Change the node server code by adding some instructions: const express = require('express'); const dotenv = require('dotenv'); dotenv.config(); const app = express(); app.get('/dotted', (req, res) => { const { word1, word2 } = req.query; const dots = '.'.repeat(30 - word1.length - word2.length); const result = `${word1}${dots}${word2}`; res.status(200).contentType('text/html').send(result); }); app.get("/fizzBuzz", (req, res) => { const start = parseInt(req.query.start); const end = parseInt(req.query.end); let response = ""; for (let i = start; i <= end; i++) { if (i % 3 === 0 && i % 5 === 0) { response += "FizzBuzz\n"; } else if (i % 3 === 0) { response += "Fizz\n"; } else if (i % 5 === 0) { response += "Buzz\n"; } else { response += `${i}\n`; } } res.contentType("text/html"); res.send(`${response}`); }); app.get('/gradeStats', (req, res) => { const { grades } = req.query; const min = Math.min(...grades); const max = Math.max(...grades); const avg = (grades.reduce((a, b) => a + b) / grades.length).toFixed(2); const result = JSON.stringify({ average: avg, minimum: min, maximum: max }); res.status(200).contentType('application/json').send(result); }); app.get("/rectangle", (req, res) => { const length = parseInt(req.query.length); const width = parseInt(req.query.width); const area = length * width; const perimeter = 2 * (length + width); const response = { area, perimeter }; res.contentType("application/json"); res.send(response); }); const PORT = process.env.PORT || 3000; const HOST = process.env.HOST || 'localhost'; app.listen(PORT, HOST, () => { console.log(`Server running at http://${HOST}:${PORT}`); }); Use Express Session Use the session to keep track of: How many commands have been executed. What the last command executed was. Create a middleware function to keep track of this information. Add a JSON endpoint: /stats It will return responses like: {"commandCount": 3, "lastCommand": "/dotted"} Calling /stats will not increment commandCount or change the lastCommand value.
Change the node server code by adding some instructions: const express = require('express'); const dotenv = require('dotenv'); dotenv.config(); const app = express(); app.get('/dotted', (req, res) => { const { word1, word2 } = req.query; const dots = '.'.repeat(30 - word1.length - word2.length); const result = `${word1}${dots}${word2}`; res.status(200).contentType('text/html').send(result); }); app.get("/fizzBuzz", (req, res) => { const start = parseInt(req.query.start); const end = parseInt(req.query.end); let response = ""; for (let i = start; i <= end; i++) { if (i % 3 === 0 && i % 5 === 0) { response += "FizzBuzz\n"; } else if (i % 3 === 0) { response += "Fizz\n"; } else if (i % 5 === 0) { response += "Buzz\n"; } else { response += `${i}\n`; } } res.contentType("text/html"); res.send(`${response}`); }); app.get('/gradeStats', (req, res) => { const { grades } = req.query; const min = Math.min(...grades); const max = Math.max(...grades); const avg = (grades.reduce((a, b) => a + b) / grades.length).toFixed(2); const result = JSON.stringify({ average: avg, minimum: min, maximum: max }); res.status(200).contentType('application/json').send(result); }); app.get("/rectangle", (req, res) => { const length = parseInt(req.query.length); const width = parseInt(req.query.width); const area = length * width; const perimeter = 2 * (length + width); const response = { area, perimeter }; res.contentType("application/json"); res.send(response); }); const PORT = process.env.PORT || 3000; const HOST = process.env.HOST || 'localhost'; app.listen(PORT, HOST, () => { console.log(`Server running at http://${HOST}:${PORT}`); }); Use Express Session Use the session to keep track of: How many commands have been executed. What the last command executed was. Create a middleware function to keep track of this information. Add a JSON endpoint: /stats It will return responses like: {"commandCount": 3, "lastCommand": "/dotted"} Calling /stats will not increment commandCount or change the lastCommand value.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Change the node server code by adding some instructions:
const express = require('express');
const dotenv = require('dotenv');
dotenv.config();
const app = express();
app.get('/dotted', (req, res) => {
const { word1, word2 } = req.query;
const dots = '.'.repeat(30 - word1.length - word2.length);
const result = `<pre>${word1}${dots}${word2}</pre>`;
res.status(200).contentType('text/html').send(result);
});
app.get("/fizzBuzz", (req, res) => {
const start = parseInt(req.query.start);
const end = parseInt(req.query.end);
let response = "";
for (let i = start; i <= end; i++) {
if (i % 3 === 0 && i % 5 === 0) {
response += "FizzBuzz\n";
} else if (i % 3 === 0) {
response += "Fizz\n";
} else if (i % 5 === 0) {
response += "Buzz\n";
} else {
response += `${i}\n`;
}
}
res.contentType("text/html");
res.send(`<pre>${response}</pre>`);
});
app.get('/gradeStats', (req, res) => {
const { grades } = req.query;
const min = Math.min(...grades);
const max = Math.max(...grades);
const avg = (grades.reduce((a, b) => a + b) / grades.length).toFixed(2);
const result = JSON.stringify({ average: avg, minimum: min, maximum: max });
res.status(200).contentType('application/json').send(result);
});
app.get("/rectangle", (req, res) => {
const length = parseInt(req.query.length);
const width = parseInt(req.query.width);
const area = length * width;
const perimeter = 2 * (length + width);
const response = { area, perimeter };
res.contentType("application/json");
res.send(response);
});
const PORT = process.env.PORT || 3000;
const HOST = process.env.HOST || 'localhost';
app.listen(PORT, HOST, () => {
console.log(`Server running at http://${HOST}:${PORT}`);
});
Use Express Session
- Use the session to keep track of:
- How many commands have been executed.
- What the last command executed was.
- Create a middleware function to keep track of this information.
- Add a JSON endpoint: /stats
- It will return responses like: {"commandCount": 3, "lastCommand": "/dotted"}
- Calling /stats will not increment commandCount or change the lastCommand value.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
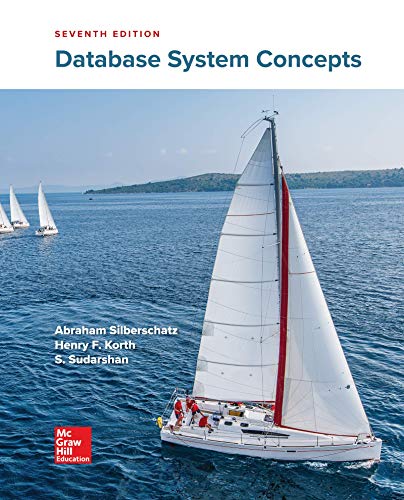
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
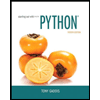
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
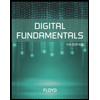
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
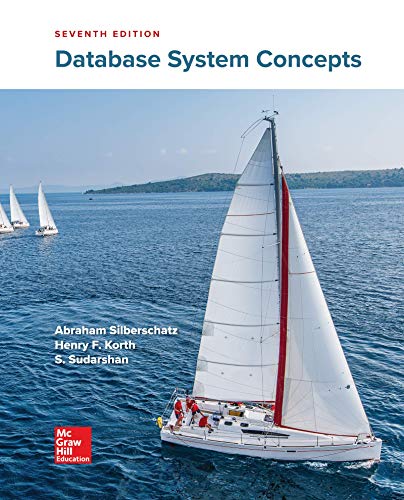
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
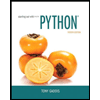
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
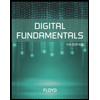
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
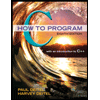
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
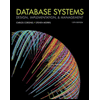
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
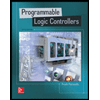
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education