Integer variables totalBudget and productCost are read from input. A product costs productCost to make, and a budget is given by totalBudget. Assign remainingBudget with the remaining budget after making as many products as possible. Ex: If the input is 13 4, then the output is: Remaining budget: 1 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 15 16 17 } int totalBudget; int product Cost; int remainingBudget; cin >> totalBudget; cin >> productCost; /* Your code goes here */ cout << "Remaining budget: " << remainingBudget << endl; return 0;
Integer variables totalBudget and productCost are read from input. A product costs productCost to make, and a budget is given by totalBudget. Assign remainingBudget with the remaining budget after making as many products as possible. Ex: If the input is 13 4, then the output is: Remaining budget: 1 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 15 16 17 } int totalBudget; int product Cost; int remainingBudget; cin >> totalBudget; cin >> productCost; /* Your code goes here */ cout << "Remaining budget: " << remainingBudget << endl; return 0;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
Code is for C++

Transcribed Image Text:### Understanding Budget Allocation in C++
In this lesson, we will learn how to allocate a given budget to produce the maximum possible number of products and determine the remaining budget. We'll use a C++ program to achieve this goal.
#### Problem Description
We have two integer variables:
1. `totalBudget` - the total amount of money available.
2. `productCost` - the cost of making one product.
The program will read these values from the input and calculate the `remainingBudget` after producing as many products as possible.
#### Example
If the input values are `13` for `totalBudget` and `4` for `productCost`, the output should be:
```
Remaining budget: 1
```
#### C++ Program
Here’s the example code to implement the given task:
```cpp
#include <iostream>
using namespace std;
int main() {
int totalBudget;
int productCost;
int remainingBudget;
cin >> totalBudget;
cin >> productCost;
/* Your code goes here */
cout << "Remaining budget: " << remainingBudget << endl;
return 0;
}
```
##### Code Explanation
1. **Include Libraries**
```cpp
#include <iostream>
using namespace std;
```
We include the iostream library for input and output operations.
2. **Main Function and Variable Declaration**
```cpp
int main() {
int totalBudget;
int productCost;
int remainingBudget;
```
We declare three integer variables: `totalBudget`, `productCost`, and `remainingBudget`.
3. **Input Handling**
```cpp
cin >> totalBudget;
cin >> productCost;
```
We take input values for `totalBudget` and `productCost`.
4. **Code Placeholder**
```cpp
/* Your code goes here */
```
This is where we will include the logic to calculate `remainingBudget`.
5. **Output the Result**
```cpp
cout << "Remaining budget: " << remainingBudget << endl;
return 0;
```
Finally, we print the `remainingBudget`.
#### Adding the Missing Code
Let’s include the logic needed to compute `remainingBudget` inside the placeholder.
To find the remaining budget:
- First, determine how many products can be made: `numberOfProducts = totalBudget / productCost`
- Then, calculate
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
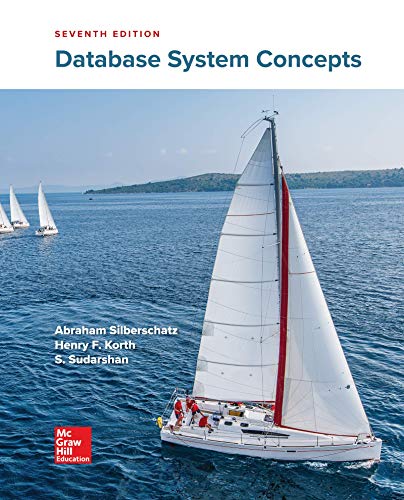
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
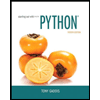
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
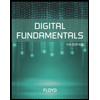
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
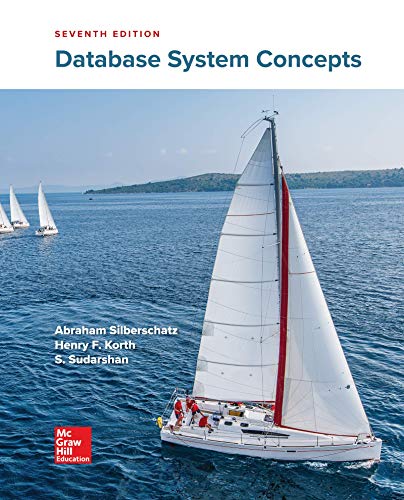
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
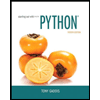
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
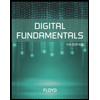
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
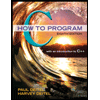
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
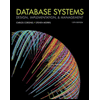
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
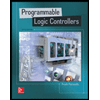
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education