Complete the recursive function remove_last which creates a new list identical to the input list s but with the last element in the sequence that is equal to x removed. Hint: Remember that you can use negative indexing on lists! For example 1st [-1] refers to the last element in a list 1st, lst [-2] refers to the second to last element... def remove_last(x, s): """Create a new list that is identical to s but with the last element from the list that is equal to x removed. >>> remove_last(1, []) [] remove_last(1, [1]) >>> remove_last(1, [1,1]) [1] >>> remove_last(1, [2,1]) [2] >>> [] >>> remove_last(1, [3,1,2]) [3, 2] >>> remove_last(1, [3,1,2,1]) [3, 1, 2] >>> remove_last (5, [3, 5, 2, 5, 11]) [3, 5, 2, 11] HIGH "*** YOUR CODE HERE ***"
Complete the recursive function remove_last which creates a new list identical to the input list s but with the last element in the sequence that is equal to x removed. Hint: Remember that you can use negative indexing on lists! For example 1st [-1] refers to the last element in a list 1st, lst [-2] refers to the second to last element... def remove_last(x, s): """Create a new list that is identical to s but with the last element from the list that is equal to x removed. >>> remove_last(1, []) [] remove_last(1, [1]) >>> remove_last(1, [1,1]) [1] >>> remove_last(1, [2,1]) [2] >>> [] >>> remove_last(1, [3,1,2]) [3, 2] >>> remove_last(1, [3,1,2,1]) [3, 1, 2] >>> remove_last (5, [3, 5, 2, 5, 11]) [3, 5, 2, 11] HIGH "*** YOUR CODE HERE ***"
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Complete the recursive function remove_last which creates a new list identical to the input list s but
with the last element in the sequence that is equal to x removed.
Hint: Remember that you can use negative indexing on lists! For example 1st [-1] refers to the last
element in a list 1st, lst [-2] refers to the second to last element...
def remove_last(x, s):
"""Create a new list that is identical to s but with the last
element from the list that is equal to x removed.
>>> remove_last(1, [])
[]
>>> remove_last(1, [1])
[]
>>>
[1]
>>>
[2]
remove_last(1, [1,1])
remove_last(1, [2,1])
>>> remove_last(1, [3,1,2])
[3, 2]
>>> remove_last(1, [3,1,2,1])
[3, 1, 2]
>>> remove_last (5, [3, 5, 2, 5, 11])
[3, 5, 2, 11]
IIIII
"*** YOUR CODE HERE ***"
Illustrated here is a more complete doctest that shows good testing methodology. It is a little cumbersome
as documentation, but you'll want to think about it for your projects. Test every condition that might come
up. Then you won't be surprised when it does.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc32a25d1-aced-4632-8199-60cb873673c0%2Fe05b2827-7cec-4659-a4eb-8e970c46900b%2Fvbw3k7_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Complete the recursive function remove_last which creates a new list identical to the input list s but
with the last element in the sequence that is equal to x removed.
Hint: Remember that you can use negative indexing on lists! For example 1st [-1] refers to the last
element in a list 1st, lst [-2] refers to the second to last element...
def remove_last(x, s):
"""Create a new list that is identical to s but with the last
element from the list that is equal to x removed.
>>> remove_last(1, [])
[]
>>> remove_last(1, [1])
[]
>>>
[1]
>>>
[2]
remove_last(1, [1,1])
remove_last(1, [2,1])
>>> remove_last(1, [3,1,2])
[3, 2]
>>> remove_last(1, [3,1,2,1])
[3, 1, 2]
>>> remove_last (5, [3, 5, 2, 5, 11])
[3, 5, 2, 11]
IIIII
"*** YOUR CODE HERE ***"
Illustrated here is a more complete doctest that shows good testing methodology. It is a little cumbersome
as documentation, but you'll want to think about it for your projects. Test every condition that might come
up. Then you won't be surprised when it does.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
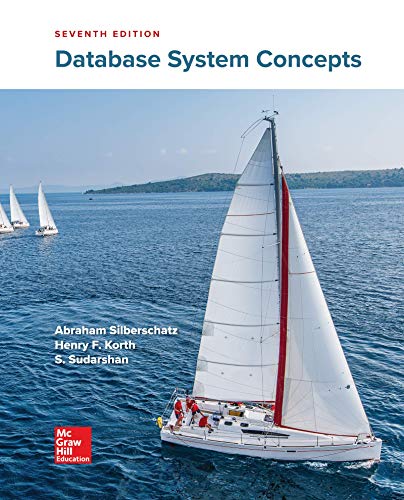
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
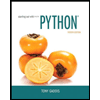
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
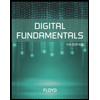
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
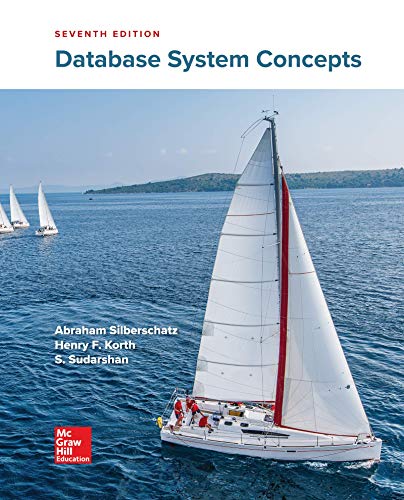
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
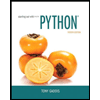
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
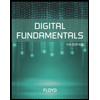
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
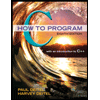
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
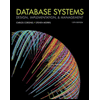
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
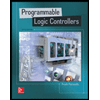
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education