Code in Python mystr is "numberlike" if: - mystr is not the empty string - the first character in mystr is a digit - the count of '.' in mystr is zero or one (can't have more than one decimal point in a number) - every character in mystr is a digit ('0', '1', '2', ..., '9') or a decimal point ('.') Write the code to define the function is_numberlike(mystr), which takes a string parameter and returns a boolean result. If mystr is numberlike, the function returns True; otherwise, the function returns False. Hint: Check the first three requirements with decision statements, then use a for loop to iterate over mystr and check the every character is a digit or a dot For example: Test Result if not (is_numberlike("13.5") is True): print("error") if not (is_numberlike("-5532") is False): print("error") if not (is_numberlike("175") is True): print("error")
Code in Python mystr is "numberlike" if: - mystr is not the empty string - the first character in mystr is a digit - the count of '.' in mystr is zero or one (can't have more than one decimal point in a number) - every character in mystr is a digit ('0', '1', '2', ..., '9') or a decimal point ('.') Write the code to define the function is_numberlike(mystr), which takes a string parameter and returns a boolean result. If mystr is numberlike, the function returns True; otherwise, the function returns False. Hint: Check the first three requirements with decision statements, then use a for loop to iterate over mystr and check the every character is a digit or a dot For example: Test Result if not (is_numberlike("13.5") is True): print("error") if not (is_numberlike("-5532") is False): print("error") if not (is_numberlike("175") is True): print("error")
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Code in Python
mystr is "numberlike" if:
- mystr is not the empty string
- the first character in mystr is a digit
- the count of '.' in mystr is zero or one (can't have more than one decimal point in a number)
- every character in mystr is a digit ('0', '1', '2', ..., '9') or a decimal point ('.')
Write the code to define the function is_numberlike(mystr), which takes a string parameter and returns a boolean result.
If mystr is numberlike, the function returns True; otherwise, the function returns False.
Hint: Check the first three requirements with decision statements, then use a for loop to iterate over mystr and check the every character is a digit or a dot
For example:
Test | Result |
---|---|
if not (is_numberlike("13.5") is True): print("error") | |
if not (is_numberlike("-5532") is False): print("error") | |
if not (is_numberlike("175") is True): print("error") |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
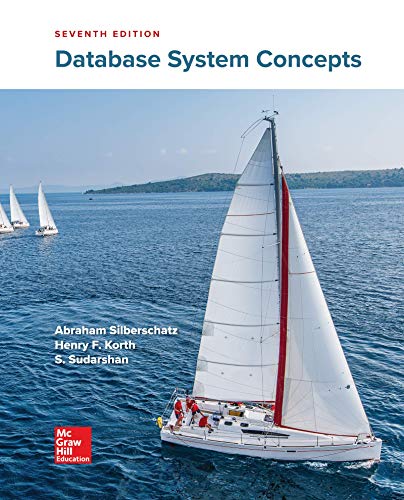
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
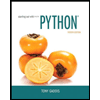
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
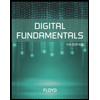
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
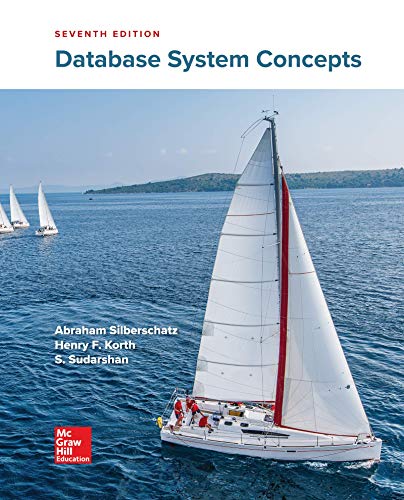
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
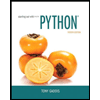
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
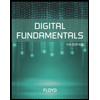
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
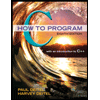
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
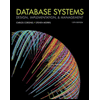
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
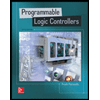
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education