choiceA - SLNode temp = myFront.getSuccessor(); myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(temp); myFront.setSuccessor(temp.getSuccessor()); temp.setSuccessor(null); temp = null; choiceB - SLNode temp = myFront.getSuccessor(); myFront.getSuccessor().getSuccessor().setSuccessor(temp); myFront.setSuccessor(temp.getSuccessor()); temp.setSuccessor(null); temp = null; choiceC- SLNode temp = myFront.getSuccessor(); myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(temp); myFront.getSuccessor().setSuccessor(temp.getSuccessor()); temp.setSuccessor(null); temp = null; choiceD- SLNode temp = myFront; myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(temp); myFront.setSuccessor(temp.getSuccessor()); temp.setSuccessor(null); temp = null;
choiceA - SLNode<Integer> temp = myFront.getSuccessor();
myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(temp);
myFront.setSuccessor(temp.getSuccessor());
temp.setSuccessor(null);
temp = null;
choiceB - SLNode<Integer> temp = myFront.getSuccessor();
myFront.getSuccessor().getSuccessor().setSuccessor(temp);
myFront.setSuccessor(temp.getSuccessor());
temp.setSuccessor(null);
temp = null;
choiceC- SLNode<Integer> temp = myFront.getSuccessor();
myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(temp);
myFront.getSuccessor().setSuccessor(temp.getSuccessor());
temp.setSuccessor(null);
temp = null;
choiceD- SLNode<Integer> temp = myFront;
myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(temp);
myFront.setSuccessor(temp.getSuccessor());
temp.setSuccessor(null);
temp = null;
![Which code shown below changes the circularly linked list of nodes (with a
header/sentinel node) below:
myFront
3
Into this singularly lined list (with a header/sentinel node):
myFront →
>¬2>3]>1> null
In both cases, myFront is a variable of type SLNode referring to a header/sentinel node
at the front of the chain of nodes.
Here is the code which creates the initial condition:
= new SLNode<Integer>(3, null);
SLNode<Integer> myFront
myFront
myFront
myFront
myFront.getSuccessor().getSuccessor().getSuccessor().setSuccessor(myFront);
= new SLNode<Integer>(2, myFront);
new SLNode<Integer>(1, myFront);
= new SLNode<Integer>(null, myFront);
(The definition of the SLNode class appears below.)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8a000fe0-342d-4f80-8d89-1b56a9b64020%2F8507f655-8bba-4bcf-9d20-e6c6e75d2e09%2Fvpndvpf_processed.jpeg&w=3840&q=75)


Step by step
Solved in 2 steps

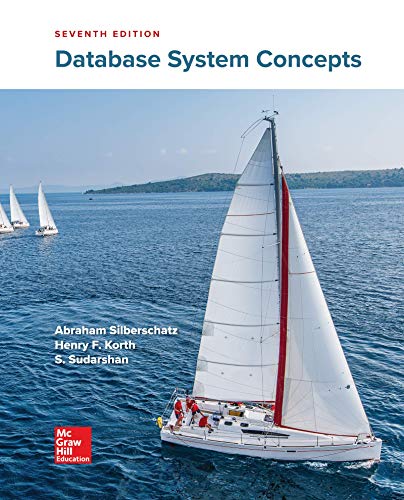
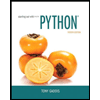
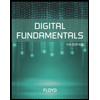
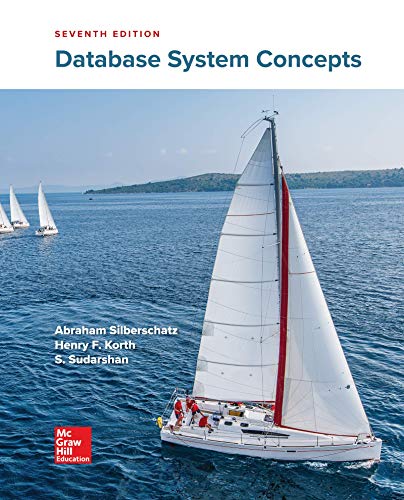
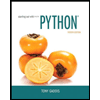
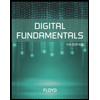
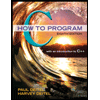
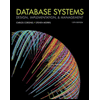
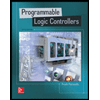