Conceptual Question: How do you find the Big Oh, Big Omega, and Big Theta of an Algorithm? What do each of them mean? Can you provide an example for solving for each with some algorithm? Like... (Java) for (i = 1; i < n; i++) { j = i; while ((j > 0) && (s[j] < s[j-1])) { temp = s[j]; s[j] = s[j-1]; s[j-1] = temp; } j--; } And what about if Algorithm is recurssive like... public int runBinarySearchRecursively(int[] sortedArray, int key, int low, int high) { int middle = (low + high) / 2; if (high < low) { return -1; } if (key == sortedArray[middle]) { return middle; } else if (key < sortedArray[middle]) { return runBinarySearchRecursively(sortedArray, key, low, middle - 1); } else { return runBinarySearchRecursively(sortedArray, key, middle + 1, high); } }
Conceptual Question:
How do you find the Big Oh, Big Omega, and Big Theta of an
What do each of them mean?
Can you provide an example for solving for each with some algorithm?
Like... (Java)
for (i = 1; i < n; i++)
{
j = i;
while ((j > 0) && (s[j] < s[j-1]))
{
temp = s[j];
s[j] = s[j-1];
s[j-1] = temp;
}
j--;
}
And what about if Algorithm is recurssive like...
public int runBinarySearchRecursively(int[] sortedArray, int key, int low, int high)
{
int middle = (low + high) / 2;
if (high < low) {
return -1;
}
if (key == sortedArray[middle]) {
return middle;
} else if (key < sortedArray[middle]) {
return runBinarySearchRecursively(sortedArray, key, low, middle - 1);
} else {
return runBinarySearchRecursively(sortedArray, key, middle + 1, high);
}
}

When examining algorithms, one of the most intriguing aspects is how their performance scales with input size. Big O, Big Omega, and Big Theta are notations used to represent the upper, lower, and tight constraints on an algorithm's temporal complexity growth rate.
The principles of Big O, Big Omega, and Big Theta are discussed below, along with an analysis of the offered algorithms.
Step by step
Solved in 6 steps

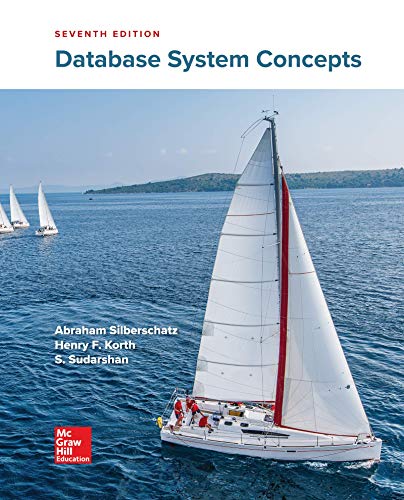
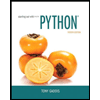
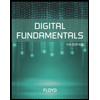
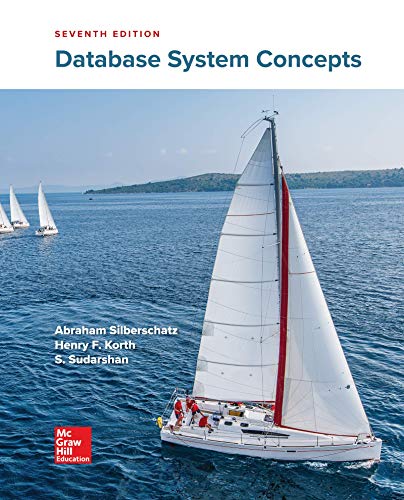
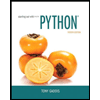
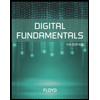
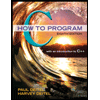
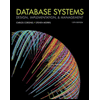
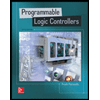