# Program 4: Internet Usage Status ## Program Description: Write a Visual C++ program to read Internet usage data from a file and create a report to summarize the data. This program gives you practice with using text files for input and output. You will also use loop algorithms for finding a total, average, and highest value. When you are ready to type in your program, create a new C++ project in Visual Studio using your last name, first initial, and pgm4 as the project name and C++ file name (e.g., `IrvingK_pgm4` and `IrvingK_pgm4.cpp`). Name the report file your program creates `pgm4rpt.txt`. ## Input: Data about each area of the world is stored in a file called `pgm4.txt`. Your program should read in an area name and three numbers representing the population, number of Internet users in 2000, and number of current Internet users for that area. After processing the data for one area, your program should read in the data for the next area, and so on. Copy the file `pgm4.txt` from Canvas into your project folder. The data in the file includes four lines for each area with the area name followed by the three numbers. Assume that you do not know the number of areas ahead of time, so your program should be able to handle any amount of data that may be in the file. Note: Pay attention to the range of integer numbers, you may have to use a long int to hold on some very large numbers contained in this file. The first couple of areas look like this: ``` Africa 1012795909 45943600 138146670 Asia 3843672925 ``` ## Program Processing Steps: 1. **Initialization** - Your program should first open the input file (to read the data from) and an output file (to write the report to) and check the success of each of these operations. Also, do any initialization of variables required and print the headings for your report to the output file. 2. **Use a while loop to:** - **Read in the name, population, and Internet usage values for an area.** - **Print the penetration and growth percentages for the area using the formulas below.** \[ \text{Penetration \%} = \frac{\text{Current Internet Users}}{\text CS2010 - Program 4: Internet Usage Status **Program Description**: Write a Visual C++ program to read in Internet usage data from a file and create a report to summarize the data. This program gives you practice with using text files for input and output. You will also use loop algorithms for finding a total, average, and highest value. When you are ready to type in your program, create a new C++ project in Visual Studio using your last name, first initial, and p4 as the project name and C++ file name (e.g., IrvingK_p4 and IrvingK_p4.cpp). Name the report file your program creates *pgm4rpt.txt*. Input: Data about each area of the world is stored in a file called *pgm4.txt*. Your program should read an area name and three numbers representing the population, number of Internet users in 2000, and number of current Internet users for that area. After processing the data for one area, your program should read in the data for the next area and so on. Copy the file *pgm4.txt* from Canvas into your project folder. The data in the file includes four lines for each area with the area name followed by the three numbers. Assume you do not know the number of areas ahead of time so your program should be able to handle any amount of data that may be in the file. Note: Pay attention to the range of integer numbers. You may have to use long long to hold on some very large numbers contained in this file. The first couple of areas look like this: ``` Africa 1019477950 45193371 297885898 Asia 3130300145 114304000 3887492825 ``` **Program Processing Steps**: a. Your program should first open the input file (to read the data from) and an output file (to write the report) and check the success of each of these operations. Also, do any initialization of variables required and print the headings for your report to the output file. b. **Use a while loop to**: - Read in the name, population, and Internet usage values for an area. - Compute the penetration and growth percentages for the area using the formulas below: \[ \text{Penetration \%} = \left(\frac{\text{Current Internet Users}}{\text{Population}}\right
# Program 4: Internet Usage Status ## Program Description: Write a Visual C++ program to read Internet usage data from a file and create a report to summarize the data. This program gives you practice with using text files for input and output. You will also use loop algorithms for finding a total, average, and highest value. When you are ready to type in your program, create a new C++ project in Visual Studio using your last name, first initial, and pgm4 as the project name and C++ file name (e.g., `IrvingK_pgm4` and `IrvingK_pgm4.cpp`). Name the report file your program creates `pgm4rpt.txt`. ## Input: Data about each area of the world is stored in a file called `pgm4.txt`. Your program should read in an area name and three numbers representing the population, number of Internet users in 2000, and number of current Internet users for that area. After processing the data for one area, your program should read in the data for the next area, and so on. Copy the file `pgm4.txt` from Canvas into your project folder. The data in the file includes four lines for each area with the area name followed by the three numbers. Assume that you do not know the number of areas ahead of time, so your program should be able to handle any amount of data that may be in the file. Note: Pay attention to the range of integer numbers, you may have to use a long int to hold on some very large numbers contained in this file. The first couple of areas look like this: ``` Africa 1012795909 45943600 138146670 Asia 3843672925 ``` ## Program Processing Steps: 1. **Initialization** - Your program should first open the input file (to read the data from) and an output file (to write the report to) and check the success of each of these operations. Also, do any initialization of variables required and print the headings for your report to the output file. 2. **Use a while loop to:** - **Read in the name, population, and Internet usage values for an area.** - **Print the penetration and growth percentages for the area using the formulas below.** \[ \text{Penetration \%} = \frac{\text{Current Internet Users}}{\text CS2010 - Program 4: Internet Usage Status **Program Description**: Write a Visual C++ program to read in Internet usage data from a file and create a report to summarize the data. This program gives you practice with using text files for input and output. You will also use loop algorithms for finding a total, average, and highest value. When you are ready to type in your program, create a new C++ project in Visual Studio using your last name, first initial, and p4 as the project name and C++ file name (e.g., IrvingK_p4 and IrvingK_p4.cpp). Name the report file your program creates *pgm4rpt.txt*. Input: Data about each area of the world is stored in a file called *pgm4.txt*. Your program should read an area name and three numbers representing the population, number of Internet users in 2000, and number of current Internet users for that area. After processing the data for one area, your program should read in the data for the next area and so on. Copy the file *pgm4.txt* from Canvas into your project folder. The data in the file includes four lines for each area with the area name followed by the three numbers. Assume you do not know the number of areas ahead of time so your program should be able to handle any amount of data that may be in the file. Note: Pay attention to the range of integer numbers. You may have to use long long to hold on some very large numbers contained in this file. The first couple of areas look like this: ``` Africa 1019477950 45193371 297885898 Asia 3130300145 114304000 3887492825 ``` **Program Processing Steps**: a. Your program should first open the input file (to read the data from) and an output file (to write the report) and check the success of each of these operations. Also, do any initialization of variables required and print the headings for your report to the output file. b. **Use a while loop to**: - Read in the name, population, and Internet usage values for an area. - Compute the penetration and growth percentages for the area using the formulas below: \[ \text{Penetration \%} = \left(\frac{\text{Current Internet Users}}{\text{Population}}\right
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
pgm4.txt
Africa
1013779050
4514400
110931700
Asia
3834792852
114304000
825094396
Australia
34700201
7620480
21263990
Europe
813319511
105096093
475069448
Middle_East
212336924
3284800
63240946
North America
344124450
108096800
266244500
South_America
592556972
18068919
204689836

Transcribed Image Text:# Program 4: Internet Usage Status
## Program Description:
Write a Visual C++ program to read Internet usage data from a file and create a report to summarize the data. This program gives you practice with using text files for input and output. You will also use loop algorithms for finding a total, average, and highest value.
When you are ready to type in your program, create a new C++ project in Visual Studio using your last name, first initial, and pgm4 as the project name and C++ file name (e.g., `IrvingK_pgm4` and `IrvingK_pgm4.cpp`). Name the report file your program creates `pgm4rpt.txt`.
## Input:
Data about each area of the world is stored in a file called `pgm4.txt`. Your program should read in an area name and three numbers representing the population, number of Internet users in 2000, and number of current Internet users for that area. After processing the data for one area, your program should read in the data for the next area, and so on.
Copy the file `pgm4.txt` from Canvas into your project folder. The data in the file includes four lines for each area with the area name followed by the three numbers. Assume that you do not know the number of areas ahead of time, so your program should be able to handle any amount of data that may be in the file. Note: Pay attention to the range of integer numbers, you may have to use a long int to hold on some very large numbers contained in this file. The first couple of areas look like this:
```
Africa
1012795909
45943600
138146670
Asia
3843672925
```
## Program Processing Steps:
1. **Initialization**
- Your program should first open the input file (to read the data from) and an output file (to write the report to) and check the success of each of these operations. Also, do any initialization of variables required and print the headings for your report to the output file.
2. **Use a while loop to:**
- **Read in the name, population, and Internet usage values for an area.**
- **Print the penetration and growth percentages for the area using the formulas below.**
\[
\text{Penetration \%} = \frac{\text{Current Internet Users}}{\text

Transcribed Image Text:CS2010 - Program 4: Internet Usage Status
**Program Description**:
Write a Visual C++ program to read in Internet usage data from a file and create a report to summarize the data. This program gives you practice with using text files for input and output. You will also use loop algorithms for finding a total, average, and highest value.
When you are ready to type in your program, create a new C++ project in Visual Studio using your last name, first initial, and p4 as the project name and C++ file name (e.g., IrvingK_p4 and IrvingK_p4.cpp). Name the report file your program creates *pgm4rpt.txt*.
Input: Data about each area of the world is stored in a file called *pgm4.txt*. Your program should read an area name and three numbers representing the population, number of Internet users in 2000, and number of current Internet users for that area. After processing the data for one area, your program should read in the data for the next area and so on.
Copy the file *pgm4.txt* from Canvas into your project folder. The data in the file includes four lines for each area with the area name followed by the three numbers. Assume you do not know the number of areas ahead of time so your program should be able to handle any amount of data that may be in the file. Note: Pay attention to the range of integer numbers. You may have to use long long to hold on some very large numbers contained in this file. The first couple of areas look like this:
```
Africa
1019477950
45193371
297885898
Asia
3130300145
114304000
3887492825
```
**Program Processing Steps**:
a. Your program should first open the input file (to read the data from) and an output file (to write the report) and check the success of each of these operations. Also, do any initialization of variables required and print the headings for your report to the output file.
b. **Use a while loop to**:
- Read in the name, population, and Internet usage values for an area.
- Compute the penetration and growth percentages for the area using the formulas below:
\[
\text{Penetration \%} = \left(\frac{\text{Current Internet Users}}{\text{Population}}\right
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
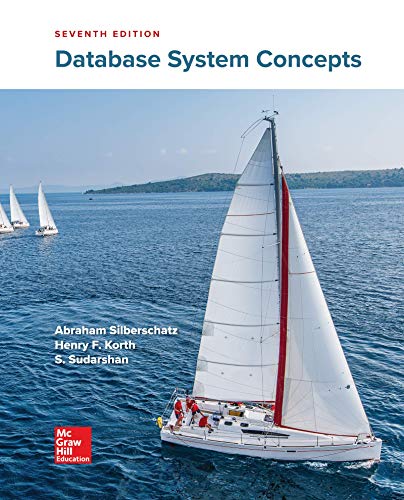
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
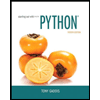
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
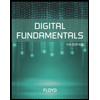
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
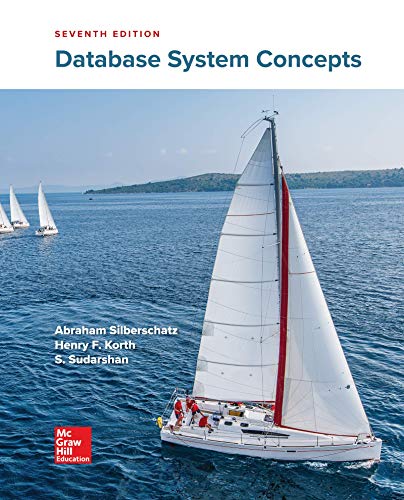
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
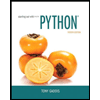
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
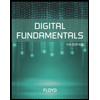
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
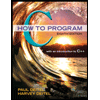
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
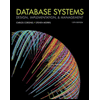
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
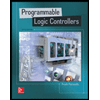
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education