Consider objects Building, House and House Address. House should inherit from the Building class and have a or be composed of a House Address object. You will then instantiate a House and print its Building information and its House Address information.
Consider objects Building, House and House Address. House should inherit from the Building class and have a or be composed of a House Address object. You will then instantiate a House and print its Building information and its House Address information.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Introduction to Object-Oriented Programming: Inheritance and Composition**
### Problem Statement:
Consider three objects: Building, House, and House Address. The task is to implement a `House` class that inherits from the `Building` class and has or is composed of a `House Address` object. Once defined, you will instantiate a `House` and print its Building information and its House Address information.
### Steps to Solve:
1. **Define the Building Class:**
- This class could include attributes such as the building's name, type, and other relevant details.
2. **Define the House Address Class:**
- This class could include attributes such as the street name, city, zip code, and other relevant address details.
3. **Define the House Class:**
- This class will inherit from the `Building` class.
- It will include an instance (or composition) of the `House Address` object.
4. **Instantiate a House Object:**
- Create an instance of the `House` class.
- Assign values to both the inherited attributes from the `Building` class and the composed `House Address` object.
5. **Print Information:**
- Print the `Building` information.
- Print the `House Address` information.
### Example Implementation (in Python):
```python
class Building:
def __init__(self, name, building_type):
self.name = name
self.building_type = building_type
def get_building_info(self):
return f"Building Name: {self.name}, Building Type: {self.building_type}"
class HouseAddress:
def __init__(self, street, city, zip_code):
self.street = street
self.city = city
self.zip_code = zip_code
def get_address_info(self):
return f"Address: {self.street}, {self.city}, {self.zip_code}"
class House(Building):
def __init__(self, name, building_type, street, city, zip_code):
super().__init__(name, building_type)
self.address = HouseAddress(street, city, zip_code)
def get_house_info(self):
building_info = self.get_building_info()
address_info = self.address.get_address_info()
return f"{building_info}, {address_info}"
# Example Usage:
my_house = House("Sweet Home", "Residential", "123 Elm Street
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
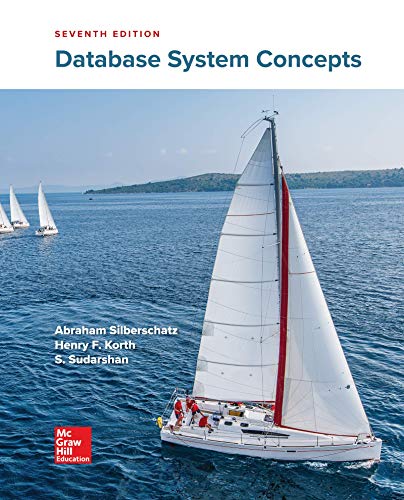
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
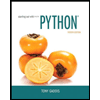
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
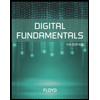
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
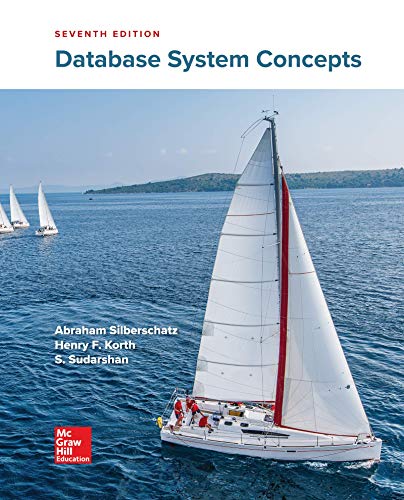
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
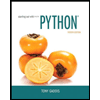
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
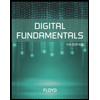
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
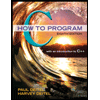
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
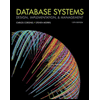
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
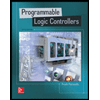
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education