Consider the below code of circular linked list. Your work is to update the same code, by providing the functionalities with tail pointer only.
Consider the below code of circular linked list. Your work is to update the same code, by providing the functionalities with tail pointer only.
#include <iostream>
using namespace std;
class Node{
public:
int data;
Node *next;
};
class List{
public:
Node *head;
List(){
head = NULL;
}
void insert(int data){
Node *nn = new Node;
nn->data = data;
if(head==NULL){
head = nn;
nn->next = head;
cout<<"Node inserted successfully at head"<<endl;
}
else{
Node *temp = head;
while(temp->next!=head)
temp = temp->next;
nn->next = head;
temp->next=nn;
cout<<"Node inserted successfully at end"<<endl;
}
}
void insertInBetween(Node *node, int data){
Node *nn = new Node;
nn->data = data;
Node *temp = node->next;
node->next = nn;
nn->next = temp;
cout<<nn->data<<" inserted successfully after: "<<node->data<<endl;
}
void display(){
Node *temp = head;
while(temp->next!=head)
{
cout<<temp->data<<endl;
temp=temp->next;
}
cout<<temp->data<<endl;
}
void insertAtHead(int data){
Node *nn = new Node;
nn->data = data;
nn->next = head;
Node *tail = head;
while(tail->next!=head)
tail = tail->next;
tail->next = nn;
head = nn;
}
Node* search(int data){
Node *temp = head;
if(temp->data==data){
cout<<"Data found"<<endl;
return temp;
}
temp = temp->next;
while(temp!=head)
{
if(temp->data==data){
cout<<"Data found"<<endl;
return temp;
}
temp = temp->next;
}
return NULL;
}
void deleteNode(int data){
Node *temp = search(data);
if(temp==NULL)
cout<<"Node not found"<<endl;
else{
if(head==temp){
Node *tail = head;
while(tail->next!=head)
tail = tail->next;
tail->next = head->next;
head = head->next;
}
else
{
Node *before = head;
while(before->next != temp)
before = before->next;
before->next = temp->next;
}
delete temp;
}
}
};
int main() {
List list;
list.insert(10);
list.insert(20);
list.insert(30);
list.display();
Node *found = list.search(20);
if(found==NULL)
cout<<"Node not found"<<endl;
else
cout<<"Found node: "<<found->data<<endl;
list.insertInBetween(found, 25);
list.display();
list.insertAtHead(5);
list.display();
list.deleteNode(5);//node not found
list.display();
return 0;
}

Step by step
Solved in 3 steps with 2 images

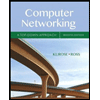
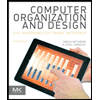
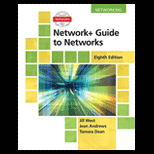
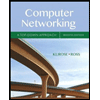
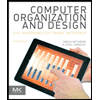
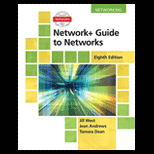
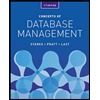
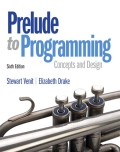
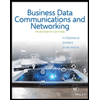