consider the following code * #include using namespace std; int main() { double wages, hours, OThours, OTamount, totalWages; double rate = 20.00; double OTrate = rate * 1.5; cout << "how many hours did you work this week?" << endl; cin >> hours; if (hours > 0 && hours <= 40) { wages = (rate * hours); totalWages = wages; } else if (hours > 40 && hours <= 60) { wages = (rate * 40); OThours = (hours - 40); cout << "OTHours = " << OThours << endl; OTamount = (OThours * OTrate); cout << "OTamount = " << OTamount << endl; totalWages = (wages + OTamount); } cout << "totalWages = " << totalWages << endl; } What is the OTamount if 50 hours were worked?
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
* consider the following code *
#include <iostream>
using namespace std;
int main()
{
double wages, hours, OThours, OTamount, totalWages;
double rate = 20.00;
double OTrate = rate * 1.5;
cout << "how many hours did you work this week?" << endl;
cin >> hours;
if (hours > 0 && hours <= 40)
{
wages = (rate * hours);
totalWages = wages;
}
else if (hours > 40 && hours <= 60)
{
wages = (rate * 40);
OThours = (hours - 40);
cout << "OTHours = " << OThours << endl;
OTamount = (OThours * OTrate);
cout << "OTamount = " << OTamount << endl;
totalWages = (wages + OTamount);
}
cout << "totalWages = " << totalWages << endl;
}
What is the OTamount if 50 hours were worked?

Step by step
Solved in 2 steps

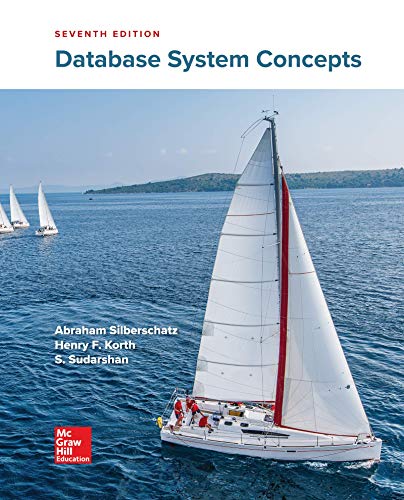
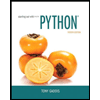
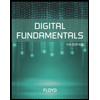
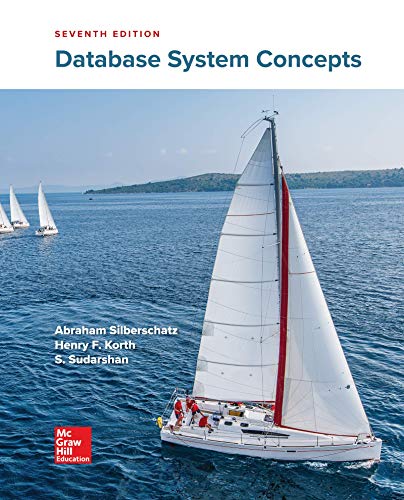
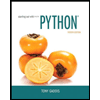
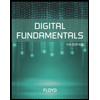
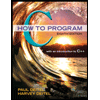
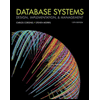
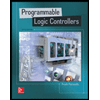