Complete an Array-Based implementation of the ADT List including the following main class:
Complete an Array-Based implementation of the ADT List including the following main class:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Complete an Array-Based implementation of the ADT List including the following main class:
![// *********
// Interface ListInterface for the ADT list.
// ***************************
public interface ListInterface {
public boolean isEmpty();
public int size();
public void add (int index, Object item)
}
***
throws ListIndexOutOfBoundsException,
public Object get (int index)
public void remove(int index)
// ********
ListException;
public void removeAll();
// end ListInterface
throws ListIndexOutOfBounds Exception;
throws ListIndexOutOfBoundsException;
The following class implements the interface List Interface, using arrays.
// Array-based implementation of the ADT list.
// *****************************
} // end size
public class ListArrayBased implements List Interface {
private static final int MAX_LIST = 50;
private Object items []; // an array of list items
private int numItems; // number of items in list
public ListArrayBased () {
items = new Object [MAX_LIST];
numItems = 0;
} // end default constructor
public boolean isEmpty() {
return (numItems == 0);
} // end isEmpty
public int size() {
return numItems;
public void removeAll() {
// Creates a new array; marks old array for
// garbage collection.
items = new Object [MAX_LIST];
numItems = 0;
} // end removeAll
public void add(int index, Object item)
throws ListIndexOutOfBounds Exception {
if (numItems > MAX_LIST) {
throw new ListException ("ListException on add");
} // end if](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5ccb13ef-bf62-4bab-95f5-f0fc9e7adadc%2F000dd6c6-23ee-4999-b05f-01b965f52498%2Flgvpi9q_processed.png&w=3840&q=75)
Transcribed Image Text:// *********
// Interface ListInterface for the ADT list.
// ***************************
public interface ListInterface {
public boolean isEmpty();
public int size();
public void add (int index, Object item)
}
***
throws ListIndexOutOfBoundsException,
public Object get (int index)
public void remove(int index)
// ********
ListException;
public void removeAll();
// end ListInterface
throws ListIndexOutOfBounds Exception;
throws ListIndexOutOfBoundsException;
The following class implements the interface List Interface, using arrays.
// Array-based implementation of the ADT list.
// *****************************
} // end size
public class ListArrayBased implements List Interface {
private static final int MAX_LIST = 50;
private Object items []; // an array of list items
private int numItems; // number of items in list
public ListArrayBased () {
items = new Object [MAX_LIST];
numItems = 0;
} // end default constructor
public boolean isEmpty() {
return (numItems == 0);
} // end isEmpty
public int size() {
return numItems;
public void removeAll() {
// Creates a new array; marks old array for
// garbage collection.
items = new Object [MAX_LIST];
numItems = 0;
} // end removeAll
public void add(int index, Object item)
throws ListIndexOutOfBounds Exception {
if (numItems > MAX_LIST) {
throw new ListException ("ListException on add");
} // end if
![if (index >= 0 && index <= numItems) {
// make room for new element by shifting all items at
// positions >= index toward the end of the
// list (no shift if index == numItems+1)
for (int pos = numItems; pos >= index; pos--) {
items [pos+1] = items [pos];
}
} // end for
// insert new item
items [index] = item;
numItems++;
}
else { // index out of range
throw new ListIndexOutOfBoundsException (
"ListIndexOutOfBounds Exception on add");
// end if
}
} //end add
public Object get (int index)
if (index >= 0 && index < numItems) {
return items [index];
}
else { // index out of range
throw new ListIndexOutOfBoundsException (
"ListIndexOutOfBoundsException on get");
} // end if
} // end get
throws ListIndexOutOfBounds Exception {
public void remove(int index)
if (index >= 0 && index < numItems) {
// delete item by shifting all items at
// positions> index toward the beginning of the list
// (no shift if index == size)
numItems--;
for (int pos= index+1; pos <= size(); pos++) {
items [pos-1] = items [pos];
// end for
} // end if
} // end remove
throws ListIndexOutOfBounds Exception {
}
else { // index out of range
throw new ListIndexOutOfBoundsException(
"ListIndexOutOfBounds Exception on remove");
// end ListArrayBased
The following program segment demonstrates the use of ListArrayBased:
static public void main(String args[]) {
ListArrayBased aList = new ListArrayBased ();
String dataItem;
aList.add(0, "Cathryn");
dataItem = (String) aList.get(0);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5ccb13ef-bf62-4bab-95f5-f0fc9e7adadc%2F000dd6c6-23ee-4999-b05f-01b965f52498%2Frmsp3on_processed.png&w=3840&q=75)
Transcribed Image Text:if (index >= 0 && index <= numItems) {
// make room for new element by shifting all items at
// positions >= index toward the end of the
// list (no shift if index == numItems+1)
for (int pos = numItems; pos >= index; pos--) {
items [pos+1] = items [pos];
}
} // end for
// insert new item
items [index] = item;
numItems++;
}
else { // index out of range
throw new ListIndexOutOfBoundsException (
"ListIndexOutOfBounds Exception on add");
// end if
}
} //end add
public Object get (int index)
if (index >= 0 && index < numItems) {
return items [index];
}
else { // index out of range
throw new ListIndexOutOfBoundsException (
"ListIndexOutOfBoundsException on get");
} // end if
} // end get
throws ListIndexOutOfBounds Exception {
public void remove(int index)
if (index >= 0 && index < numItems) {
// delete item by shifting all items at
// positions> index toward the beginning of the list
// (no shift if index == size)
numItems--;
for (int pos= index+1; pos <= size(); pos++) {
items [pos-1] = items [pos];
// end for
} // end if
} // end remove
throws ListIndexOutOfBounds Exception {
}
else { // index out of range
throw new ListIndexOutOfBoundsException(
"ListIndexOutOfBounds Exception on remove");
// end ListArrayBased
The following program segment demonstrates the use of ListArrayBased:
static public void main(String args[]) {
ListArrayBased aList = new ListArrayBased ();
String dataItem;
aList.add(0, "Cathryn");
dataItem = (String) aList.get(0);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
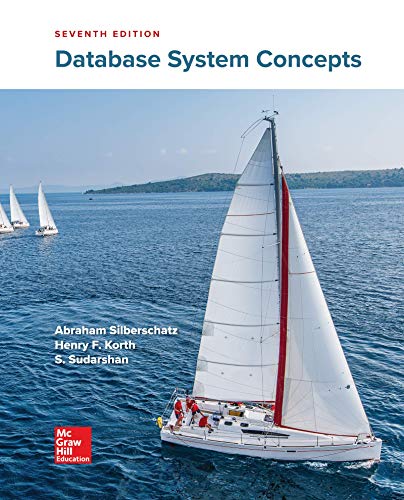
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
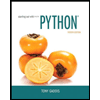
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
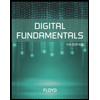
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
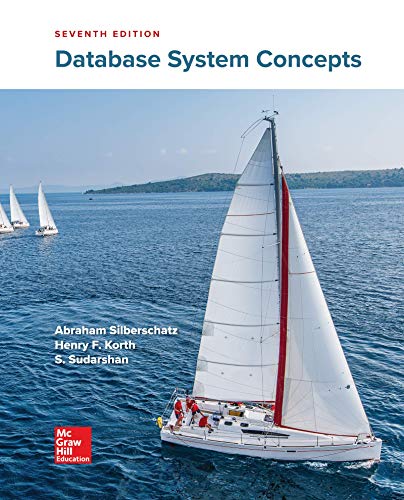
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
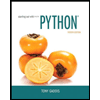
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
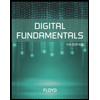
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
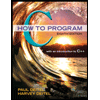
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
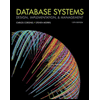
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
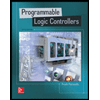
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education