for a: “ << endl; cin >> a; /// The user enters 1 cout << “Enter value for b: “ << endl; cin >> b; /// The user enters 2 x = a * b; } void procVal(int u, int& v) { int intNum3; intNum3= x; v = intNum3 * 4; u = u - v; } Answer the following questions: What is the output in the cout statements? Consider variable scope.
- Consider the following program. Assume all variables are declared and the program compiles.
#include <iostream>
using namespace std;
void getVal(int&, int&);
void procVal(int, int&);
int x;
int main() {
int intNum1;
int intNum2;
x = 6;
getVal(intNum1, intNum2);
cout << intNum1 << " " << intNum2 << " " << x << endl;
procVal(intNum1, intNum2);
cout << intNum1 << " " << intNum2 << " " << x << endl;
return 0;
}
void getVal(int& a, int& b)
{
cout << “Enter value for a: “ << endl;
cin >> a; /// The user enters 1
cout << “Enter value for b: “ << endl;
cin >> b; /// The user enters 2
x = a * b;
}
void procVal(int u, int& v)
{
int intNum3;
intNum3= x;
v = intNum3 * 4;
u = u - v;
}
Answer the following questions:
- What is the output in the cout statements? Consider variable scope.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

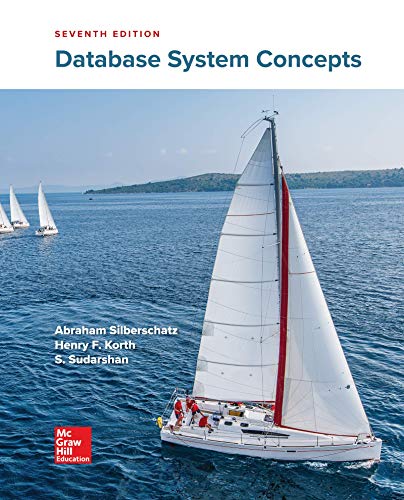
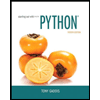
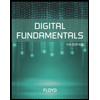
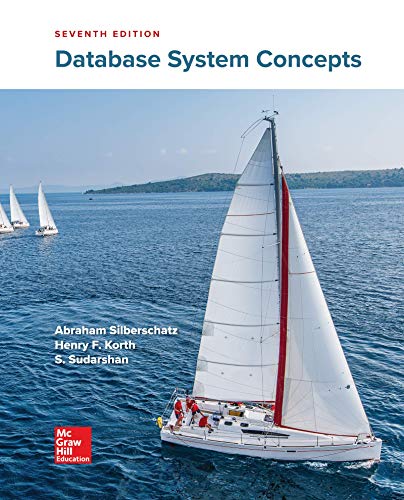
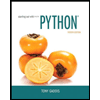
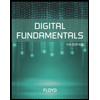
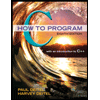
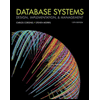
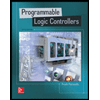